How to Remove Item From an Array in PowerShell
-
Use the
Remove()
Method to Remove Item From anArrayList
in PowerShell -
Use the
RemoveAt()
Method to Remove Item From anArrayList
in PowerShell -
Use the
RemoveRange()
Method to Remove Item From anArrayList
in PowerShell -
Use the
ForEach-Object
Method to Remove Items From anArrayList
in PowerShell - Conclusion
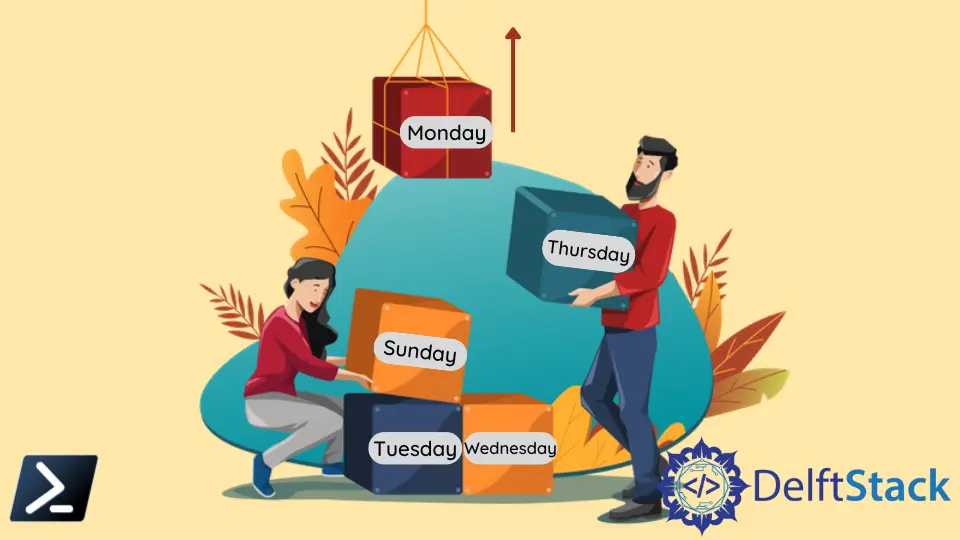
Managing and manipulating data structures like arrays is a common task in PowerShell scripting. When it comes to removing items from an ArrayList
, PowerShell provides various methods to suit different needs and scenarios.
This article delves into four distinct methods: Remove()
, RemoveAt()
, RemoveRange()
, and ForEach-Object
, each offering unique capabilities for removing items based on different criteria. From removing specific values to eliminating elements at specific index positions, PowerShell users can choose the most appropriate method for their array manipulation requirements.
Use the Remove()
Method to Remove Item From an ArrayList
in PowerShell
In PowerShell, the Remove()
method provides a straightforward way to eliminate specific elements from an array. This method is particularly useful when you know the exact value you want to remove from the array.
Whether you’re managing a list of files, user names, or any other type of data, the Remove()
method allows for efficient manipulation of array contents.
Example:
# Create an ArrayList
$myArrayList = [System.Collections.ArrayList]@("apple", "banana", "cherry")
# Remove "banana" from the ArrayList
$myArrayList.Remove("banana")
# Display the modified ArrayList
$myArrayList
In this code snippet, we’ve utilized the Remove()
method on an ArrayList
in PowerShell to eliminate a specific item from the collection. We first initialize an ArrayList
named $myArrayList
containing three elements: apple
, banana
, and cherry
.
Subsequently, we invoke the Remove()
method on $myArrayList
, specifying banana
as the argument to be removed. This action effectively removes banana
from the ArrayList
, adjusting the ArrayList
’s structure accordingly.
Finally, we print the modified ArrayList
to verify the removal. By leveraging the Remove()
method with ArrayLists
, we can seamlessly manage and manipulate dynamic collections of data in PowerShell scripts, ensuring precise control over the contents of the ArrayList
.
Output:
Use the RemoveAt()
Method to Remove Item From an ArrayList
in PowerShell
The RemoveAt()
method in PowerShell is used to remove an element from an array at a specific index position. This method modifies the original array by removing the element at the specified index and shifting all subsequent elements to the left to fill the gap.
The index parameter specifies the position of the element to remove, starting from 0 for the first element in the array.
