How to Remove Non Alphanumeric Characters Using JavaScript
- Remove Non-Alphanumeric Characters Using JavaScript
-
Use the
JSON.stringify()
to Convert Strings in JavaScript -
Use the
\u0600-\u06FF
to Remove Except Alphanumeric in JavaScript
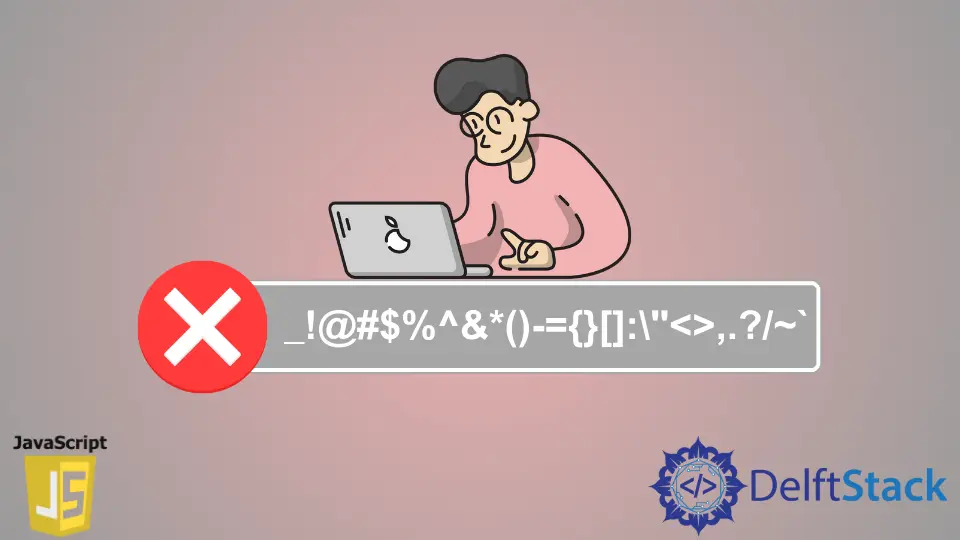
Non-alphanumeric means everything except alphabets and numbers. The alphabet can be of any language.
This tutorial elaborates on how we can remove non-alphanumeric characters using JavaScript. We can use the replace()
method in two ways.
First as str.replace()
and second as JSON.stringify(obj).replace()
. The replace()
function searches for a value or a pattern (also known as a regular expression) in the given string, replace it and outputs a brand new string without modifying the original string.
Remember, it is compulsory to use regular expressions with modifiers if we want to replace multiple instances in a string.
The JSON.stringify()
converts an object to a string, and the resulting string follows the JSON notation. This method is very useful if we want to replace non-alphanumeric characters in all elements of an array.
Remove Non-Alphanumeric Characters Using JavaScript
Here, we discard everything except English alphabets (Capital and small) and numbers. The g
modifier says global, and i
matches case-insensitivity.
var input = '123abcABC-_*(!@#$%^&*()_-={}[]:\"<>,.?/~`';
var stripped_string = input.replace(/[^a-z0-9]/gi, '');
console.log(stripped_string);
Output:
"123abcABC"
We can optimize the regex used in the above code by replacing it with /[\W]/g
where \W
is equivalent to [^0-9a-zA-Z]
. So, our final regex would be /[\W_]/g
because we remove underscore
from the input
string.
Check the code given below.
var input = '123abcABC-_*(!@#$%^&*()_-={}[]:\"<>,.?/~`';
var stripped_string = input.replace(/[\W_]/g, '')
console.log(stripped_string);
Output:
"123abcABC"
Use the JSON.stringify()
to Convert Strings in JavaScript
Now suppose that we have an array that contains multiple strings as elements. Here, we can use JSON.stringify()
to convert them into strings and replace them depending on the provided regex.
var input = ['123abcABC-_*(!@#$%^&*', 'ABC()_-={}[]:\"<>,.?/~`'];
var stripped_string = JSON.stringify(input).replace(/[\W_]/g, '')
console.log(stripped_string);
Output:
"123abcABCABC"
Use the \u0600-\u06FF
to Remove Except Alphanumeric in JavaScript
We want to remove everything except alphanumeric, but we have Arabic and English alphabets this time. To identify Arabic letters, we have to use the Unicode’s relative block range, \u0600-\u06FF
.
var input = 'ن$%^&*(ص ع___ربي-abc123_*(!@#$%^&*()_-={}[]:\"<>,.?/~`';
var stripped_string = input.replace(/[^0-9a-z\u0600-\u06FF]/gi, '')
console.log(stripped_string);
Output:
"نصعربيabc123"
Think a bit more and imagine working with multiple languages and searching for relative block range from Unicode for each language. That would be time-consuming, right?
In this situation, /[^\p{L}\d]/gu
is very useful. Here, \p{L}
finds letters from any language and \d
looks for numbers.
The g (global)
replaces globally while Unicode escape sequences are identified using u (Unicode)
.
Here, the ^
symbol is used to negate the given character set. So, the final regex /[^\p{L}\d]/gu
means replacing everything that is negating the provided character set.
function nonAlphaNumericRemoval(input) {
return input.replace(/[^\p{L}\d]/gu, '')
}
input_string = [
'#$asdé5kfjdk?',
'%^uQjoFß^ßI$jI',
'*(无论3如何?!',
'[фв@#ео1]'
]
for (var input of input_string) {
console.log(nonAlphaNumericRemoval(input))
}
Output:
"asdé5kfjdk"
"uQjoFßßIjI"
"无论3如何"
"фвео1"