Phone Number RegEx in JavaScript
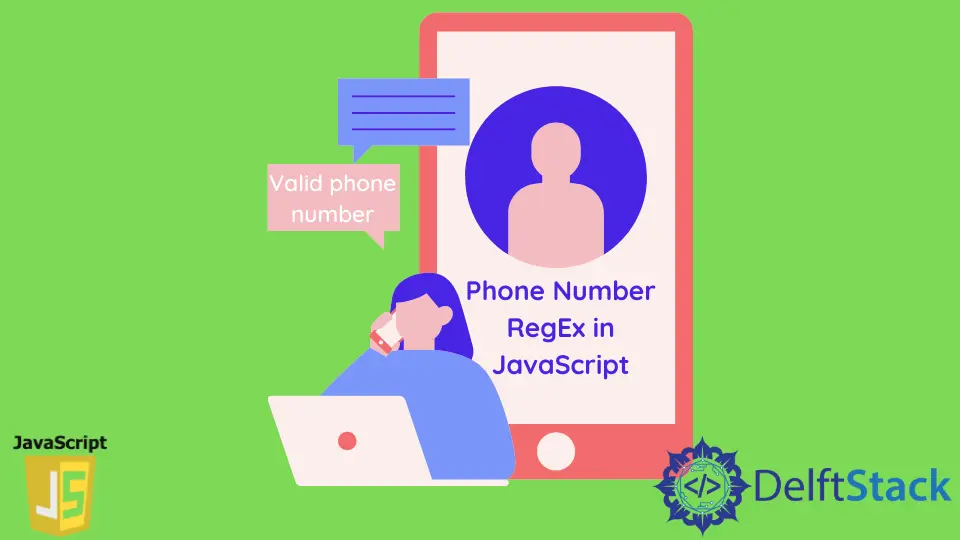
Each country has its own number format, and before we store this data in the database, we need to make sure that the user is entering the correct number format. In this post, we’re going to learn how to write regular expressions for phone numbers in JavaScript.
RegEx
in JavaScript
Regular expressions are like a search tool that can find specific patterns in strings. A good resource to learn RegEx is https://regexr.com. JavaScript also supports regular expressions as an object. These patterns are used with the matchAll()
, match()
, replaceAll()
, replace()
, search()
, and split()
. There are two ways to construct the regular expression, use a literal regular expression and call the constructor function of the RegExp object.
Let’s understand some of the common patterns before we move on to the phone number RegEx.
\d
: This regular expression matches any number/digit. It is similar to[0-9]
.\w
: This regular expression matches any given word character code in the range ofA
-Z
,a
-z
,0
-9
and_
.\s
: This regular expression matches every whitespace character, such as spaces, tabs, line breaks.\b
: This regular expression matches any word boundary position between a word character and a non-word character or a position (beginning/end of the character string).[A-Z]
: This regular expression matches any given character code in the range fromA
-Z
.[0-9]
: This regular expression matches any given character code in the range from0
-9
.(?:ABC)
: This regular expression groups multiple tokens together without creating a capturing group.
Each RegEx contains certain tags listed below:
i
: This flag means that it is case insensitive, which means that the entire expression is not case sensitive.g
: This flag means a global search that maintains the index of the last match so that subsequent searches can start at the end of the previous match. Without the global flag, subsequent searches will return the same match.m
: This flag means multi-line. When the multi-line flag is on, the start and end anchors (^
and$
) match the beginning and end of a line, not the beginning and end of the entire chain.u
: This flag means Unicode. Users can use extended Unicode escapes of the form\ x {FFFFF}
by activating this flag.y
: This flag means sticky. The expression only matches its lastIndex position and ignores the global flag (g) if it is set. Since every search in RegEx is discrete, this indicator has no further effect on the displayed results.s
: This flag means dotAll. A period (.
) matches each character, including the new line.
Syntax of RegEx in JavaScript
const regEx = /pattern/;
const regEx = new RegExp('pattern');
Example Code:
<form name="form1">
<input type='text' id="phoneNumber" name='text1'/>
<button type="submit" name="submit" onclick="phonenumber()">Submit</button>
</form>
function phonenumber() {
const indiaRegex = /^\+91\d{10}$/;
const inputText = document.getElementById('phoneNumber').value;
if (inputText.match(indiaRegex)) {
console.log('Valid phone number');
} else {
console.log('Not a valid Phone Number');
}
}
Output:
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn