How to Extract Month and Year Separately From Datetime Column in Pandas
-
pandas.Series.dt.year()
andpandas.Series.dt.month()
Methods to Extract Month and Year -
strftime()
Method to Extract Year and Month -
pandas.DatetimeIndex.month
andpandas.DatetimeIndex.year
to Extract Year and Month
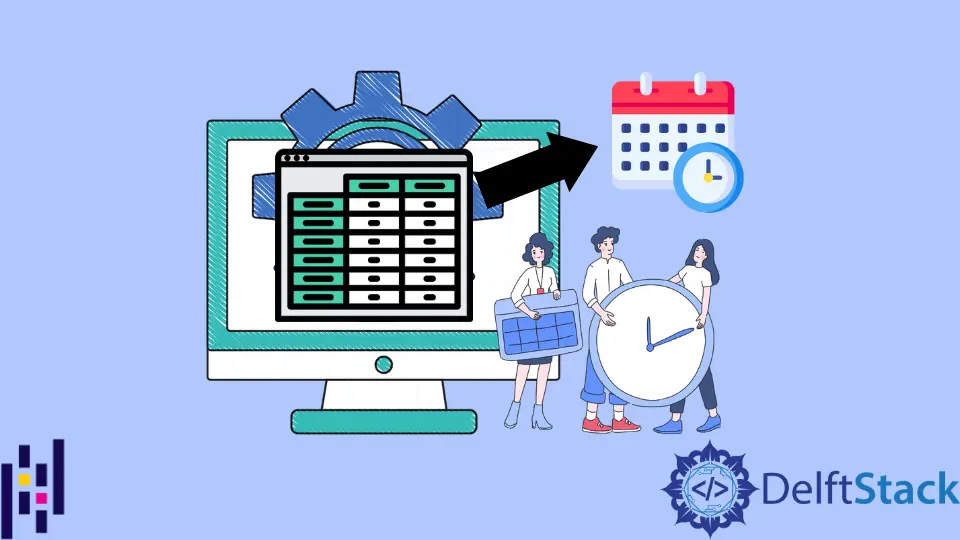
We could extract year and month from Datetime
column using pandas.Series.dt.year()
and pandas.Series.dt.month()
methods respectively. If the data isn’t in Datetime
type, we need to convert it firstly to Datetime
. We can also extract year and month using pandas.DatetimeIndex.month
along with pandas.DatetimeIndex.year
and strftime()
method.
pandas.Series.dt.year()
and pandas.Series.dt.month()
Methods to Extract Month and Year
pandas.Series.dt.year()
and pandas.Series.dt.month()
methods applied on Datetime
type returns NumPy
array of year and month respectively of the Datetime
entry in the series object.
import pandas as pd
import numpy as np
import datetime
list_of_dates = ["2019-11-20", "2020-01-02", "2020-02-05", "2020-03-10", "2020-04-16"]
employees = ["Hisila", "Shristi", "Zeppy", "Alina", "Jerry"]
df = pd.DataFrame({"Joined date": pd.to_datetime(list_of_dates)}, index=employees)
df["Year"] = df["Joined date"].dt.year
df["Month"] = df["Joined date"].dt.month
print(df)
Output:
Joined date Year Month
Hisila 2019-11-20 2019 11
Shristi 2020-01-02 2020 1
Zeppy 2020-02-05 2020 2
Alina 2020-03-10 2020 3
Jerry 2020-04-16 2020 4
However, if the column is not Datetime
type, we should first convert the column to Datetime
type using the to_datetime()
method.
import pandas as pd
import numpy as np
import datetime
list_of_dates = ["11/20/2019", "01/02/2020", "02/05/2020", "03/10/2020", "04/16/2020"]
employees = ["Hisila", "Shristi", "Zeppy", "Alina", "Jerry"]
df = pd.DataFrame({"Joined date": pd.to_datetime(list_of_dates)}, index=employees)
df["Joined date"] = pd.to_datetime(df["Joined date"])
df["Year"] = df["Joined date"].dt.year
df["Month"] = df["Joined date"].dt.month
print(df)
Output:
Joined date Year Month
Hisila 2019-11-20 2019 11
Shristi 2020-01-02 2020 1
Zeppy 2020-02-05 2020 2
Alina 2020-03-10 2020 3
Jerry 2020-04-16 2020 4
strftime()
Method to Extract Year and Month
The strftime()
method takes Datetime takes format codes as input and returns a string representing the specific format specified in output. We use %Y
and %m
as format codes to extract year and month.
import pandas as pd
import numpy as np
import datetime
list_of_dates = ["2019-11-20", "2020-01-02", "2020-02-05", "2020-03-10", "2020-04-16"]
employees = ["Hisila", "Shristi", "Zeppy", "Alina", "Jerry"]
df = pd.DataFrame({"Joined date": pd.to_datetime(list_of_dates)}, index=employees)
df["year"] = df["Joined date"].dt.strftime("%Y")
df["month"] = df["Joined date"].dt.strftime("%m")
print(df)
Output:
Joined date year month
Hisila 2019-11-20 2019 11
Shristi 2020-01-02 2020 01
Zeppy 2020-02-05 2020 02
Alina 2020-03-10 2020 03
Jerry 2020-04-16 2020 04
pandas.DatetimeIndex.month
and pandas.DatetimeIndex.year
to Extract Year and Month
Another simple approach to extract the month and year from the Datetime
column is by retrieving values of year and month attributes of objects of pandas.DatetimeIndex
class.
import pandas as pd
import numpy as np
import datetime
list_of_dates = ["2019-11-20", "2020-01-02", "2020-02-05", "2020-03-10", "2020-04-16"]
employees = ["Hisila", "Shristi", "Zeppy", "Alina", "Jerry"]
df = pd.DataFrame({"Joined date": pd.to_datetime(list_of_dates)}, index=employees)
df["year"] = pd.DatetimeIndex(df["Joined date"]).year
df["month"] = pd.DatetimeIndex(df["Joined date"]).month
print(df)
Output:
Joined date Year Month
Hisila 2019-11-20 2019 11
Shristi 2020-01-02 2020 1
Zeppy 2020-02-05 2020 2
Alina 2020-03-10 2020 3
Jerry 2020-04-16 2020 4
pandas.DatetimeIndex
class is an immutable ndarray of datetime64 data. It has attributes like year
, month
, day
, etc.
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn