Nested Map in JavaScript
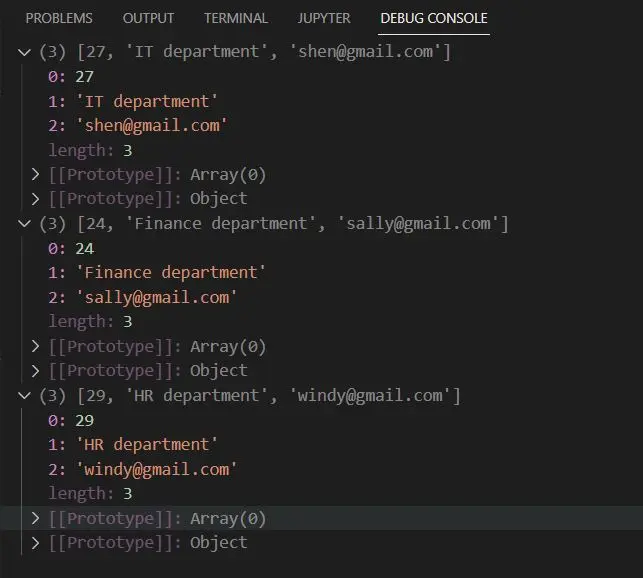
JavaScript is the world’s most popular programming language for web development, along with HTML and CSS.
In JavaScript, data can be stored in pairs using a data structure called Map. They included unique keys and values which map to the key.
JavaScript ES6 version initialized the map()
method, which detains key and value pairs where the keys can be any data type. It creates an array that calls a given function on every element calling on the array and adds a result to the new array.
This article mainly focuses on a nested map based on the map()
method. It creates an array of arrays with the map()
method to perform provided function on each element in the parent array by the iterating array.
Create Nested Map in JavaScript
Let’s take a look at how to create a map. Following is the standard syntax to create a map along with key-value pairs.
const map = new Map([iterable])
As per the above syntax, const
is a keyword that defines a new map. We can create a new map object with a new Map()
.
Inside that, we can define key-value pairs; in the above code, it is defined as iterable
. We can create an array map as follows using the above syntax.
<!DOCTYPE html>
<html>
<title>Map javascript</title>
<body>
<p id="map"></p>
<script>
const books = new Map([["Beloved",400 ],
["Jane Eyre", 500],
["Lolita", 700]]);
let string = "";
for(const booksName of books.entries()){
string += booksName + "<br>"
}
document.getElementById("map").innerHTML = string;
</script>
</body>
</html>
Considering the above example, we create a new map()
as an object. It declared three key-value pairs.
We can define a variable to assign these key-value pairs.
To print them, we can use the for
loop with the entries()
method, which iterates through the key-value pairs in the array. So, this is how we create a map()
.
The following is the result of the above code.
So, as we create a map()
, we can create a nested map, otherwise an array of arrays. Initially, let’s look at how to create a nested array in JavaScript.
const flowers = [['Rose', 'Red'], ['Sunflower', 'Yellow'], ['Tulips', 'white']]
We can put square brackets to create an array inside it and another square bracket to create an array.
Following is the syntax used to call a function on every element of the array and add it to the new array used to create a nested map.
array.map(function(currentValue, index, arr), thisValue)
We should define a function to call for each element, and the currentValue
is the current element’s value. Index performs the index of the current value.
The arr
defines the array of the current element. Finally, we have thisValue
, the default value of the passed function.
Then the function can be used as its thisValue
function, and currentValue
parameters are required out of other parameters, and others are optional.
Let’s consider the following example.
<!DOCTYPE html>
<html>
<title>Map javascript</title>
<body>
<p id="map"></p>
<script>
const person = [{name:"Shen", info:[27,"IT department","shen@gmail.com"]},
{name:"Sally", info:[24,"Finance department","sally@gmail.com"]},
{name:"Windy", info:[29,"HR department","windy@gmail.com"]}]
person.map((person,index) =>{console.log(person.info)})
</script>
</body>
</html>
There is an array called the person in the above example. It has declared a nested array called info
.
This nested array included three different values.
Then person
array assigns to the map()
method. Inside the map()
method, there are two parameters: person
and index
.
For each parameter, the person passes an array, and the index
gives an index of each element of the array. Using the arrow
function, we can call the console.log
method to get info which is a nested array.
So, the result can obtain as follows:
So, as we can see info
array was created as a new array using the map()
method. This is one of the ways that use the nested map.
Also, we can provide a function to perform on each element on a nested array as follows.
<!DOCTYPE html>
<html>
<title>Map javascript</title>
<body>
<p id="map"></p>
<script>
const num = [[14,8],[5,31],[23,41]]
function squareRoot(x){
return x*2;
}
var newArr = num.map(subarray =>subarray.map( squareRoot ));
console.log(newArr)
</script>
</body>
</html>
The above code is used to get a square root of elements in the array num
. It has three nested arrays.
We can create a function to call each element on this array. In the above example, multiply each element of arrays in 2 (x*2
).
After that assigned new array is as newArr
to store the final result. map()
method calls a function squareRoot
and iterates all over the elements in subarrays.
So, we can get the output as follows.
As we can see, nested arrays are assigned as a new array using the map()
method after performing the function on each element.
This is how we can work on nested maps. We can perform any function in each value of nested arrays using the map()
method.
Conclusion
This article indicates how to work nested maps in JavaScript. Mainly map()
method is used to perform tasks on map elements.
The important thing is a map()
process does not run a function for empty elements. Also, we cannot change the parent array using the map()
method.
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.