How to Get the Row Count of a Pandas DataFrame
-
.shape
Method to Get Row Count ofDataFrame
-
.len(DataFrame.index)
as the Fastest Method to Get Row Count in Pandas -
dataframe.apply()
to Count Rows That Satisfy a Condition in Pandas
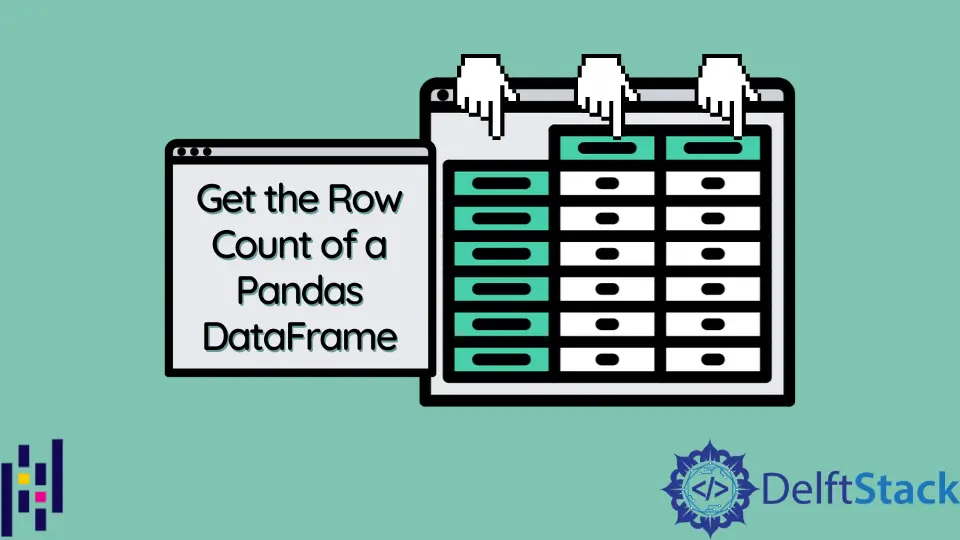
We will introduce how to get the row count of a Pandas DataFrame
, with different methods like shape
and len(DataFrame.index)
. They have notable performance differences and the len(DataFrame.index)
method is the fastest.
We also look at how we can use dataframe.apply()
to get how many elements of rows satisfies a condition or not.
.shape
Method to Get Row Count of DataFrame
Suppose df
is our DataFrame
, to calculate row count,
# python 3.x
import pandas as pd
import numpy as np
df = pd.DataFrame(np.arange(15).reshape(3, 5))
print(df)
print("Row count is:", df.shape[0])
Output:
0 1 2 3 4
0 0 1 2 3 4
1 5 6 7 8 9
2 10 11 12 13 14
Row count is: 3
For columns count, we can use df.shape[1]
.
.len(DataFrame.index)
as the Fastest Method to Get Row Count in Pandas
We can calculate the row count in the DataFrame
by getting the index row’s length.
# python 3.x
import pandas as pd
import numpy as np
df = pd.DataFrame(np.arange(15).reshape(3, 5))
print(df)
print("Row count is:", len(df.index))
Output:
0 1 2 3 4
0 0 1 2 3 4
1 5 6 7 8 9
2 10 11 12 13 14
Row count is: 3
We can also pass df.axes[0]
instead of df.index
:
# python 3.x
import pandas as pd
import numpy as np
df = pd.DataFrame(np.arange(15).reshape(3, 5))
print(df)
print("Row count is:", len(df.axes[0]))
Output:
0 1 2 3 4
0 0 1 2 3 4
1 5 6 7 8 9
2 10 11 12 13 14
Row count is: 3
For columns’ count we can use df.axes[1]
.
dataframe.apply()
to Count Rows That Satisfy a Condition in Pandas
By counting the number of True
in the returned result of dataframe.apply()
, we can get the count of rows in DataFrame
that satisfies the condition.
# python 3.x
import pandas as pd
import numpy as np
df = pd.DataFrame(np.arange(15).reshape(3, 5))
counterFunc = df.apply(lambda x: True if x[1] > 3 else False, axis=1)
numOfRows = len(counterFunc[counterFunc == True].index)
print(df)
print("Row count > 3 in column[1]is:", numOfRows)
Output:
0 1 2 3 4
0 0 1 2 3 4
1 5 6 7 8 9
2 10 11 12 13 14
Row count > 3 in column[1]is: 2
We get the count of rows whose value in column[1]
is greater than 3.
Related Article - Pandas DataFrame Row
- How to Randomly Shuffle DataFrame Rows in Pandas
- How to Filter Dataframe Rows Based on Column Values in Pandas
- How to Iterate Through Rows of a DataFrame in Pandas
- How to Get Index of All Rows Whose Particular Column Satisfies Given Condition in Pandas
- How to Find Duplicate Rows in a DataFrame Using Pandas