Null Variables in PowerShell
- Introduction to Null Variable in PowerShell
- Impacts of the Null Variable in PowerShell
- Checking for Null Values in PowerShell
- Conclusion
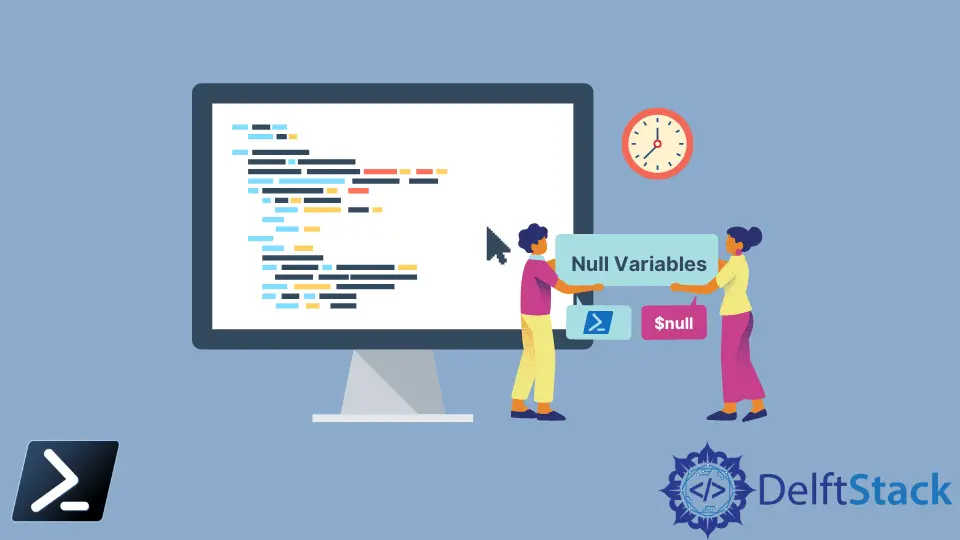
PowerShell treats the $Null
object with the value null, and some commands require some output to generate. They generate the value to null if there is any error and can also be helpful in the troubleshooting purpose in the scripts to check if the command generates any value.
This article talks about the null variable, the impacts of using the null variable in different syntax in PowerShell, and ways of checking the null values.
Introduction to Null Variable in PowerShell
We can think of NULL as an unknown or empty value. For example, a variable is NULL until you assign a value or object.
This variable can be important because some commands require a value and will fail if the value is NULL.
The $null
variable is an automatic variable in PowerShell used to represent NULL. We can assign it to variables, use it in comparisons, and use it as a placeholder for NULL in a collection.
PowerShell treats $null
as an object with the NULL value. Therefore, this variable is different than what you may expect if you come from another language.
Impacts of the Null Variable in PowerShell
The behavior of $null
values can vary depending on where they appear within your code. Let’s explore how NULL variables affect different aspects of PowerShell scripting.
Impact on Strings
If you use $null
in a string, it will be an empty string (or a blank value).
$value = $null
Write-Output "The value is $value."
Output:
The value is .
In order to maintain clarity in your scripts, it’s advisable to enclose variables in brackets ([]
) when using them in log messages, especially when the variable’s value is at the end of the string.
$value = $null
Write-Output "The value is [$value]"
Output:
The value is []
This practice helps distinguish between empty strings and $null
values.
Impact on Numerical Equations
When a $null
variable is used in a numeric equation, our results will be invalid if they do not give an error. Sometimes, the $null
variable will evaluate to 0
, or it will make the whole result $null
.
Consider this example involving multiplication, which yields either 0
or $null
based on the order of the values.
$null * 5
$null -eq ( $null * 5 )
Output:
True
Impact on Collections
A collection allows us to use an index to access values. If we try to index into a null collection, we will get this error "Cannot index into a null array"
.
$value = $null
$value[10]
Output:
Cannot index into a null array.
At line:2 char:1
+ $value[10]
+ ~~~~~~~~~~
+ CategoryInfo : InvalidOperation: (:) [], RuntimeException
+ FullyQualifiedErrorId : NullArray
However, if you have a collection and attempt to retrieve an element that doesn’t exist in the collection, you will receive a $null
result.
$array = @( 'one', 'two', 'three' )
$null -eq $array[100]
Output:
True
Impacts on Objects
If we try to access a property or sub-property of an object with no specified property, we can get a $null
variable like you would for an undefined variable. It does not matter if the value is $null
or an actual object in this case.
