How to Pandas Create Column Based on Other Columns
- Create New Columns in Pandas DataFrame Based on the Values of Other Columns Using the Element-Wise Operation
-
Create New Columns in Pandas DataFrame Based on the Values of Other Columns Using the
DataFrame.apply()
Method
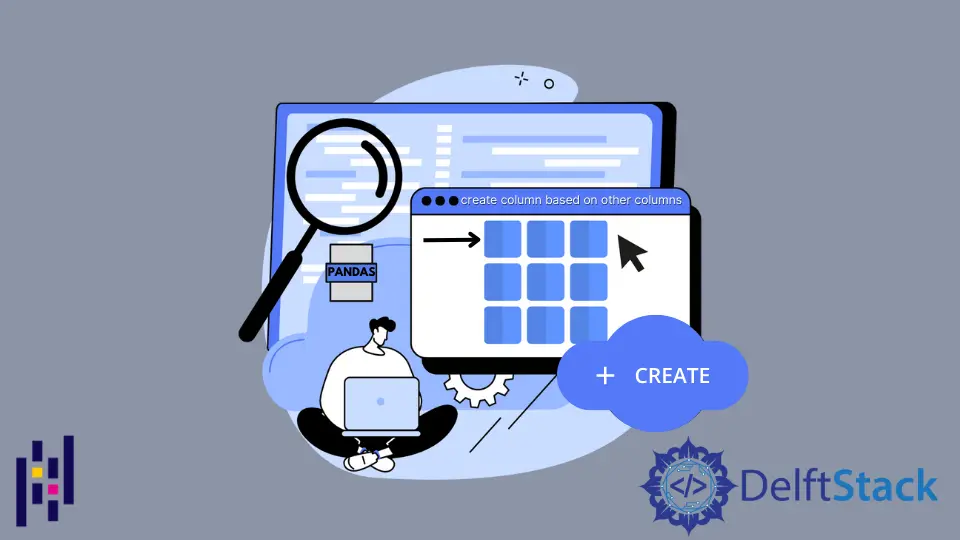
This tutorial will introduce how we can create new columns in Pandas DataFrame based on the values of other columns in the DataFrame by applying a function to each element of a column or using the DataFrame.apply()
method.
import pandas as pd
items_df = pd.DataFrame(
{
"Id": [302, 504, 708, 103, 343, 565],
"Name": ["Watch", "Camera", "Phone", "Shoes", "Laptop", "Bed"],
"Cost": [300, 400, 350, 100, 1000, 400],
"Discount(%)": [10, 15, 5, 0, 2, 7],
}
)
print(items_df)
Output:
Id Name Cost Discount(%)
0 302 Watch 300 10
1 504 Camera 400 15
2 708 Phone 350 5
3 103 Shoes 100 0
4 343 Laptop 1000 2
5 565 Bed 400 7
We will use the DataFrame displayed above in the code snippet to demonstrate how we can create new columns in Pandas DataFrame based on other columns’ values in the DataFrame.
Create New Columns in Pandas DataFrame Based on the Values of Other Columns Using the Element-Wise Operation
import pandas as pd
items_df = pd.DataFrame(
{
"Id": [302, 504, 708, 103, 343, 565],
"Name": ["Watch", "Camera", "Phone", "Shoes", "Laptop", "Bed"],
"Actual Price": [300, 400, 350, 100, 1000, 400],
"Discount(%)": [10, 15, 5, 0, 2, 7],
}
)
print("Initial DataFrame:")
print(items_df, "\n")
items_df["Final Price"] = items_df["Actual Price"] - (
(items_df["Discount(%)"] / 100) * items_df["Actual Price"]
)
print("DataFrame after addition of new column")
print(items_df, "\n")
Output:
Initial DataFrame:
Id Name Actual Price Discount(%)
0 302 Watch 300 10
1 504 Camera 400 15
2 708 Phone 350 5
3 103 Shoes 100 0
4 343 Laptop 1000 2
5 565 Bed 400 7
DataFrame after addition of new column
Id Name Actual Price Discount(%) Final Price
0 302 Watch 300 10 270.0
1 504 Camera 400 15 340.0
2 708 Phone 350 5 332.5
3 103 Shoes 100 0 100.0
4 343 Laptop 1000 2 980.0
5 565 Bed 400 7 372.0
It calculates each product’s final price by subtracting the value of the discount amount from the Actual Price
column in the DataFrame. Then it assigns the Series
of the final price values to the Final Price
column of the DataFrame items_df
.
Create New Columns in Pandas DataFrame Based on the Values of Other Columns Using the DataFrame.apply()
Method
import pandas as pd
items_df = pd.DataFrame(
{
"Id": [302, 504, 708, 103, 343, 565],
"Name": ["Watch", "Camera", "Phone", "Shoes", "Laptop", "Bed"],
"Actual_Price": [300, 400, 350, 100, 1000, 400],
"Discount_Percentage": [10, 15, 5, 0, 2, 7],
}
)
print("Initial DataFrame:")
print(items_df, "\n")
items_df["Final Price"] = items_df.apply(
lambda row: row.Actual_Price - ((row.Discount_Percentage / 100) * row.Actual_Price),
axis=1,
)
print("DataFrame after addition of new column")
print(items_df, "\n")
Output:
Initial DataFrame:
Id Name Actual_Price Discount_Percentage
0 302 Watch 300 10
1 504 Camera 400 15
2 708 Phone 350 5
3 103 Shoes 100 0
4 343 Laptop 1000 2
5 565 Bed 400 7
DataFrame after addition of new column
Id Name Actual_Price Discount_Percentage Final Price
0 302 Watch 300 10 270.0
1 504 Camera 400 15 340.0
2 708 Phone 350 5 332.5
3 103 Shoes 100 0 100.0
4 343 Laptop 1000 2 980.0
5 565 Bed 400 7 372.0
It applies the lambda function defined in the apply()
method to each row of the DataFrame items_df
and finally assigns the series of results to the Final Price
column of the DataFrame items_df
.
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn