How to Submit Form With POST Request in JavaScript
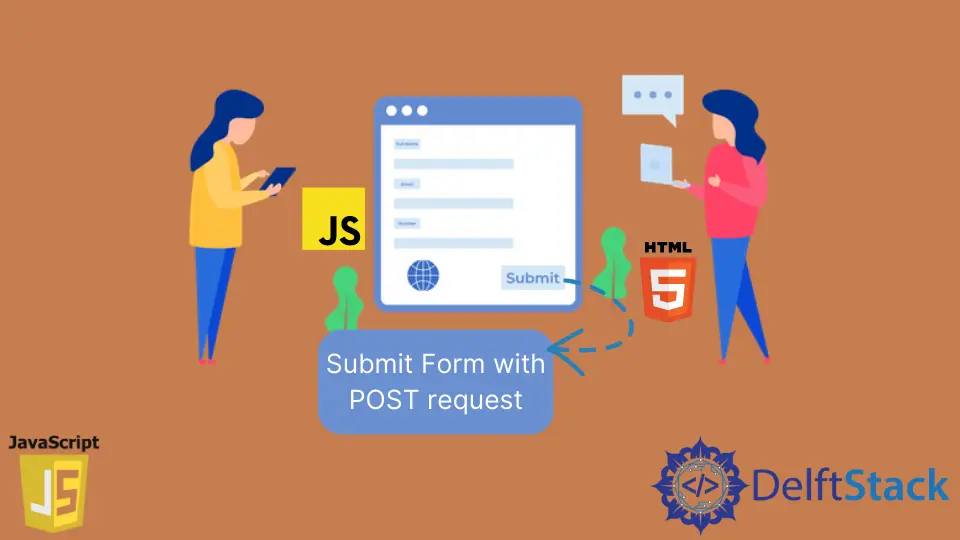
In JavaScript, you can perform a POST request with the HTML forms. The post is a method used in HTML forms to submit or send the data to the server.
Submit a Form With a POST Request in JavaScript
The below code shows you how to use JavaScript to submit a form in a POST request. Here, we will create a new post request and send the user to the new URL myPath
.
First, we have created a function that takes three arguments path (the URL or endpoint), parameters (an object with key-value pair), and the last one is the post
method which we are directly initializing at the time of function creation.
Then using document.createElement()
method we will create a form element and store it inside the form
variable. Then we will initialize this form.method
with the post method and then form.action
with the path. In the end, we will append the form we have created to the body
tag present inside the DOM.
function sendData(path, parameters, method = 'post') {
const form = document.createElement('form');
form.method = method;
form.action = path;
document.body.appendChild(form);
for (const key in parameters) {
const formField = document.createElement('input');
formField.type = 'hidden';
formField.name = key;
formField.value = parameters[key];
form.appendChild(formField);
}
form.submit();
}
sendData('/myPath/', {query: 'hello world', num: '1'});
At this point, we have created and appended the form to the body element, and currently, the form does not contain any elements inside it. So, to add the data inside the form, we will use the parameters
object, which we are passing to our sendData()
function. To iterate over this object, we will use a for loop and grab each key from the object.
Then we will create an input
form field and store it inside the formField
variable. Now we will set its type to hidden
since we don’t want to show this form inside on our webpage. At last, we will initialize the form fields name
and value
parameters with the parameters
object’s key and value.
Since in our case, we are passing an object which contains two key-value pairs; therefore, the for loop will for two times. After which, we will use the submit()
method to perform the post request and send the user to a different endpoint or URL i.e myPath
. The sendData()
function is called by passing these parameters sendData('/myPath/', {query: 'hello world', num: '1'});
.
If you run the above code, it will make a POST request and then take you to a new endpoint, as shown below.
An alternative way of doing this is by creating the entire form inside the HTML itself instead of JavaScript. And then accessing this form and its elements inside our JavaScript code as follows.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<form id="form" action="https://www.wikipedia.org/" method="POST">
<input id="formField" type="hidden" name="Director" value="James Cameron">
</form>
<script src="./script.js"></script>
</body>
</html>
function postData(path, name, value) {
document.getElementById('formField').name = name;
document.getElementById('formField').value = value;
document.getElementById('form').action = path;
document.getElementById('form').submit();
}
postData('https://www.wikipedia.org/', 'Writer', 'Jim Collins');
Here, we are directly adding the method
as POST
to the HTML form itself. Inside our postData()
method we are just passing the path
, name
and value
as postData("https://www.google.com/","Writer","Jim Collins");
. Then we will access the formField
using the document.getElementById()
method and then set the values appropriately as shown above. After running this code, it will perform a POST request and take the user to the Wikipedia website, as shown below.
The above code works the same way as that of the previous code, but it is much more concise and readable.
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn