How to Reset Pandas DataFrame Index
-
Pandas DataFrame
reset_index()
Method -
Reset Index of a DataFrame Using
pandas.DataFrame.reset_index()
Method
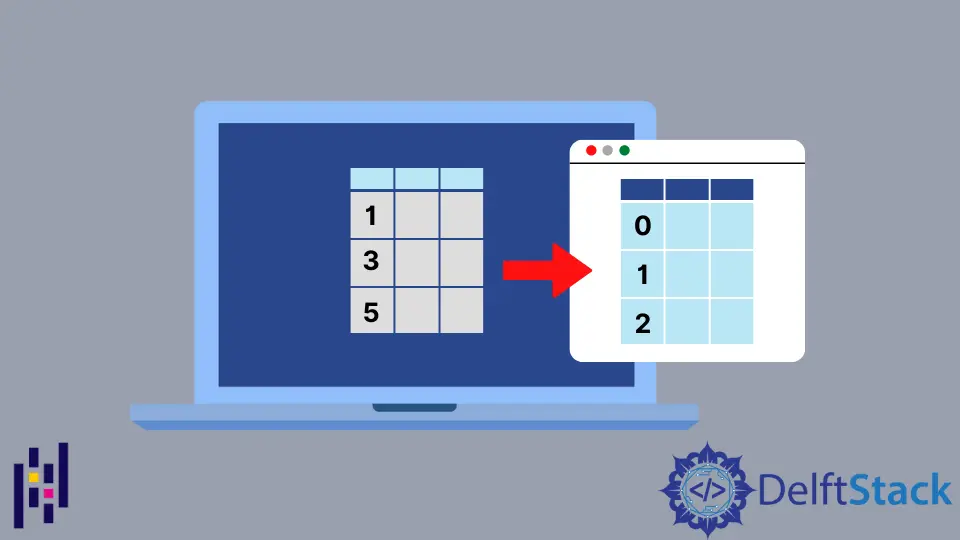
This tutorial explains how we can reset the index in Pandas DataFrame using pandas.DataFrame.reset_index()
. The reset_index()
method sets the index of the DataFrame to default index with numbers ranging from 0
to (number of rows in DataFrame-1)
.
Pandas DataFrame reset_index()
Method
Syntax
DataFrame.reset_index(level=None, drop=False, inplace=False, col_level=0, col_fill="")
Reset Index of a DataFrame Using pandas.DataFrame.reset_index()
Method
import pandas as pd
roll_no = [501, 502, 503, 504, 505]
student_df = pd.DataFrame(
{
"Name": ["Alice", "Steven", "Neesham", "Chris", "Alice"],
"Age": [17, 20, 18, 21, 15],
"City": ["New York", "Portland", "Boston", "Seattle", "Austin"],
"Grade": ["A", "B-", "B+", "A-", "A"],
},
index=roll_no,
)
print(student_df)
Output:
Name Age City Grade
501 Alice 17 New York A
502 Steven 20 Portland B-
503 Neesham 18 Boston B+
504 Chris 21 Seattle A-
505 Alice 15 Austin A
Suppose we have a DataFrame with five rows and four columns as displayed in the output. We also have an index set in the DataFrame.
Reset Index of a DataFrame Keeping the Initial Index of DataFrame as a Column
import pandas as pd
roll_no = [501, 502, 503, 504, 505]
student_df = pd.DataFrame(
{
"Name": ["Alice", "Steven", "Neesham", "Chris", "Alice"],
"Age": [17, 20, 18, 21, 15],
"City": ["New York", "Portland", "Boston", "Seattle", "Austin"],
"Grade": ["A", "B-", "B+", "A-", "A"],
},
index=roll_no,
)
print("Initial DataFrame:")
print(student_df)
print("")
print("DataFrame after reset_index:")
student_df.reset_index(inplace=True, drop=False)
print(student_df)
Output:
Initial DataFrame:
Name Age City Grade
501 Alice 17 New York A
502 Steven 20 Portland B-
503 Neesham 18 Boston B+
504 Chris 21 Seattle A-
505 Alice 15 Austin A
DataFrame after reset_index:
index Name Age City Grade
0 501 Alice 17 New York A
1 502 Steven 20 Portland B-
2 503 Neesham 18 Boston B+
3 504 Chris 21 Seattle A-
4 505 Alice 15 Austin A
It resets the index of the DataFrame student_df
to the default index. The inplace=True
makes the change in the original DataFrame itself. If we use drop=False
, the initial index is placed as a column in the DataFrame after using the reset_index()
method.
Reset Index of a DataFrame Removing the Initial Index of DataFrame
import pandas as pd
roll_no = [501, 502, 503, 504, 505]
student_df = pd.DataFrame(
{
"Name": ["Alice", "Steven", "Neesham", "Chris", "Alice"],
"Age": [17, 20, 18, 21, 15],
"City": ["New York", "Portland", "Boston", "Seattle", "Austin"],
"Grade": ["A", "B-", "B+", "A-", "A"],
},
index=roll_no,
)
print("Initial DataFrame:")
print(student_df)
print("")
print("DataFrame after reset_index:")
student_df.reset_index(inplace=True, drop=True)
print(student_df)
Output:
Initial DataFrame:
Name Age City Grade
501 Alice 17 New York A
502 Steven 20 Portland B-
503 Neesham 18 Boston B+
504 Chris 21 Seattle A-
505 Alice 15 Austin A
DataFrame after reset_index:
Name Age City Grade
0 Alice 17 New York A
1 Steven 20 Portland B-
2 Neesham 18 Boston B+
3 Chris 21 Seattle A-
4 Alice 15 Austin A
It resets the index of the DataFrame student_df
to the default index. As we have set drop=True
in the reset_index()
method, the initial index is dropped from the DataFrame.
Reset Index of a DataFrame After Deleting Rows
import pandas as pd
roll_no = [501, 502, 503, 504, 505]
student_df = pd.DataFrame(
{
"Name": ["Alice", "Steven", "Neesham", "Chris", "Alice"],
"Age": [17, 20, 18, 21, 15],
"City": ["New York", "Portland", "Boston", "Seattle", "Austin"],
"Grade": ["A", "B-", "B+", "A-", "A"],
}
)
student_df.drop([2, 3], inplace=True)
print("Initial DataFrame:")
print(student_df)
print("")
student_df.reset_index(inplace=True, drop=True)
print("DataFrame after reset_index:")
print(student_df)
Output:
Initial DataFrame:
Name Age City Grade
0 Alice 17 New York A
1 Steven 20 Portland B-
4 Alice 15 Austin A
DataFrame after reset_index:
Name Age City Grade
0 Alice 17 New York A
1 Steven 20 Portland B-
2 Alice 15 Austin A
As we can see in the output, we have missing indices after deleting rows. In such cases, we can use the reset_index()
method to use the index without missing values.
If we want the initial index to be placed as the column of the DataFrame, we can use drop=False
in the reset_index()
method.
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn