How to Convert List to NumPy Array in Python
-
Use the
numpy.array()
to Convert List to NumPy Array in Python -
Use the
numpy.asarray()
to Convert List to NumPy Array in Python
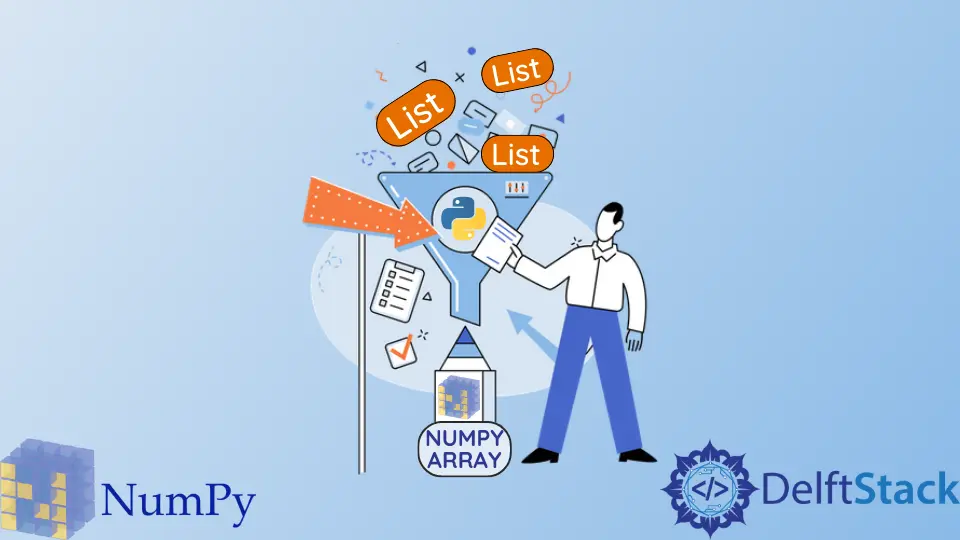
Lists and arrays are two of the most fundamental and frequently used collection objects in Python.
Both of them are mutable, used to store a collection of elements under a common name, and every element has a specific index that can be used to access it.
However, there are a few notable differences. Lists are already inbuilt in Python, whereas for arrays, we need to import arrays
or NumPy
module and need to declare arrays before using them. Arrays also store data more efficiently in memory and are highly used for mathematical operations.
In this tutorial, we will convert a list to a NumPy array.
Use the numpy.array()
to Convert List to NumPy Array in Python
The numpy.array
function is used to declare and create arrays in Python. In this function, we usually specify the elements in square brackets to pass the list directly. It works for a list of lists also. For example,
import numpy as np
l1 = [5, 7, 8]
arr = np.array(l1)
print(arr, arr.shape)
l2 = [[1, 5, 8], [18, 9, 2]]
arr_d = np.array(l2)
print(arr_d, arr_d.shape)
Output:
[5 7 8] (3,)
[[ 1 5 8]
[18 9 2]] (2, 3)
Use the numpy.asarray()
to Convert List to NumPy Array in Python
The numpy.asarray()
is used to convert objects of different types like dictionaries, lists, and more to numpy arrays. We will convert a list to a numpy array in the code below using the asarray()
function.
import numpy as np
l1 = [5, 7, 8]
arr = np.asarray(l1)
print(arr, arr.shape)
l2 = [[1, 5, 8], [18, 9, 2]]
arr_d = np.asarray(l2)
print(arr_d, arr_d.shape)
Output:
[5 7 8] (3,)
[[ 1 5 8]
[18 9 2]] (2, 3)
Note that both the methods mentioned above also work in converting a list of lists to a numpy array.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn