JavaScript Subscription Method
-
Use the
.subscribe()
Method in JavaScript -
Use the
unsubscribe()
Method in JavaScript -
the
subscribe()
Plugin in jQuery
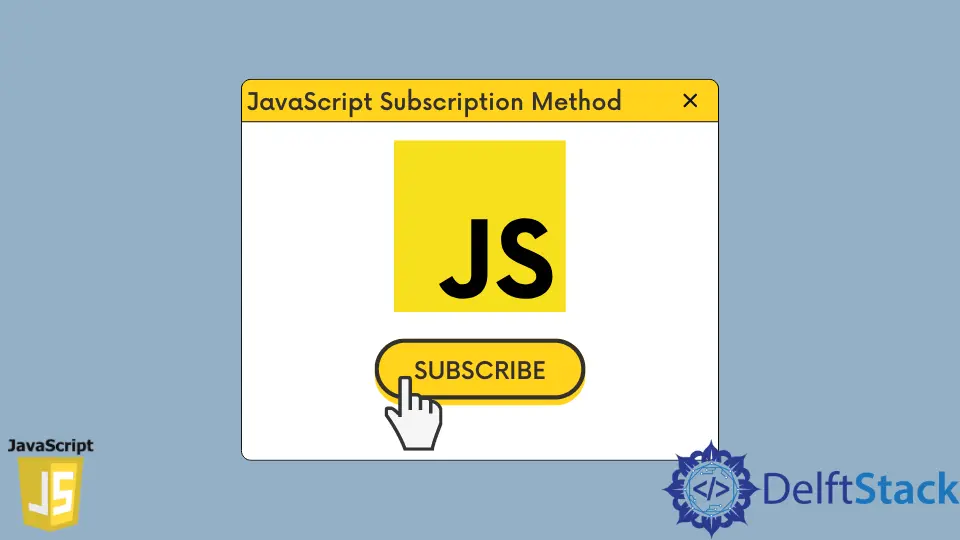
We will learn the purpose of the subscription functionality and its usage in JavaScript or jQuery. We will see how to create and execute the subscription using the subscribe()
method in our JavaScript code statements with different examples.
Use the .subscribe()
Method in JavaScript
The .subscribe()
method is alike to the Promise .then()
, .catch()
, and .finally()
methods of jQuery, but rather dealing with promises it deals with Observables.
If we are looking to execute and run some logic on fail case (like to .catch()
) or on success case (like to .finally()
) we can pass that logic to the subscribe as shown below:
myObservable.subscribe(
value => toDoOnlyOnSuccess(value), error => toDoOnlyOnError(error),
() => toDoAnyCase());
To represent a disposable resource, we use a subscription which is an object. It is the execution of an observable.
An essential subscription method is unsubscribe()
, which takes no argument and throws away the resource detained by the subscription. The subscription was called Disposable
in previous versions of RxJs, a reactive extension for JavaScript.
import {interval} from 'rxjs';
const myObservable = interval(1000);
const mySubscription = myObservable.subscribe(x => console.log(x));
// ongoing Observable execution is canceled
// which was started by calling subscribe with an Observer.
mySubscription.unsubscribe();
Use the unsubscribe()
Method in JavaScript
We have the unsubscribe()
function in Subscription to release resources or cancel ongoing observable execution. We can also put subscriptions together to call an unsubscribe()
method for multiple subscriptions.
We can achieve this by adding one subscription into another, as shown below:
import {interval} from 'rxjs';
const observableA = interval(500);
const observableB = interval(400);
const subscriptionMain =
observableA.subscribe(x => console.log('first subscription: ' + x));
const SubscriptionChild =
observableB.subscribe(x => console.log('second subscription: ' + x));
mySubscription.add(SubscriptionChild);
setTimeout(() => {
// Unsubscribes subscriptionMain and SubscriptionChild together
mySubscription.unsubscribe();
}, 1000);
Output:
second subscription: 0
first subscription: 0
second subscription: 1
first subscription: 1
second subscription: 2
the subscribe()
Plugin in jQuery
A jQuery plugin subscribe()
that is used as an event aggregator,the name of event can be defined as .subscribe("HelloWorld",function(){})
. It enables developer to write out code with low coupling in JavaScript/jQuery.
We need to specify event handle to event name as shown below:
`.subscribe("HelloWorld", function(data) {alert("Hello World!");});`
The subscribe()
method subscribes a specific event name with an event handler, and the handler will be executed when the topic is subscribed.