How to Run a Flask App
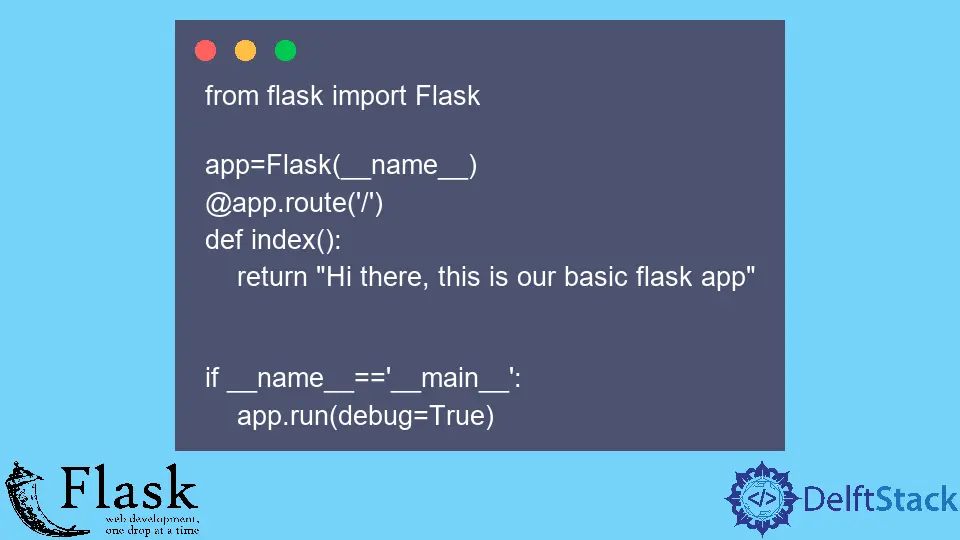
With this explanation, we will learn how to set up a Flask environment in Visual Studio Code and create a basic application of Flask.
Create a Basic Flask Application
Flask is a micro web framework of Python and a famous framework for building a web application. It is also well known for creating rest APIs.
Let’s get into the work; before starting, you must make sure that you have Python installed on your machine. If an installation is needed, you can go to the official Python website and download the latest version and after that, select the OS you have and perform the installation.
Once you have performed the installation, you must check if you have successfully installed Python onto your machine. You can go to the command prompt and type the following command.
python --version
If your Python version is displaying, then we will use Python libraries. We are using Visual Studio Code because this is a lightweight editor, but you can go to write the code with any editor.
Once we create a new folder, we will open up the VS Code environment inside this directory. Then, create a virtual environment, and why do we need to create a virtual environment?
A virtual environment is like an isolated environment on your machine; if you are working with a Python script in a virtual environment using a different version of libraries and dependencies, all are stored in one containerized isolated environment on your machine.
It does not interfere with other projects and system settings, which is why it is useful when working on a serious project. Now we will be going ahead and setting up our virtual environment; we need to press Ctrl+` to launch the terminal.
Once we do that, we can type the following command to install the virtual environment module.
pip install virtualenv
After successful installation, we need to create a virtual environment and use the virtualenv env
, env
is the name of our virtual environment. When we create a virtual environment, we need to name it.
Next, we need to activate the environment or enter it; now, we will write the following command.
\env\Scripts\activate.bat
We have entered into our virtual environment; if you are on Mac OS, use the below command to activate your virtual environment.
source env\bin\activate
Now we can go ahead and install the Flask using the below command.
pip install flask
When the Flask has been successfully installed, we made all our installations in this virtual environment that is separate from our machine. We need to create our main app; we will call it the app.py
file.
We will write the most basic Flask application to demonstrate how we can get a quick Flask application and make it able to run the webpage.
The first thing is needed to import the Flask, so the first Flask is lowercase, which is the library, and then the second is in uppercase, the class for the Flask. Now we need to instantiate this Flask
class and app
equal to Flask(__name__)
that references the name of the current app.
Now we need to create something that is called a route which is just the URL endpoint for a particular webpage in our app. We will just put a slash in this route @app.route('/')
, but you can put whatever you want here. It can be something like page name or endpoint name.
Then define a function, and the name can be whatever you want, but you name it something descriptive. In this case, this is the index()
, which means our website’s base URL; then, we will write a return
statement that returns a string.
The last thing is to run the application by the Python interpreter; the __name__=='__main__'
variable is set to the actual name of the Python file. The if
statement here ensures the server only runs if the script is executed directly from the Python interpreter.
We are jumping to the last line of our code; we use the run()
function to run the local server with our application.
In our case, we are just going to set debug
to True
, so this is a way to get to know the errors but remember, when you launch a Flask application into a production environment, you always have to set debug
to False
.
from flask import Flask
app = Flask(__name__)
@app.route("/")
def index():
return "Hi there, this is our basic flask app"
if __name__ == "__main__":
app.run(debug=True)
Now we are good to go ahead and launch our application by typing the command that is shown below. When we hit the Enter and get a localhost address, we need to copy and paste it into our browser where we put the URL.
Output:
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn