How to Split String in Bash
-
Using the
tr
Command to Split a String in Bash -
Using
IFS
to Split a String in Bash -
Using the
read
Command to Split a String in Bash - Using Parameter Expansion to Split a String in Bash
-
Using the
cut
Command to Split a String in Bash
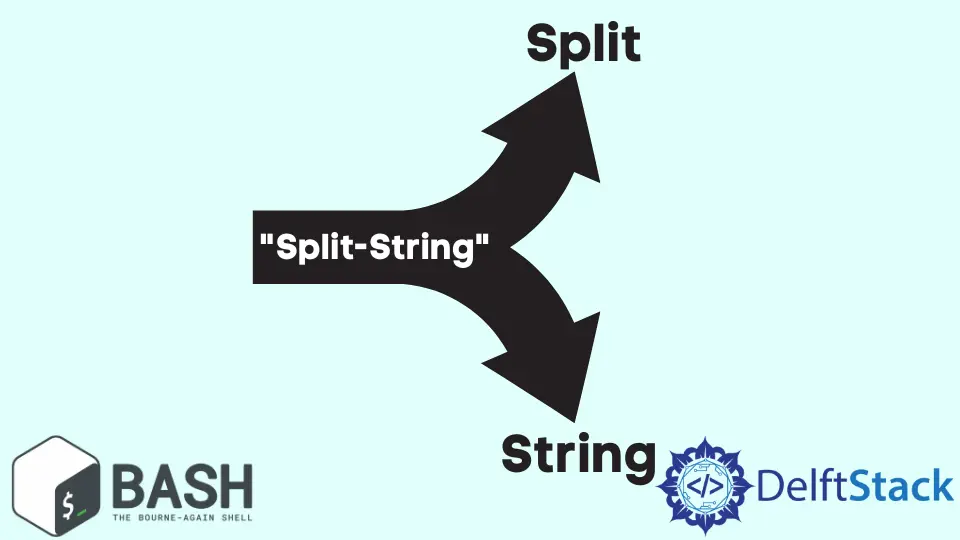
This tutorial demonstrates splitting a string on a delimiter in bash using the tr
command, the IFS
, the read
command, parameter expansion, and the cut
command.
Using the tr
Command to Split a String in Bash
The tr
command is a short form for translate
. It translates, deletes, and squeezes characters from the standard input and writes the results to the standard output.
It is a useful command for manipulating text on the command line or in a bash script. It can remove repeated characters, convert lowercase to uppercase, and replace characters.
In the bash script below, the echo
command pipes the string variable, $addrs
, to the tr
command, which splits the string variable on a delimiter, -
. Once the string has been split, the values are assigned to the IP
variable.
Then, the for
loop loops through the $IP
variable and prints out all the values using the echo
command.
#!/usr/bin/env bash
addrs="192.168.8.1-192.168.8.2-192.168.8.3-192.168.8.4"
IP=$(echo $addrs | tr "-" "\n")
for ip in $IP
do
echo "$ip"
done
The output below shows that the $addr
string variable has been split on the delimiter, -
, into 4 separate strings.
192.168.8.1
192.168.8.2
192.168.8.3
192.168.8.4
Using IFS
to Split a String in Bash
IFS
stands for Internal Field Separator.
The IFS
is used for word splitting after expansion and to split lines into words with the built-in read
command. The value of IFS
tells the shell how to recognize word boundaries.
The default value of the IFS
is a space, a tab, and a new line. In the script below, the original value of the IFS
has been stored in the OIFS
variable, and the new IFS
value has been set to -
.
This means that the shell should treat -
, as the new word boundary. The shell splits the string variable addrs
on -
and assigns the new values to the ips
variable.
Then, the for
loop loops through the $ips
variable and prints out all the values using the echo
command.
IFS=$OIFS
is used to restore the original value of the IFS
variable, and the unset OIFS
, tells the shell to remove the variable OIFS
from the list of variables that it keeps track of.
#!/usr/bin/env bash
addrs="192.168.8.1-192.168.8.2-192.168.8.3-192.168.8.4"
OIFS=$IFS
IFS='-'
ips=$addrs
for ip in $ips
do
echo "$ip"
done
IFS=$OIFS
unset OIFS
The output below shows that the $addr
string variable has been split on the delimiter, -
, into 4 separate strings.
192.168.8.1
192.168.8.2
192.168.8.3
192.168.8.4
Using the read
Command to Split a String in Bash
The read
command is a built-in command on Linux systems.
It is used to read the content of a line into a variable. It also splits words of a string that is assigned to a shell variable.
The variable $addrs
string is passed to the read
command in the script below. The IFS
sets the delimiter that acts as a word boundary on the string variable.
This means the -
is the word boundary in our case. The -a
option tells the read
command to store the words that have been split into an array, while the -r
option tells the read
command to treat any escape characters as they are and not interpret them.
The words that have been split are stored into the IP
array. The for
loop loops through the $IP
array and prints out all the values using the echo
command.
#!/usr/bin/env bash
addrs="192.168.8.1-192.168.8.2-192.168.8.3-192.168.8.4"
IFS='-' read -ra IP <<< "$addrs"
for ip in "${IP[@]}";
do
echo "$ip"
done
From the output below, we can observe that the $addr
string variable has been split on the delimiter, -
, into separate 4 strings.
192.168.8.1
192.168.8.2
192.168.8.3
192.168.8.4
Using Parameter Expansion to Split a String in Bash
The script below uses parameter expansion to search and replace characters. The syntax used for for parameter expansion is ${variable//search/replace}
. This searches for a pattern that matches search
in the variable
and replaces it with the replace
.
In our case, the script searches for the pattern -
and replaces it with white space. The parenthesis around ${addrs//-/ }
are used to define an array of the new string, called ip_array
.
We use the for
loop to iterate over all the ip_array
variable elements and display them using the echo
command.
#!/usr/bin/env bash
addrs="192.168.8.1-192.168.8.2-192.168.8.3-192.168.8.4"
ip_array=(${addrs//-/ })
for ip in "${ip_array[@]}"
do
echo "$ip"
done
The output below shows all the elements in the ip_array
.
192.168.8.1
192.168.8.2
192.168.8.3
192.168.8.4
We can access individual elements of the ip_array
variable by passing in the index. In the script below, we have passed the index 0
to refer to the first element in the array.
#!/usr/bin/env bash
addrs="192.168.8.1-192.168.8.2-192.168.8.3-192.168.8.4"
ip_array=(${addrs//-/ })
printf "${ip_array[0]}\n"
The output shows the first element of the ip_array
.
192.168.8.1
Using the cut
Command to Split a String in Bash
The script below uses the cut
command to extract a substring. The -d
option specifies the delimiter to use to divide the string into fields and the -f
option sets the number of the field to extract.
But the string is divided using -
as the delimiter, in our case, and to access the first field, we pass the argument 1
to the -f
option, and we do the same to access the second field by passing 2
to the -f
option.
The values are assigned to the ip_one
and ip_two
variables, respectively. The printf
command is used to display the values of the variables.
#!/usr/bin/env bash
addrs="192.168.8.1-192.168.8.2-192.168.8.3-192.168.8.4"
ip_one=$(echo $addrs | cut -d '-' -f 1)
ip_two=$(echo $addrs | cut -d '-' -f 2)
printf "$ip_one\n$ip_two\n"
The output below displays the first and second strings split from the $addrs
string variable.
192.168.8.1
192.168.8.2