$null -eq $undefined.not.existing
Output:
True
Impacts on Methods
Calling a method on a $null
variable will throw a RuntimeException
exception.
$value = $null
$value.toString()
Output:
You cannot call a method on a null valued expression.
At line:2 char:1
+ $value.toString()
+ ~~~~~~~~~~~~~~~~~
+ CategoryInfo : InvalidOperation: (:) [], RuntimeException
+ FullyQualifiedErrorId : InvokeMethodOnNull
Whenever you encounter the phrase "You cannot call a method on a null valued expression"
, it’s a signal to inspect your code for places where a method is being called on a variable without first checking for $null
values.
Checking for Null Values in PowerShell
Use Comparison Operators -eq
and -ne
to Check for Null Values in PowerShell
When checking for $null
values in PowerShell, it’s a best practice to place the $null
variable on the left side of comparisons. This style of writing is not only intentional but also accepted as a PowerShell best practice.
However, there are scenarios where placing $null
on the right side may give you unexpected results. Let us look at the following code and try to predict the results:
if ( $value -eq $null ) {
'The array is $null'
}
if ( $value -ne $null ) {
'The array is not $null'
}
If we do not define $value
, the first one will evaluate $true
, and our message will be The array is $null
. The caveat here is that creating a $value
that will allow both results to be $false
is possible.
Output:
The array is $null
In this case, the $value
variable is an array that contains a $null
.
The -eq
operator checks every value in the array and returns the matched $null
, which evaluates to $false
. Similarly, the -ne
operator returns everything that does not match the $null
value, resulting in no results (also evaluating to $false
).
Therefore, neither condition is $true
, even though one of them might be expected to be.
Use the -not
Operator to Check for Null Values in PowerShell
The -not
operator is a logical operator in PowerShell that negates a condition. When applied to a variable, it checks if the variable is not null.
$myVariable = $null
if (-not $myVariable) {
Write-Host "The variable is null."
}
In this code snippet, we have a variable $myVariable
initialized with a null value. The-not
operator is used in the if
statement to check if $myVariable
is not null.
If the condition is true, the script will run the code block within the if
statement.
Output:
The variable is null.
As demonstrated in the output, the script identifies that $myVariable
is null and prints the message "The variable is null"
.
Use the IsNullOrEmpty()
Method to Check for Null Values in PowerShell
The IsNullOrEmpty()
is a method of the System.String
class, not a native PowerShell method. Different data types may have different ways to check for null or empty values.
In this code, we create a variable called $myString
and assign it the value $null
, which represents a null or empty string.
We use the [string]::IsNullOrEmpty($myString)
method to check if $myString
is null or empty. This method is part of the [string]
class in the .NET Framework and is used to determine if a string is either null or an empty string.
If $myString
is indeed null or empty, the condition is true, and the script prints "The string is null or empty."
.
$myString = $null
if ([string]::IsNullOrEmpty($myString)) {
Write-Host 'The string is null or empty.'
}
Output:
The string is null or empty.
Since $myString
is assigned the value $null
, the condition is true, and the message is displayed in the output.
Use the IsNullOrWhiteSpace()
Method to Check for Null Values in PowerShell
The IsNullOrWhiteSpace()
is a useful method available in the .NET Framework’s System.String
class, and you can use it in PowerShell by calling it on a string variable.
This method checks whether a string is either null, empty, or only consists of whitespace characters. It’s handy when you want to check if a string variable is effectively empty, even if it contains only spaces or tabs.
In this code below, we create a variable called $myString
and assign it a string that contains only whitespace characters (spaces).
Then, we use the [string]::IsNullOrWhiteSpace($myString)
method to check if $myString
is null or contains only whitespace.
$myString = $null
if ([string]::IsNullOrWhiteSpace($myString)) {
Write-Host 'The string is null or contains only whitespace.'
}
Output:
The string is null or contains only whitespace.
Since the condition is true ($myString
is null), the script prints "The string is null or contains only whitespace."
.
This method is helpful when you want to ensure that a string is truly empty, even if it appears to be "blank"
due to spaces or tabs.
Conclusion
In PowerShell scripting, understanding how $null
variables work is important because they can affect how your scripts behave in different situations. To write reliable scripts, it’s a good idea to follow best practices, like making sure you check for null values correctly by placing $null
on the left side.
Whether you’re new to scripting or have experience, knowing these details will help you become better at using PowerShell.
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn