How to Copy a Collection Within the Same Database in MongoDB
- Copy a Collection Within the Same Database While Working in MongoDB
-
Use
mongodump
andmongorestore
to Copy a Collection Within the Same Database in MongoDB -
Use the
aggregate()
Method to Copy a Collection Within the Same Database in MongoDB -
Use the
forEach()
Loop to Copy a Collection Within the Same Database in MongoDB
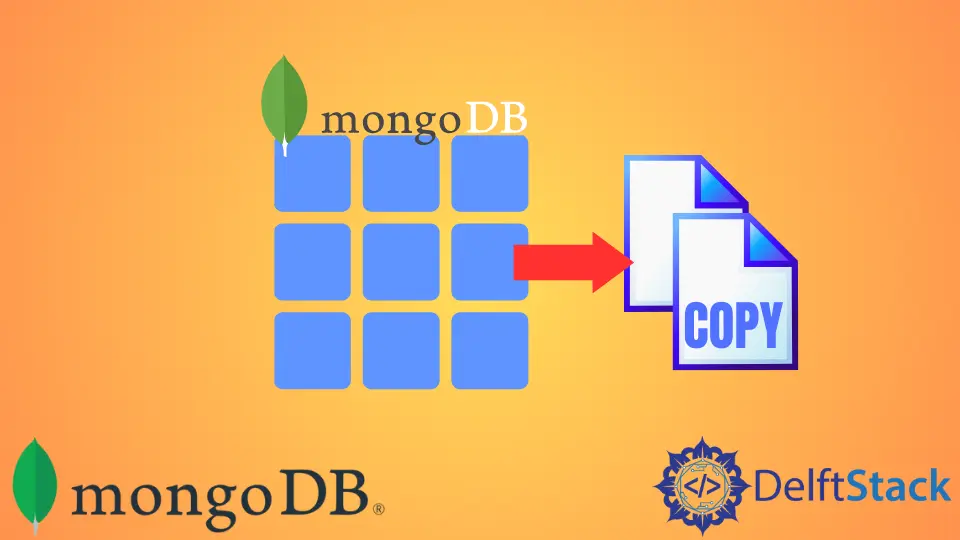
Copying a collection within the same database is an amazing task; it saves time and effort. This tutorial demonstrates using mongodump
, mongorestore
, aggregate()
, and forEach()
to copy a collection within the same database using MongoDB.
Copy a Collection Within the Same Database While Working in MongoDB
In the old versions of MongoDB, for instance less than 3.0, we could use the copyTo()
method as db.collection_name.copyTo()
to copy the collection but that is deprecated now.
The eval
is also deprecated with the start of MongoDB Version 4.2. Please note that the db.collection_name.copyTo()
wraps the eval
, which means we cannot copy the collection by using either of them if we have MongoDB version 4.2 or higher.
There are a few other ways in new versions of MongoDB that we can use to copy a collection within the same database. A few of them are given here.
- Use the
mongodump
andmongorestore
commands - Use the
aggregate()
method - Use
forEach()
loop
Let’s start learning each of them one by one.
Use mongodump
and mongorestore
to Copy a Collection Within the Same Database in MongoDB
It is the fastest way to clone/copy a collection within the same database using MongoDB Database Tools; particularly, we can use the mongodump
and mongorestore
. The database tool is a suite of command-line utilities for working in MongoDB.
We can use the following commands on the Windows command prompt to check the version of mongodump
and mongorestore
. If it returns the respective version successfully, the database tools are installed.
Otherwise, follow this to install the database tools.
Example Code:
C:/Users/Dell> mongodump --version
Example Code:
C:/Users/Dell> mongorestore --version
Remember, we have to execute the mongodump
and mongorestore
commands from the command line of our system, for instance, the Command Prompt from Windows OS or terminal if we are using Ubuntu. Never run this command from the mongo shell.
Once we have the database tools for working with MongoDB, run the following command to dump the teachers
collection within the same database: test
.
Example Code:
C:/Users/Dell> mongodump -d test -c teachers
OUTPUT:
2022-05-27T13:05:14.497+0500 writing test.teachers to dump\test\teachers.bson
2022-05-27T13:05:14.503+0500 done dumping test.teachers (3 documents)
The above output shows that the dump file is written at dump\test\teachers.bson
. So, we need to restore it using the command given below.
Example Code:
C:/Users/Dell>mongorestore -d test -c teachers1 --dir=dump/<db>/<sourcecollection.bson>
OUTPUT:
2022-05-27T13:05:28.085+0500 checking for collection data in dump\test\teachers.bson
2022-05-27T13:05:28.088+0500 reading metadata for test.teachers1 from dump\test\teachers.metadata.json
2022-05-27T13:05:28.252+0500 restoring test.teachers1 from dump\test\teachers.bson
2022-05-27T13:05:28.312+0500 finished restoring test.teachers1 (3 documents, 0 failures)
2022-05-27T13:05:28.312+0500 no indexes to restore for collection test.teachers1
2022-05-27T13:05:28.313+0500 3 document(s) restored successfully. 0 document(s) failed to restore.
The output will look like the above, which means the collection is successfully copied. The target collection named teachers1
will be created if it does not exist in the current database.
Next, open the mongo shell and execute the following query to see if the copied collection is there.
Example Code:
> show collections
OUTPUT:
teachers
teachers1
Alternatively, we can also use mongoexport
to export the collection (teachers
) from a database (test
) and then use mongoimport
to import it into the teachers2
collection within the same database.
Example Code:
C:/Users/Dell> mongoexport -d test -c teachers | mongoimport -d test -c teachers2 --drop
Use the aggregate()
Method to Copy a Collection Within the Same Database in MongoDB
Example Code:
> db.teachers.aggregate([{$out: "teachers3"}])
This command is executed using the mongo shell. After that, we use the show collections
command to see if teachers3
is there.
Example Code:
> show collections
OUTPUT:
teachers
teachers1
teachers2
teachers3
We are using the aggregation pipeline that returns the data from the teachers
collection and writes them into the specified collection, which is teachers3
here. We can use this approach if we have MongoDB version 4.4 or above.
Use the forEach()
Loop to Copy a Collection Within the Same Database in MongoDB
Example Code:
> db.teachers.find().forEach((doc) => {
db.teachers4.insert(doc)
})
Use the following query to confirm that teachers4
resides within the same database.
Example Code:
> show collections
OUTPUT:
teachers
teachers1
teachers2
teachers3
teachers4
This method is the slowest compared to all of the above approaches because of using a loop. It iterates over all the documents of the source collection and inserts them into the target collection one by one.