jQuery Confirm Plugin
-
Use of jQuery
.$alert
to Ensure Confirmation -
Use of jQuery
$.dialog
to Ensure Confirmation -
Use of jQuery
$.confirm
to Verify Confirmation
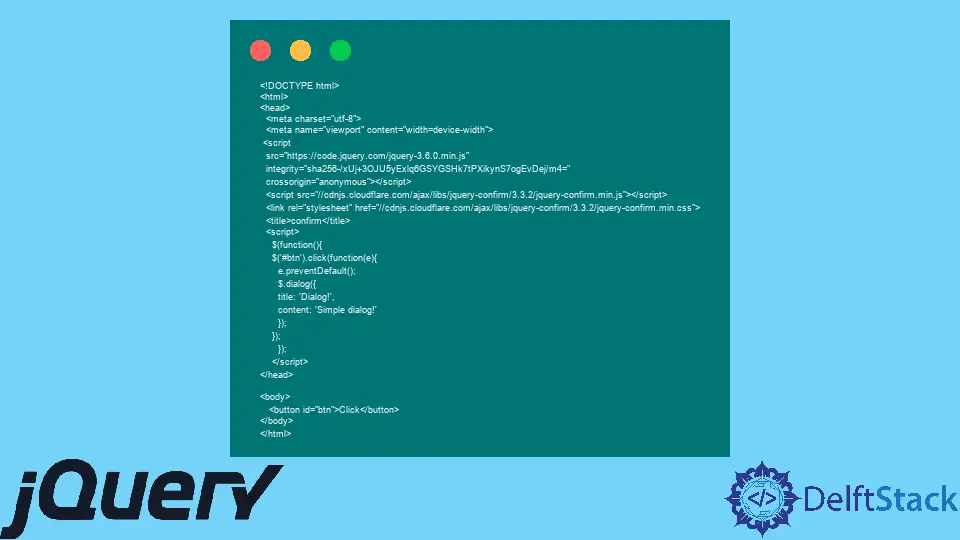
In jQuery, the confirm
plug-in comprises a function similar to the JavaScript confirm
function. But the basic distinctive characteristic is that the jQuery $.confirm
has some properties (including OK
and Cancel
buttons) which can be altered as a preference, while the JavaScript confirm
is rigid, and the minimal buttons cannot be changed.
So, in other words, jQuery does get the spotlight for being more flexible. The task of confirming can be implemented by JavaScript, jQuery confirm
plug-in and jQuery dialog
UI.
We will only focus on the solution based on the jQuery confirm
plug-in.
To enable the plug-in on your local PC, you can depend on the CDNs, or you might install the package via npm
. This portal can be a proper user guide for you.
In our example sets, we will use the CDNs for versatile use cases and examination.
Use of jQuery .$alert
to Ensure Confirmation
According to basic differentiation between alert
, dialog
, and confirm
actions, the alert
comes with one single button, OK
. In contrast, the dialog
previews a basic modal with customized content and title values.
So, let’s visualize the alert
type confirmation.
Code Snippet:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<script
src="https://code.jquery.com/jquery-3.6.0.min.js"
integrity="sha256-/xUj+3OJU5yExlq6GSYGSHk7tPXikynS7ogEvDej/m4="
crossorigin="anonymous"></script>
<script src="//cdnjs.cloudflare.com/ajax/libs/jquery-confirm/3.3.2/jquery-confirm.min.js"></script>
<link rel="stylesheet" href="//cdnjs.cloudflare.com/ajax/libs/jquery-confirm/3.3.2/jquery-confirm.min.css">
<title>confirm</title>
<script>
$(function(){
$('#btn').click(function(e){
e.preventDefault();
$.alert({
title: 'Alert!',
content: 'Simple alert!'
});
});
});
</script>
</head>
<body>
<button id="btn">Click</button>
</body>
</html>
Output:
Use of jQuery $.dialog
to Ensure Confirmation
We will see the basic template of the dialog
action and alter the title and content of it. As dialog
does not have any buttons, all it does is show a message of preference.
It has a cross (x
) button in the upper-right corner to return to the main contents.
Code Snippet:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<script
src="https://code.jquery.com/jquery-3.6.0.min.js"
integrity="sha256-/xUj+3OJU5yExlq6GSYGSHk7tPXikynS7ogEvDej/m4="
crossorigin="anonymous"></script>
<script src="//cdnjs.cloudflare.com/ajax/libs/jquery-confirm/3.3.2/jquery-confirm.min.js"></script>
<link rel="stylesheet" href="//cdnjs.cloudflare.com/ajax/libs/jquery-confirm/3.3.2/jquery-confirm.min.css">
<title>confirm</title>
<script>
$(function(){
$('#btn').click(function(e){
e.preventDefault();
$.dialog({
title: 'Dialog!',
content: 'Simple dialog!'
});
});
});
</script>
</head>
<body>
<button id="btn">Click</button>
</body>
</html>
Output:
Use of jQuery $.confirm
to Verify Confirmation
In this regard, we will have two button options. By default, for every confirm
action, the texts are OK
and Cancel
.
However, here we have the privilege to alter these texts. Also, some other parameters can be manipulated.
The link in the introduction can let you through the latest list. Let’s check our example for a better view of the task.
Code Snippet:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<script
src="https://code.jquery.com/jquery-3.6.0.min.js"
integrity="sha256-/xUj+3OJU5yExlq6GSYGSHk7tPXikynS7ogEvDej/m4="
crossorigin="anonymous"></script>
<script src="//cdnjs.cloudflare.com/ajax/libs/jquery-confirm/3.3.2/jquery-confirm.min.js"></script>
<link rel="stylesheet" href="//cdnjs.cloudflare.com/ajax/libs/jquery-confirm/3.3.2/jquery-confirm.min.css">
<title>confirm</title>
<script>
$(function(){
var ask = true;
$('#btn').click(function(e){
if(ask){
e.preventDefault();
$.confirm({
title: "Delete",
content: "You sure?",
buttons: {
confirm: {
text: "Yes",
action: function(){
ask = false;
alert("Deleted.");
}
},
cancel: {
text: "No"
}
}
});
}
});
});
</script>
</head>
<body>
<button id="btn">Click</button>
</body>
</html>
Output:
One fact to focus is, all the functions $.alert()
, $.confirm()
, and $.dialog()
are part of the jconfirm()
function. All of them are interchangeably used based on the use case.
Also, we have used the CDNs for jQuery minified js
, jquery-confirm
minified js
, and jquery-confirm
minified css
. Make sure to check on the versions and later updates while taking the examples as references.