How to Process Form Data With Mysqli_real_escape_string
- Set Up a Local Server
- Create a Database and a Table
- Create the HTML Form
- Process Form Data
-
Causes of an Error in
Mysqli_real_escape_string
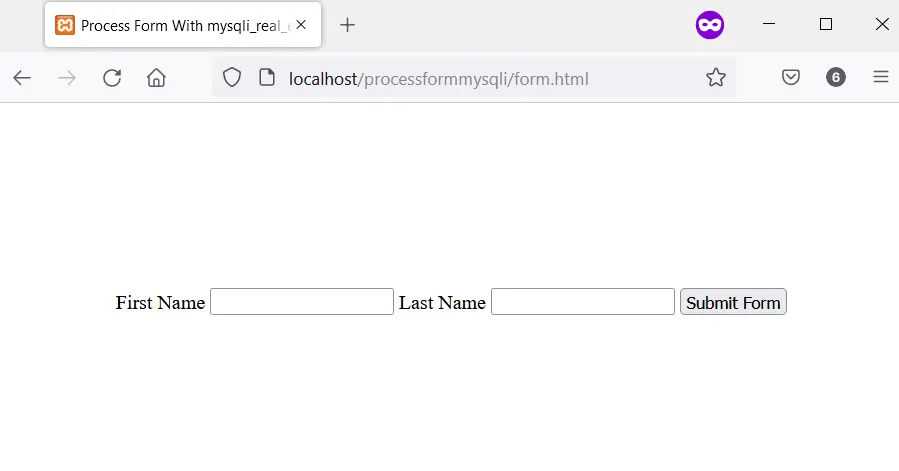
This article will teach you to process form data using mysqli_real_escape_string
.
First, we’ll set up a sample database and a table. Then we’ll create an HTML form that’ll accept user input.
Afterward, in PHP, we’ll explain how to use mysqli_real_escape_string
without causing an error.
Set Up a Local Server
All the code for this article will work on a server. So, if you have access to a live server, you can skip this section and move on to the next one.
If not, install a local server like XAMPP from Apache Friends. Once XAMPP is installed, locate the htdocs
folder and create a folder.
This folder will store all the code for this article.
Create a Database and a Table
In XAMPP, you can create a database using phpMyAdmin
or the command line. If you are on the command line, log in to MySQL using the following command:
#login to mysql
mysql -u root -p
When you’ve logged into MySQL, create a database. For this article, we’ll call the database my_details
.
CREATE database my_details
With the database created, create a table with the next SQL code. The table will hold the data for our sample project.
CREATE TABLE bio_data (
id INT NOT NULL AUTO_INCREMENT,
first_name VARCHAR(50) NOT NULL,
last_name VARCHAR(50) NOT NULL,
PRIMARY KEY (id)) ENGINE = InnoDB;
Create the HTML Form
The HTML form will have two form inputs. The first collects the user’s first name, while the second is for the last name.
<head>
<meta charset="utf-8">
<title>Process Form With mysqli_real_escape_string</title>
<style>
body {
display: grid;
justify-content: center;
align-items: center;
height: 100vh;
}
</style>
</head>
<body>
<main>
<form action="process_form.php" method="post">
<label id="first_name">First Name</label>
<input id="first_name" type="text" name="first_name" required>
<label id="last_name">Last Name</label>
<input id="last_name" type="text" name="last_name" required>
<input type="submit" name="submit_form" value="Submit Form">
</form>
</main>
</body>
Output:
Process Form Data
During the form processing, we use mysqli_real_escape_string
to escape the form inputs. What’s more, the database connection should be the first argument of mysqli_real_escape_string
.
So, we’ve used mysqli_real_escape_string
on the first and last name in the following. To use the code, save it as process_form.php
.
<?php
if (isset($_POST['submit_form']) && isset($_POST["first_name"]) && isset($_POST["last_name"])) {
// Set up a database connection.
// Here, our password is empty
$connection_string = new mysqli("localhost", "root", "", "my_details");
// Escape the first name and last name using
// mysqli_real_escape_string function. Meanwhile,
// the first parameter to the function should
// be the database connection. If you omit, the
// database connection, you'll get an error.
$first_name = mysqli_real_escape_string($connection_string, trim(htmlentities($_POST['first_name'])));
$last_name = mysqli_real_escape_string($connection_string, trim(htmlentities($_POST['last_name'])));
// If there is a connection error, notify
// the user, and Kill the script.
if ($connection_string->connect_error) {
echo "Failed to connect to Database. Please, check your connection details.";
exit();
}
// Check string length, empty strings and
// non-alphanumeric characters.
if ( $first_name === "" || !ctype_alnum($first_name) ||
strlen($first_name) <= 3
) {
echo "Your first name is invalid.";
exit();
}
if ( $last_name === "" || !ctype_alnum($last_name) ||
strlen($last_name) < 2
) {
echo "Your last name is invalid.";
exit();
}
// Insert the record into the database
$query = "INSERT into bio_data (first_name, last_name) VALUES ('$first_name', '$last_name')";
$stmt = $connection_string->prepare($query);
$stmt->execute();
if ($stmt->affected_rows === 1) {
echo "Data inserted successfully";
}
} else {
// User manipulated the HTML form or accessed
// the script directly. Kill the script.
echo "An unexpected error occurred. Please, try again later.";
exit();
}
?>
Output (if processing was successful):
Causes of an Error in Mysqli_real_escape_string
You’ll get an error if you omit the database connection in mysqli_real_escape_string
. So, in the following code, we’ve modified process_form.php
.
Meanwhile, this version does not have the database connection in mysqli_real_escape_string
. As a result, you’ll get an error when you want to insert data into the database.
<?php
if (isset($_POST['submit_form']) && isset($_POST["first_name"]) && isset($_POST["last_name"])) {
$connection_string = new mysqli("localhost", "root", "", "my_details");
// We've omitted the connection string
$first_name = mysqli_real_escape_string(trim(htmlentities($_POST['first_name'])));
$last_name = mysqli_real_escape_string(trim(htmlentities($_POST['last_name'])));
if ($connection_string->connect_error) {
echo "Failed to connect to Database. Please, check your connection details.";
exit();
}
if ( $first_name === "" || !ctype_alnum($first_name) ||
strlen($first_name) <= 3
) {
echo "Your first name is invalid.";
exit();
}
if ( $last_name === "" || !ctype_alnum($last_name) ||
strlen($last_name) < 2
) {
echo "Your last name is invalid.";
exit();
}
$query = "INSERT into bio_data (first_name, last_name) VALUES ('$first_name', '$last_name')";
$stmt = $connection_string->prepare($query);
$stmt->execute();
if ($stmt->affected_rows === 1) {
echo "Data inserted successfully";
}
} else {
echo "An unexpected error occurred. Please, try again later.";
exit();
}
?>
Sample error message:
Fatal error: Uncaught ArgumentCountError: mysqli_real_escape_string() expects exactly 2 arguments, 1 given in C:\xampp\htdocs\processformmysqli\process_form.php:12 Stack trace: #0 C:\xampp\htdocs\processformmysqli\process_form.php(12): mysqli_real_escape_string('Johnson') #1 {main} thrown in C:\xampp\htdocs\processformmysqli\process_form.php on line 12
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn