How to Buffer Output Data in PHP With ob_start Method
-
Buffer Simple String Using the
ob_start
Method Then Get Data Using theob_get_contents
Method in PHP -
Buffer HTML Data Using the
ob_start
Method and Get Data Using theob_get_contents
Method in PHP -
Buffer String Data and Replace Chars in the String Using the
ob_start
Method With a Callback Function
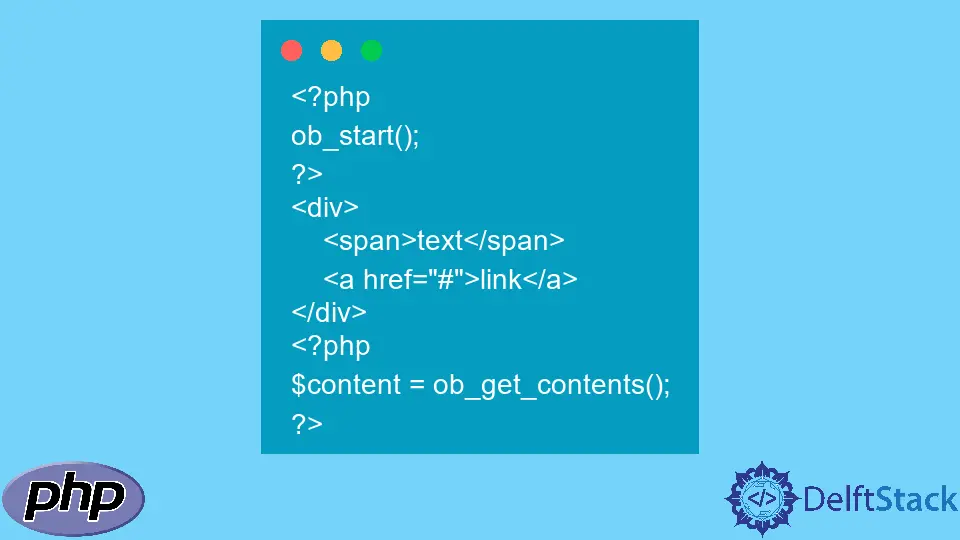
We will initialize a buffer with the ob_start
method and then output a simple string which will be automatically buffered; we will then get the data from the buffer with the ob_get_contents
method and then print it.
We will also initialize a buffer ob_start
method and then output an HTML block which will be automatically buffered; we will then get the data from the buffer with the ob_get_contents
method and then print it.
Lastly, we will initialize a buffer ob_start
method, declare a simple string that will be automatically buffered, and then replace data in the string using a call back passed to the ob_start
method.
Buffer Simple String Using the ob_start
Method Then Get Data Using the ob_get_contents
Method in PHP
We will set ob_start
and then output a simple string automatically buffered; we will then get the data from the buffer using ob_get_contents
and print it.
<?php
ob_start();
echo("Hello there!"); //would normally get printed to the screen/output to browser
$output = ob_get_contents();
echo $output;
?>
Output:
Hello there! Hello there!
Buffer HTML Data Using the ob_start
Method and Get Data Using the ob_get_contents
Method in PHP
We will set ob_start
and then output HTML data automatically buffered; we will then print the buffered data.
<?php
ob_start();
?>
<div>
<span>text</span>
<a href="#">link</a>
</div>
<?php
$content = ob_get_contents();
?>
Output:
<div>
<span>text</span>
<a href="#">link</a>
</div>
Buffer String Data and Replace Chars in the String Using the ob_start
Method With a Callback Function
We will set ob_start
and then output HTML data automatically buffered; we will then print the buffered data.
<?php
//Declare a string variable
$str = "I like PHP programming. ";
echo "The original string: $str";
//Define the callback function
function callback($buffer)
{
//Replace the word 'PHP' with 'Python'
return (str_replace("PHP", "Python", $buffer));
}
echo "The replaced string: ";
//call the ob_start() function with callback function
ob_start("callback");
echo $str;
?>
Output:
The original string: I like PHP programming. The replaced string: I like Python programming.