How to Valid Variable Names in JavaScript
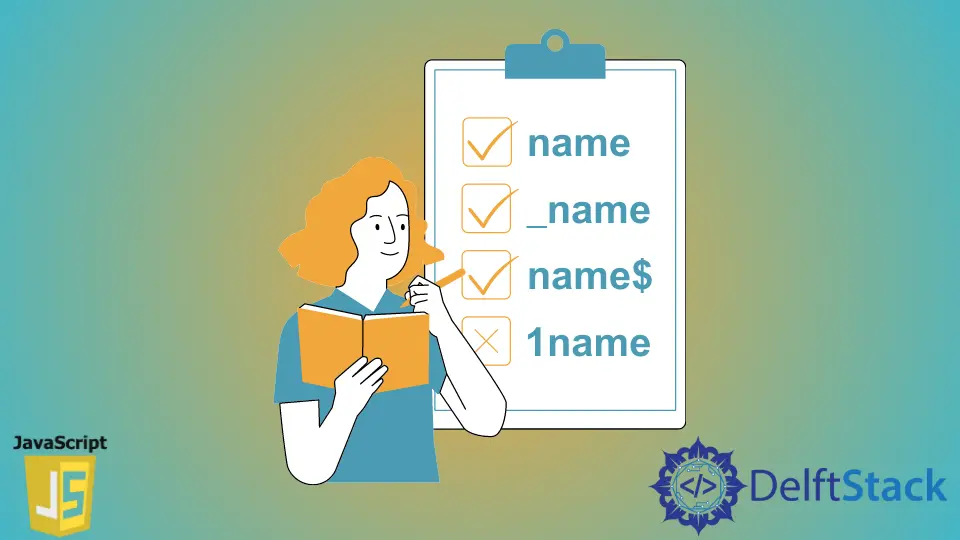
This tutorial shows the rules for JavaScript valid variable names. Before jumping there, let’s first understand the terms VARIABLE
and IDENTIFIER
.
The VARIABLE
is a container that can hold a changeable value in the memory, which means the programmer can change that value during the execution process. Every variable has a name given to the memory to access the stored value there, and that name is known as IDENTIFIER
.
JavaScript Valid Variable Names (Also Called Identifiers)
For instance, name
, age
, and gender
are called identifiers, but their actual values are the variables that can be changed.
Please observe the following example where we have the name
, age
, & gender
as identifiers (which will not be changed). Mehvish
, 30
, & F
are variables that the software developer can change during execution.
var name = 'Mehvish';
var age = 30;
var gender = 'F';
The variable names (also known as identifiers) must be meaningful and unique to avoid ambiguity in the code. Having meaningful variable names also makes the code easy for every software developer and debugging.
So, how to create a valid variable?
We have to be very careful and follow the rules for creating a valid variable name (identifier) in JavaScript. Let’s have a look at them.
- A variable name must start with an underscore (
_
), dollar sign ($
), or a letter (a-z
andA-Z
) because the JavaScript is case-sensitive (A
anda
are different for JavaScript). - A variable name can’t start with a number, but the subsequent characters can be digits (
0-9
). - We can also use the unicode escape sequences in identifiers as characters.
- A variable name can also have most of ISO 8859-1/ Unicode Letters that include
å
&ü
. More details can be found here.
Examples of Valid And Invalid Variable Names in JavaScript
Following are some of the valid and invalid JavaScript variable names.
Example Code:
var name; // valid
var 12name; // invalid
var _age; // valid
var ^ number; // invalid
var gender$; // valid
Let’s create a basic variable name validator. You can practice with the following startup code and write your own amazing variable name validator.
HTML Code:
<html>
<head>
<title>Document</title>
</head>
<body>
<input type="text" id="num" />
<input type="button" id="validateBtn" onclick="validateVariable();"
value="Validate" />
<div id="container"></div>
</body>
</html>
JavaScript Code:
var regex = '^([a-zA-Z_$][a-zA-Z\d_$]*)$';
function validateVariable() {
var input = document.getElementById('num');
if (input.value == '' || input.value == 'null')
alert('The element can\'t be empty or null');
else {
if ((input.value).match(regex))
document.getElementById('container').innerHTML =
input.value + ' is a valid variable name';
else
document.getElementById('container').innerHTML =
input.value + ' is an invalid variable name';
}
input.value = '';
}
Output:
You can use this tool to confirm that the regex is correctly designed. There is another amazing tool that you can use to check the complex variable names’ validity.
Related Article - JavaScript Variable
- How to Access the Session Variable in JavaScript
- How to Check Undefined and Null Variable in JavaScript
- How to Mask Variable Value in JavaScript
- Why Global Variables Give Undefined Values in JavaScript
- How to Declare Multiple Variables in a Single Line in JavaScript
- How to Declare Multiple Variables in JavaScript