How to Parse a JSON File in PHP
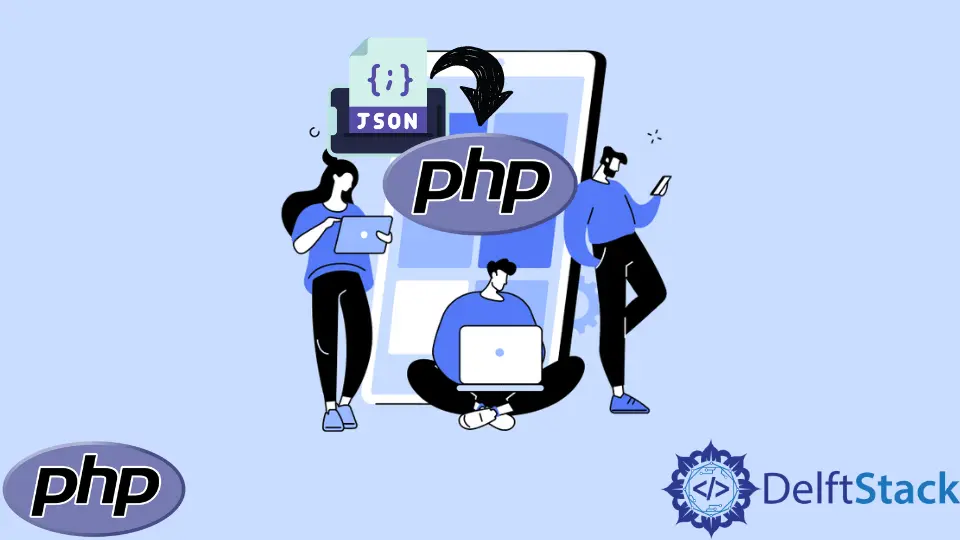
In this article, we will introduce the method to parse a JSON
file in PHP.
- Using
file_get_contents()
function
The contents of the JSON
file used in the example codes are as follows.
[
{
"id": "01",
"name": "Olivia Mason",
"designation": "System Architect"
},
{
"id": "02",
"name": "Jennifer Laurence",
"designation": "Senior Programmer"
},
{
"id": "03",
"name": "Medona Oliver",
"designation": "Office Manager"
}
]
Use file_get_contents()
Function to Parse a JSON File in PHP
The built-in function file_get_contents()
is used to read a file and store it into a string. By using this function, we can parse a JSON
file to a string. The correct syntax to use this function is as follows.
file_get_contents($pathOfFile, $searchPath, $customContext, $startingPoint, $length);
This function accepts five parameters. The detail of these parameters is as follows.
Parameter | Description | |
---|---|---|
$pathOfFile |
mandatory | It specifies the path of the file |
$searchPath |
optional | It specifies the path to search file. |
$customContext |
optional | It is used to specify a custom context. |
$startingPoint |
optional | It specifies the starting point of the reading file. |
$length |
optional | It is the maximum length of the file in bytes to be read. |
The following program shows how to parse a JSON
file.
<?php
$JsonParser = file_get_contents("myfile.json");
var_dump($JsonParser);
?>
The function file_get_contents()
have only parsed the JSON
data stored in a JSON
file. We cannot use this data directly.
Output:
string(328) "[
{
"id": "01",
"name": "Olivia Mason",
"designation": "System Architect"
},
{
"id": "02",
"name": "Jennifer Laurence",
"designation": "Senior Programmer"
},
{
"id": "03",
"name": "Medona Oliver",
"designation": "Office Manager"
}
]"
To make this data useful, we can use json_decode()
to convert the JSON
string to an array. Using this function in the below program.
<?php
$Json = file_get_contents("myfile.json");
// Converts to an array
$myarray = json_decode($Json, true);
var_dump($myarray); // prints array
?>
Output:
array(3) {
[0]=>
array(3) {
["id"]=>
string(2) "01"
["name"]=>
string(12) "Olivia Mason"
["designation"]=>
string(16) "System Architect"
}
[1]=>
array(3) {
["id"]=>
string(2) "02"
["name"]=>
string(17) "Jennifer Laurence"
["designation"]=>
string(17) "Senior Programmer"
}
[2]=>
array(3) {
["id"]=>
string(2) "03"
["name"]=>
string(13) "Medona Oliver"
["designation"]=>
string(14) "Office Manager"
}
}