Array of Strings in PowerShell
- PowerShell Array of Strings
- Method 1: Declare Array of String Using Built-In Method
-
Method 2: Declare Array of String Using
System.Collections.Arraylist
- Get the Length of the Array of Strings
- Get the Type of Array of Strings
- Add Strings to Array of Strings
- Find a String Inside an Array of Strings
- Change Case of Strings Inside Array of Strings
- Get All Existing Methods for an Array of Strings
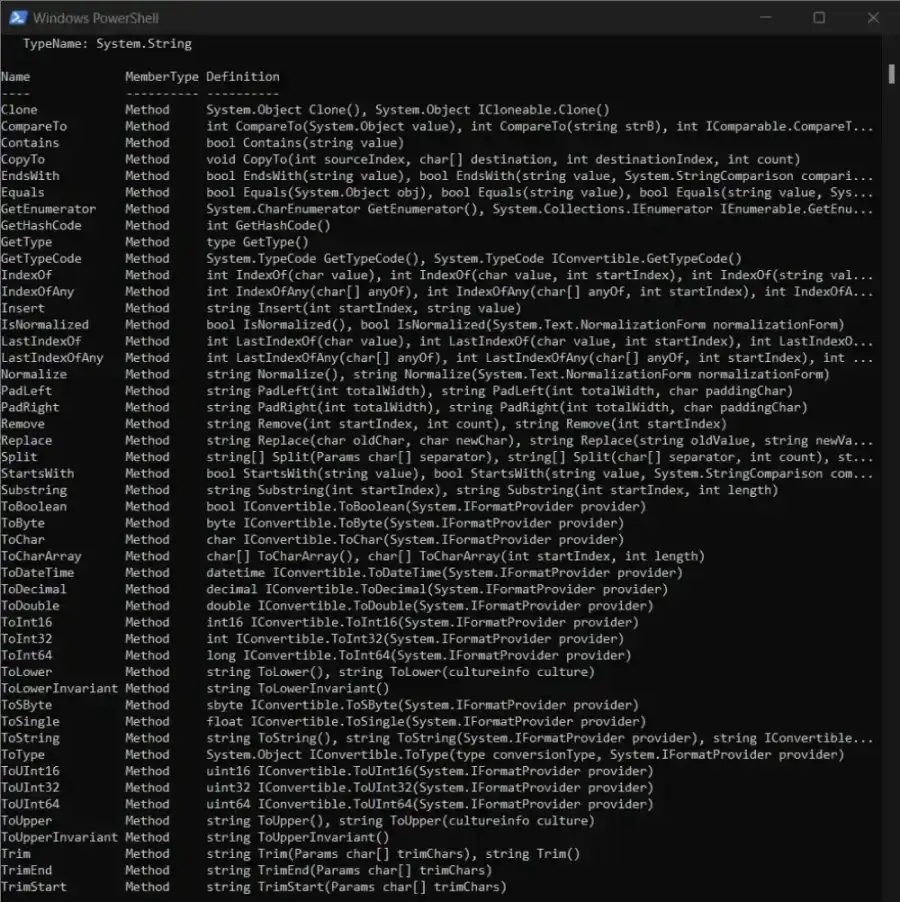
This tutorial demonstrates how to create an array of strings in PowerShell.
PowerShell Array of Strings
There are different methods to declare an array of strings in PowerShell. This tutorial demonstrates different methods to declare an array of strings and how to access the array of strings.
Method 1: Declare Array of String Using Built-In Method
The first method is to declare an array of strings by the built-in PowerShell method, where we declare an array of strings in one line. See the command:
$DemoArray = @("This is Delftstack1", "This is Delftstack2", "This is Delftstack3", "This is Delftstack4")
echo $DemoArray
The code above will create an array of strings and then print it. See the output:
This is Delftstack1
This is Delftstack2
This is Delftstack3
This is Delftstack4
Method 2: Declare Array of String Using System.Collections.Arraylist
The System.Collections.Arraylist
is a PowerShell class used to create arrays and array lists. To use this class, first, we need to create an object of this class.
See the commands:
New-Object -TypeName System.Collections.Arraylist
$DemoArray = [System.Collections.Arraylist]@("This is Delftstack1", "This is Delftstack2", "This is Delftstack3", "This is Delftstack4")
echo $DemoArray
The code above will create an ArrayList
of strings using PowerShell’s System.Collections.Arraylist
class. See the output:
This is Delftstack1
This is Delftstack2
This is Delftstack3
This is Delftstack4
Get the Length of the Array of Strings
As we have declared the array of strings in the variable $DemoArray
, we have to use the Length
method with the variable to get this length. See the command:
$DemoArray.Length
Make sure you run this Length
method on the built-in method. See the output:
19
19
19
19
Using this Length
method on the System.Collections.Arraylist
array of strings will show each string’s length. See the example:
New-Object -TypeName System.Collections.Arraylist
$DemoArray = [System.Collections.Arraylist]@("This is Delftstack1", "This is Delftstack2", "This is Delftstack3", "This is Delftstack4")
$DemoArray.Length
The above code will output the length of each string in the array. See the output:
19
19
19
19
Get the Type of Array of Strings
We can use the GetType()
method of PowerShell to get an array type. Let’s try to get the type of array of strings for both the methods above.
Method 1:
$DemoArray = @("This is Delftstack1", "This is Delftstack2", "This is Delftstack3", "This is Delftstack4")
$DemoArray.GetType()
The code above will get the type of array of strings using the first method. See the output:
IsPublic IsSerial Name BaseType
-------- -------- ---- --------
True True Object[] System.Array
Method 2:
$DemoArray = [System.Collections.Arraylist]@("This is Delftstack1", "This is Delftstack2", "This is Delftstack3", "This is Delftstack4")
$DemoArray.GetType()
The code above will get the type of array of strings using the System.Collections.Arraylist
method. See the output:
IsPublic IsSerial Name BaseType
-------- -------- ---- --------
True True ArrayList System.Object
The name of the data type is stored in the Name
column, so for the first method, the data type is Object
, and for the second method, the data type is ArrayList
. The BaseType
column shows the data type in the system.
For an array, it is always System.Array
, and for string or ArrayList
, it will be System.Object
.
Add Strings to Array of Strings
Adding or appending more strings to an array of strings can be done using the +=
concatenation operator. For example, if we want to add a few more strings to the array, then we use the following command:
$DemoArray += @("This is Delftstack5", "This is Delftstack6", "This is Delftstack7", "This is Delftstack8")
Let’s try an example where we add more strings to the array, check the length and print the array. See the example:
$DemoArray = @("This is Delftstack1", "This is Delftstack2", "This is Delftstack3", "This is Delftstack4")
echo $DemoArray
$DemoArray += @("This is Delftstack5", "This is Delftstack6", "This is Delftstack7", "This is Delftstack8")
$DemoArray.Length
echo $DemoArray
The code above will create an array of strings and print that and then add four more strings to it, check the array’s length, and finally print the array. See the output:
This is Delftstack1
This is Delftstack2
This is Delftstack3
This is Delftstack4
8
This is Delftstack1
This is Delftstack2
This is Delftstack3
This is Delftstack4
This is Delftstack5
This is Delftstack6
This is Delftstack7
This is Delftstack8
Find a String Inside an Array of Strings
Finding a string inside an array of strings in PowerShell is easy. We have to use the method Contains()
, where the parameter will be the string we want to find.
The method will return true
if it finds the string; otherwise, it returns false
. Let’s try an example with our array:
$DemoArray = @("This is Delftstack1", "This is Delftstack2", "This is Delftstack3", "This is Delftstack4")
$DemoArray.Contains("This is Delftstack1")
$DemoArray.Contains("This is Delftstack5")
The method will look for the given strings in the method contains()
and will return true
if it finds the string and return false
if it can’t. See the output:
True
False
Change Case of Strings Inside Array of Strings
We can also change the case of strings inside the array of strings. For this purpose, we use the methods toLower()
and toUpper()
, which convert the case to lower and upper, respectively.
Let’s try an example:
$DemoArray = @("This is Delftstack1", "This is Delftstack2", "This is Delftstack3", "This is Delftstack4")
$DemoArray = $DemoArray.toLower()
echo $DemoArray
$DemoArray = $DemoArray.toUpper()
echo $DemoArray
The above commands will convert the array string to lower and upper case. See the outputs:
this is delftstack1
this is delftstack2
this is delftstack3
this is delftstack4
THIS IS DELFTSTACK1
THIS IS DELFTSTACK2
THIS IS DELFTSTACK3
THIS IS DELFTSTACK4
Get All Existing Methods for an Array of Strings
We can use the methods Get-Member
and -MemberType
to get all the methods available for the array of strings. To get all the methods available for the array of string run the following command:
$DemoArray | Get-Member -MemberType Method
The above code will get all the methods available for the array $DemoArray
. See the output:
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn FacebookRelated Article - PowerShell Array
- How to Create an Empty Array of Arrays in PowerShell
- Byte Array in PowerShell
- How to Pass an Array to a Function in PowerShell
- How to Sorting Array Values Using PowerShell
- How to Count the Length of Array in PowerShell