Example:
# Create an ArrayList
$myArrayList = [System.Collections.ArrayList]@("apple", "banana", "cherry")
# Remove the item at index 1 ("banana") from the ArrayList
$myArrayList.RemoveAt(1)
# Display the modified ArrayList
$myArrayList
In this code snippet, we’ve utilized the RemoveAt()
method on an ArrayList
in PowerShell to remove a specific item based on its index position within the collection. By initializing an ArrayList
named $myArrayList
with three elements (apple
, banana
, and cherry
), we showcase how to precisely remove the item at index 1 (banana
) from the ArrayList
.
Using the RemoveAt()
method enables us to dynamically modify ArrayList
contents by specifying the index of the item we want to remove. After invoking the method, we print the modified ArrayList
to confirm the removal.
Leveraging the RemoveAt()
method provides a straightforward approach to managing ArrayList
elements by their index positions, facilitating efficient array manipulation in PowerShell scripts.
Output:
Use the RemoveRange()
Method to Remove Item From an ArrayList
in PowerShell
The RemoveAt()
method in PowerShell is used to remove an element from an array at a specific index position. This method modifies the original array by removing the element at the specified index and shifting all subsequent elements to the left to fill the gap.
The index parameter specifies the position of the element to remove, starting from 0 for the first element in the array.
Example:
# Create an ArrayList
$myArrayList = [System.Collections.ArrayList]@("apple", "banana", "cherry", "date", "elderberry")
# Remove elements starting from index 1 up to index 2
$myArrayList.RemoveRange(1, 2)
# Display the modified ArrayList
$myArrayList
In this code snippet, we’ve utilized the RemoveRange()
method on an ArrayList
in PowerShell to remove a range of elements based on their index positions within the collection. By initializing an ArrayList
named $myArrayList
with five elements (apple
, banana
, cherry
, date
, and elderberry
), we demonstrate how to remove elements starting from index 1 up to index 2 (banana
and cherry
).
By specifying the starting index and the count of elements to remove, the RemoveRange()
method efficiently eliminates a contiguous block of elements from the ArrayList
. After invoking the method, we print the modified ArrayList
to confirm the removal.
Leveraging the RemoveRange()
method provides a convenient way to manage ArrayList
contents by removing multiple items simultaneously, facilitating efficient array manipulation in PowerShell scripts.
Output:
Use the ForEach-Object
Method to Remove Items From an ArrayList
in PowerShell
In PowerShell, the ForEach-Object
cmdlet provides a convenient way to iterate through elements of an ArrayList
and perform operations on each item individually. When it comes to removing items from an ArrayList
based on certain criteria, ForEach-Object
can be effectively utilized.
By combining ForEach-Object
with conditional logic, we can selectively remove items from the ArrayList
, offering flexibility in managing the collection’s contents.
Example:
# Create an ArrayList
$myArrayList = [System.Collections.ArrayList]@('apple', 'banana', 'cherry', 'date', 'elderberry')
# Remove items containing the letter 'a'
$myArrayList = $myArrayList | ForEach-Object {
if ($_ -notlike "*a*") {
$_
}
}
# Display the modified ArrayList
$myArrayList
In this code snippet, we start by initializing an ArrayList
named $myArrayList
with five elements: apple
, banana
, cherry
, date
, and elderberry
. We then utilize the ForEach-Object
cmdlet to iterate through each item in $myArrayList
.
Within the loop, a conditional logic (-notlike "*a*"
) is applied to filter out items containing the letter a
. Those items that meet the condition are retained, while those that do not are implicitly removed from the ArrayList
.
Finally, we print the modified ArrayList
to observe the result, showcasing how ForEach-Object
can selectively remove items based on specific criteria, offering flexibility in managing ArrayList
contents in PowerShell scripts.
Output:
Conclusion
Removing items from an ArrayList
in PowerShell is a task that can be approached in several ways, depending on the specific needs of the script or automation task. The Remove()
, RemoveAt()
, RemoveRange()
, and ForEach-Object
methods provide PowerShell users with a range of options for efficiently managing array contents.
Whether removing items based on their values, index positions, or specific criteria, PowerShell’s array manipulation capabilities offer flexibility and efficiency. By understanding and leveraging these methods, PowerShell users can streamline their scripts and effectively manipulate ArrayLists
to suit their requirements, enhancing productivity and enabling precise control over array contents.