How to Get File Version in PowerShell
-
Use
Get-Item
to Get File Version in PowerShell -
Use
Get-ChildItem
to Get File Version in PowerShell -
Use
Get-Command
to Get File Version in PowerShell -
Use
System.Diagnostics.FileVersionInfo
to Get the File Version in PowerShell - Conclusion
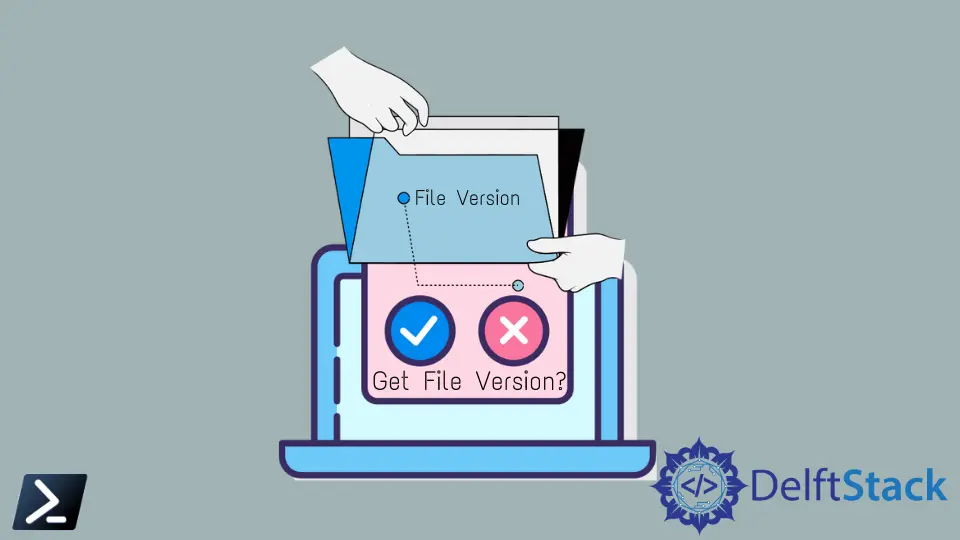
A file’s version number is a critical piece of information, especially when managing and troubleshooting software and system files. In PowerShell, you can easily retrieve this version information using various cmdlets and methods.
The executable files contain version information, such as .exe
and .dll
. Note that text files do not have any version information.
The version information holds the file name, file version, company name, product name, product version, and language. This tutorial will teach you to get the file version in PowerShell.
Use Get-Item
to Get File Version in PowerShell
PowerShell provides multiple cmdlets and methods to get the file version, and Get-Item
is one of them. This method is one of the simplest and easiest to use.
Syntax:
(Get-Item "Path\to\file").VersionInfo.FileVersion
Get-Item
: This is a cmdlet in PowerShell used to retrieve information about a file or directory at a specified path. This only obtains information about a single file or directory."Path\to\file"
: This is the argument provided toGet-Item
. It specifies the path to the file or directory that is to be inspected..VersionInfo
: This is the property that allows you to access and inspect the version information..FileVersion
: This is a sub-property of theVersionInfo
property that specifically retrieves the file version of a file.
The following example shows how to use Get-Item
and VersionInfo.FileVersion
to get the version of a file C:\P/rogram Files\Google\Chrome\Application\chrome.exe
.
(Get-Item "C:\Program Files\Google\Chrome\Application\chrome.exe").VersionInfo.FileVersion
The (Get-Item "C:\Program Files\Google\Chrome\Application\chrome.exe")
line uses the Get-Item
cmdlet to retrieve information about the file located at the specified path, which is the Chrome executable (chrome.exe
) in the given directory. Then, once the file information is obtained, the code accesses the VersionInfo
property of the file, which contains various details about the file, including its version information.
Lastly, the .FileVersion
retrieves the specific piece of information we are interested in, which is the file version of the Chrome executable.
Output:
98.0.4758.102
The output 98.0.4758.102
represents the file version of the Chrome executable at the specified path. In this case, it indicates that the version of Google Chrome installed on the system is 98.0.4758.102
.
Use Get-ChildItem
to Get File Version in PowerShell
The Get-ChildItem
gets the items and child items in one or more specified locations. We can also use Get-ChildItem
with the VersionInfo.FileVersion
property to get the file version in PowerShell.
Syntax:
(Get-ChildItem "Path\to\file").VersionInfo.FileVersion
Get-ChildItem
: This cmdlet is used to list files and directories in a specified location."Path\to\file"
: This is the argument provided toGet-ChildItem
. It specifies the path to the file or directory you want to inspect..VersionInfo
: This property allows you to access and inspect the version information..FileVersion
: This is a sub-property ofVersionInfo
that specifically retrieves the file version of a file.
Example:
(Get-ChildItem "C:\Program Files\Google\Chrome\Application\chrome.exe").VersionInfo.FileVersion
The (Get-ChildItem "C:\Program Files\Google\Chrome\Application\chrome.exe")
line uses the Get-ChildItem
cmdlet to retrieve information about the file located at the specified path, which is the Chrome executable (chrome.exe
) in the given directory. The Get-ChildItem
is used to list files and directories within a specified location.
Next, once the file information is obtained, the code accesses the VersionInfo
property of the file, which contains various details about the file, including its version information. Lastly, the .FileVersion
retrieves the specific piece of information we are interested in, which is the file version of the Chrome executable.
Output:
98.0.4758.102
The output 98.0.4758.102
represents the file version of the Chrome executable at the specified path. In this case, it indicates that the version of Google Chrome installed on the system is 98.0.4758.102
.
Use Get-Command
to Get File Version in PowerShell
The Get-Command
cmdlet gets all commands installed on the computer. It includes all cmdlets, aliases, functions, scripts, and applications.
We can use the FileVersionInfo.FileVersion
property with the Get-Command
to get the file version in PowerShell.
Syntax:
(Get-Command "Path\to\file").FileVersionInfo.FileVersion
Get-Command
: This is the cmdlet used to retrieve the information about a command, script, or executable file. It can be used to find information about PowerShell commands, functions, or external executable files, including their location properties."Path\to\file"
: This is the argument provided toGet-Command
. It specifies the path to the command, script, or executable file you want to inspect..FileVersionInfo
: This property provides details about the version information of the command or script..FileVersion
: This is a sub-property of theFileVersionInfo
property. It specifically retrieves the file version of the command or script.
The following command gets the file version number of the C:\Windows\System32\ActionCenter.dll
file:
(Get-Command C:\Windows\System32\ActionCenter.dll).FileVersionInfo.FileVersion
The (Get-Command C:\Windows\System32\ActionCenter.dll)
uses the Get-Command
cmdlet to retrieve information about the specified command (in this case, the DLL file). When we provide a file path as an argument to Get-Command
, it returns information about the file, including its properties.
Then, once the file information is obtained, the code accesses the FileVersionInfo
property of the file object. The FileVersionInfo
property contains various details about the file, including its version information.
Lastly, the .FileVersion
retrieves the specific piece of information we are interested in, which is the file version of the ActionCenter.dll
file.
Output:
10.0.19041.1 (WinBuild.160101.0800)
The output 10.0.19041.1 (WinBuild.160101.0800)
represents the file version of the ActionCenter.dll
file at the specified path. In this case, it indicates that the version of the ActionCenter.dll
file on the system is 10.0.19041.1 (WinBuild.160101.0800)
.
Use System.Diagnostics.FileVersionInfo
to Get the File Version in PowerShell
This method is invoked with the file path (the actual file path you want to retrieve the version information) as an argument. This method call retrieves version information about the specified file.
The System.Diagnostics.FileVersionInfo
class from the .NET
Framework provides version information of a file. We can use the GetVersionInfo()
method and the FileVersion
property to get the file version number.
Syntax:
[System.Diagnostics.FileVersionInfo]::GetVersionInfo("Path\to\file").FileVersion
[System.Diagnostics.FileVersionInfo]
: This part specifies the .NET Framework class that is used to access file version information. This .NET class provides access to version information about files, typically executable files, but it can be used for other types of files as well.GetVersionInfo
: This is the method that retrieves the version information for the file specified in the argument."Path\to\file"
: This is the argument provided to theGetVersionInfo
method. This specifies the exact path of the file you want to inspect..FileVersion
: This property specifically retrieves the file version of the file. The file version is typically a numerical value that represents the version of the file or software.
Example:
[System.Diagnostics.FileVersionInfo]::GetVersionInfo("C:\Windows\System32\ActionCenter.dll").FileVersion
The [System.Diagnostics.FileVersionInfo]::GetVersionInfo("C:\Windows\System32\ActionCenter.dll")
line uses the [System.Diagnostics.FileVersionInfo]
class, which is part of the .NET Framework, to obtain version information about the specified file. The ::
syntax is used to access the static method GetVersionInfo
of the FileVersionInfo
class, and the path "C:\Windows\System32\ActionCenter.dll"
is provided as an argument to this method.
Then, once the version information is obtained, the code accesses the FileVersion
property of the FileVersionInfo
object. Lastly, the FileVersion
property contains a string representing the version of the file.
Output:
10.0.19041.1 (WinBuild.160101.0800)
The output 10.0.19041.1 (WinBuild.160101.0800)
represents the file version of the ActionCenter.dll
file. In this case, it indicates that the version of the ActionCenter.dll
file on the system is 10.0.19041.1 (WinBuild.160101.0800)
.
Conclusion
Mastering the retrieval of file version information in PowerShell is a fundamental skill for efficient file management and effective troubleshooting. Throughout this article, we’ve learned four methods that allow us to extract data effortlessly.
Whether we choose to use the Get-Item
, Get-ChildItem
, Get-Command
, or the System.Diagnostics.FileVersionInfo
approach, we now have a versatile toolkit for obtaining file version details.