How to Get Random Array Item in PHP
-
Use the
array_rand()
Function to Get a Random Array Item in PHP -
Use the
mt_rand()
Function to Get a Random Array Item in PHP -
Use the
shuffle()
Function to Get a Random Array Item in PHP
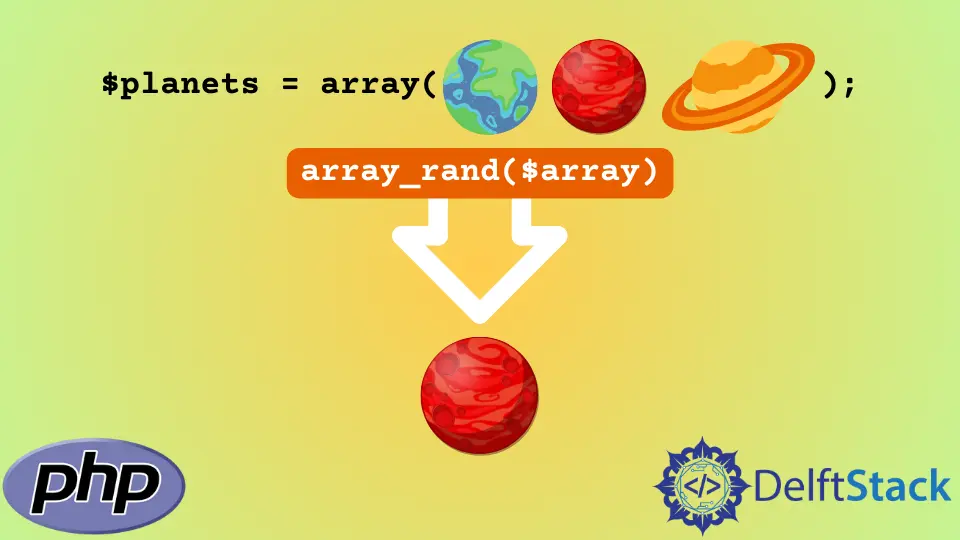
This tutorial introduces ways to get a random item from an array in PHP.
Use the array_rand()
Function to Get a Random Array Item in PHP
We can use the array_rand()
function to get the random item from an array in PHP. It uses the Mersenne Twister Random Number Generator for the randomization algorithm.
The function works both for the numeric arrays and the associative arrays. The array_rand()
function gives one or more random keys from an array.
To understand the function better, let’s hop into its syntax.
array_rand($array, $num)
We can specify an array as the function’s first parameter. The random item is taken from the array.
The parameter, $num
, is the number of random values to be returned. The default value of the $num
parameter is 1
.
If we specify values greater than 1
, the function will return multiple random keys in an array. Consequently, the array_rand()
function can return values in array, string or integer.
It returns a string value when the input array is an associative array that contains strings as the keys. For the numerically indexed array, the return value is an integer.
We can make the most of the array_rand()
function to find the random element from an array. Since the function returns a random key from an array, we can use the key as an index to find the random element.
For example, create two array variables, $array
and $age
. Create an indexed array in the $planets
variable and an associative array in the $age
variables.
Next, write a custom function find_random_item($array)
. Inside the function, use the array_rand()
function with $array
as its parameter.
Assign the function to the $rand_key
variable. Then, use the $rand_key
variable in the $array
and display the random element.
After closing the function body, invoke the find_random_item()
function once with the array $planets
and again with the array $age
as the arguments.
This is how we can find the random elements from an array using PHP’s array_rand()
function.
Example Code:
<?php
$planets = array("Mercury", "Venus", "Earth", "Mars", "Jupiter");
$age = array("Paul"=>"35", "Bob"=>"37", "Jack"=>"43");
function find_random_item($array){
$rand_key= array_rand($array);
$rand_element = $array[$rand_key];
echo $rand_element."<br>";
}
find_random_item($planets);
find_random_item($age);
Output:
Mars
37
Use the mt_rand()
Function to Get a Random Array Item in PHP
We can also use the mt_rand()
function to get a random array item. The function’s name is derived from the Mersenne Twister Random Number Generator.
The function returns a random integer. We can use that random integer as the index to get a random array element.
We need to specify the range of the random number to be returned as the parameters in the function. Let’s look at the syntax of the mt_rand()
function.
mt_rand($min, $max)
Here, $min
is the minimum value that could be generated, and $max
is the maximum value. For instance, for the function mt_rand(10,20)
, the random number can be 10 to 20.
The first step is to generate a random key for the array. We can choose 0
for the $min
option and the number one less than the array’s length for the $max
option.
As a result, a random key for the array will be generated. We can use the count()
function to generate the array’s length.
After finding the random key, we can find the random element.
For example, create an index array in the $countries
variable. Next, use the mt_rand()
function where 0
is the first parameter and count($countries)-1
is the second parameter.
Store the random key in a variable named $rand_key
. Next, use the $rand_key
as the index in the $countries
array to generate a random element from the array.
We can use the mt_rand()
function only for the indexed array for the generation of a random element.
Example Code:
$countries = array("Malaysia", "Vietnam", "Ecuador", "Malta", "Indonesia");
$rand_key = mt_rand(0, count($countries) - 1);
$rand_element = $countries[$rand_key];
echo $rand_element;
Output:
Ecuador
Use the shuffle()
Function to Get a Random Array Item in PHP
One of the simplest methods to get a random array element is the shuffle()
function. The function takes an array as the parameter and randomly shuffles the order of the elements in the array.
The function returns a Boolean value. As a result, a new array is formed.
Then, we can pick any one element from the array to get a random item.
For example, create an array in the variable $countries
. Use the array $countries
as the $shuffle
function parameter.
Next, use the echo
statement to pick the 0
th index of the $countries
array. As a result, a random array is picked.
We can use this method for both indexed and associative arrays.
Example Code:
$countries = array("Malaysia", "Vietnam", "Ecuador", "Malta", "Indonesia");
shuffle($countries);
echo $countries[0];
Output:
Malta
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedIn