How to Generate JSON File in PHP
- Understanding JSON and Its Importance
- Generating JSON File in PHP
- Handling Errors When Generating JSON Files
- Reading JSON Files in PHP
- Conclusion
- FAQ
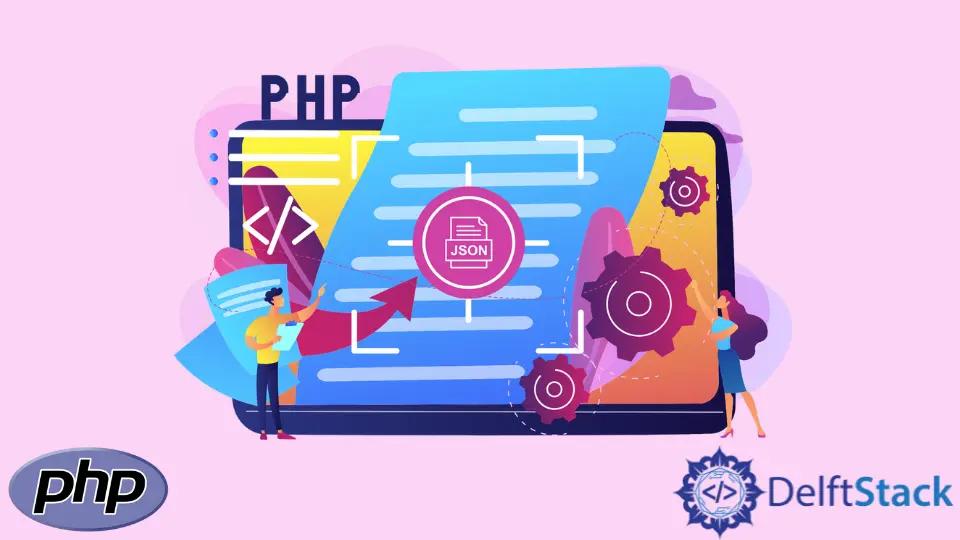
Creating a JSON file in PHP is a straightforward process that can be accomplished with a few lines of code. JSON (JavaScript Object Notation) is a lightweight data interchange format that is easy for humans to read and write and easy for machines to parse and generate. Whether you’re working on a web application or need to store data in a structured format, generating a JSON file can be incredibly useful.
In this article, we will explore how to generate a .json file in PHP using the file_put_contents()
function. By the end, you’ll have a solid understanding of how to create and manage JSON files in your PHP projects.
Understanding JSON and Its Importance
Before diving into the code, let’s take a moment to understand why JSON is so widely used. JSON is not only human-readable but also language-independent, making it a popular choice for data exchange between a server and a client. Its simplicity allows for quick parsing and serialization, which is essential for web applications that rely on data interchange. By generating JSON files in PHP, you can easily store and retrieve data, making your applications more dynamic and efficient.
Generating JSON File in PHP
To generate a JSON file in PHP, you can use the file_put_contents()
function, which writes data to a file. This function is straightforward and efficient for creating JSON files. Below is a simple example of how to create a JSON file in PHP.
<?php
$data = [
"name" => "John Doe",
"email" => "john.doe@example.com",
"age" => 30,
"is_active" => true
];
$jsonData = json_encode($data, JSON_PRETTY_PRINT);
file_put_contents('data.json', $jsonData);
?>
Output:
{
"name": "John Doe",
"email": "john.doe@example.com",
"age": 30,
"is_active": true
}
In this code snippet, we start by creating an associative array called $data
. This array contains various key-value pairs that we want to store in our JSON file. Next, we use the json_encode()
function to convert the array into a JSON string. The JSON_PRETTY_PRINT
option is used to format the JSON output for better readability. Finally, we call file_put_contents()
to write this JSON string to a file named data.json
. If the file does not exist, it will be created automatically.
Handling Errors When Generating JSON Files
When working with file operations, it’s essential to handle errors gracefully. You can enhance the previous example by adding error handling to ensure that your application behaves predictably even when something goes wrong. Here’s an updated version of the code that includes basic error handling.
<?php
$data = [
"name" => "Jane Doe",
"email" => "jane.doe@example.com",
"age" => 28,
"is_active" => true
];
$jsonData = json_encode($data, JSON_PRETTY_PRINT);
$result = file_put_contents('data.json', $jsonData);
if ($result === false) {
echo "Error writing to JSON file.";
} else {
echo "JSON file created successfully.";
}
?>
Output:
JSON file created successfully.
In this version, we check the result of file_put_contents()
. If it returns false
, we output an error message indicating that the file could not be written. If the operation is successful, we provide a success message. This approach ensures that your application can inform users of any issues during the file creation process.
Reading JSON Files in PHP
Once you’ve generated a JSON file, you might need to read it back into your PHP application. This is essential for retrieving data for display or processing. The following example demonstrates how to read a JSON file and decode it back into a PHP array.
<?php
$jsonData = file_get_contents('data.json');
$data = json_decode($jsonData, true);
print_r($data);
?>
Output:
Array
(
[name] => John Doe
[email] => john.doe@example.com
[age] => 30
[is_active] => 1
)
In this code snippet, we use file_get_contents()
to read the contents of data.json
. We then use json_decode()
to convert the JSON string back into a PHP array. By passing true
as the second argument to json_decode()
, we ensure that the result is an associative array rather than an object. Finally, we use print_r()
to display the contents of the array.
Conclusion
Generating JSON files in PHP is a valuable skill that can enhance your web applications. The file_put_contents()
function, combined with error handling and data retrieval techniques, allows you to create robust applications that can manage data efficiently. Whether you’re storing user information, configuration settings, or any other structured data, JSON offers a convenient and flexible format. Now that you understand how to create and manipulate JSON files in PHP, you can integrate this knowledge into your projects and improve your application’s data handling capabilities.
FAQ
-
How can I check if a JSON file exists in PHP?
You can use thefile_exists()
function to check if a JSON file exists before trying to read or write to it. -
Can I generate a JSON file from a database in PHP?
Yes, you can retrieve data from a database, convert it to an array, and then usejson_encode()
to create a JSON file. -
What happens if I try to write to a JSON file that is already open?
If you attempt to write to a file that is already open, it may lead to unexpected behavior. It’s best to ensure that the file is not opened elsewhere before writing. -
Is JSON format suitable for large datasets?
JSON is suitable for many applications, but for very large datasets, consider using more efficient formats like Protocol Buffers or Avro. -
How can I validate JSON data in PHP?
You can usejson_last_error()
after decoding JSON data to check for errors and validate its structure.