How to Set Window to Fullscreen in Pygame
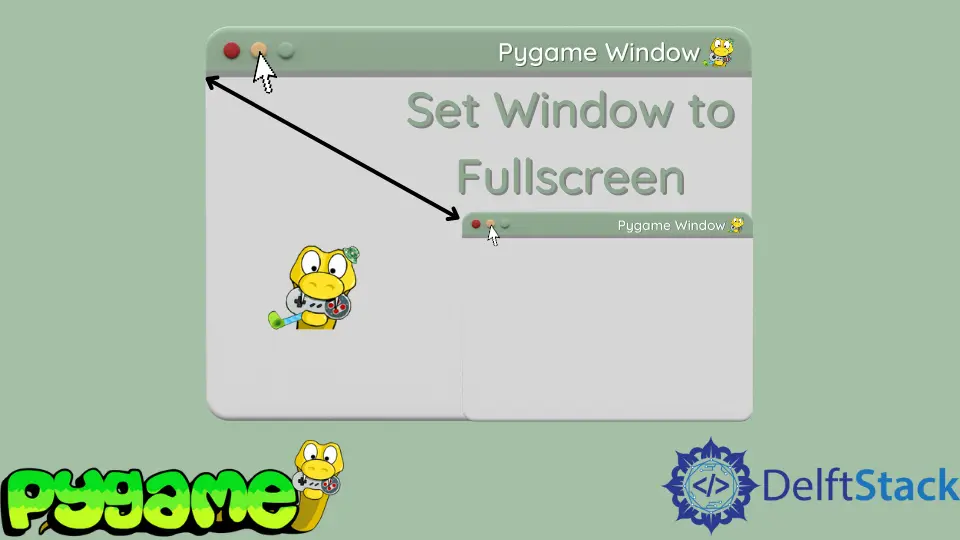
This tutorial will show you the most barebone start for any game in Pygame. Further, you’ll also learn how to set the Window to fullscreen.
Create a Simple Game Framework
The following code shows a simple framework of a game. The example will open a window and keep it running until it is closed.
- In the first 3 lines, we’ll import the
pygame
andsys
modules, and the second library will then close the program. - We continue with initiating Pygame with
pygame.init()
. - Then, we set the fps to 60; we use this in the
update
method of thepygame.time.Clock
object we create in the following line. - Furthermore, we set the screen width and height.
- We pass this info into the
pygame.display.set_mode()
method.
Now, we go over to the game’s main loop, where most of the logic happens. It is a loop because games redraw what is going on many times a second, in our example, 60 times.
We can make an infinite loop by breaking it with the pygame.quit()
and the sys.exit()
functions. We first fill the whole screen with black color; then, we loop through all events.
If one of the events is the pygame.QUIT
event, we quit the program with the above functions. This event is triggered when clicking the red x
in the Window’s top right corner.
After this, there’s room for all the code that makes up the actual game, and last, but not least, we update the screen with pygame.display.flip()
. We wait for the right time to pass, so the game runs at 60 frames per second.
Code:
# Imports
import sys
import pygame
# Configuration
pygame.init()
fps = 60
fpsClock = pygame.time.Clock()
width, height = 640, 480
screen = pygame.display.set_mode((width, height))
# Game loop.
while True:
screen.fill((0, 0, 0))
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
# Main Loop Code belongs here
pygame.display.flip()
fpsClock.tick(fps)
Set Window to Fullscreen in Pygame
If we run the example above, we will see a little window, sized by the width
and height
variables. If we want a game to be fullscreen, we pass the pygame.FULLSCREEN
flag, and there you have it, a fullscreen Pygame window.
Code:
screen = pygame.display.set_mode((width, height), pygame.FULLSCREEN)
Hi, my name is Maxim Maeder, I am a young programming enthusiast looking to have fun coding and teaching you some things about programming.
GitHub