How to Scroll to ID in JavaScript
-
Use the
element.scrollIntoView()
Method to Scroll to ID in JavaScript -
Use the
window.scrollTo()
Method With Manual View Calculation to Scroll to ID in JavaScript -
Use the
scrollTo()
Method WithscrollLeft
andscrollTop
to Scroll to ID in JavaScript - Conclusion
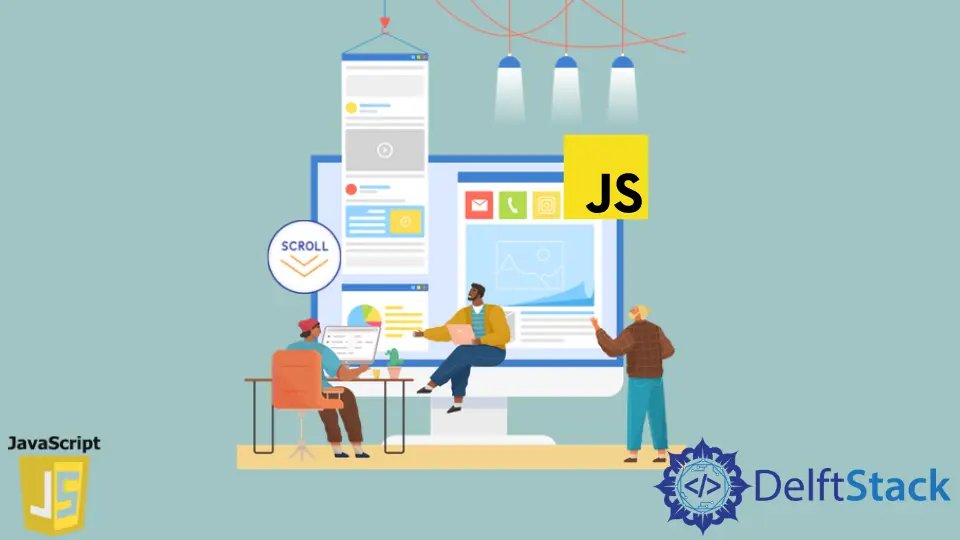
Scrolling to a specific section on a webpage can enhance user experience and improve navigation. In JavaScript, there are several methods to achieve this.
In the general case, when we set an anchor tag in our HTML body and set the href
to a specific ID, we can scroll to that element. However, this practice is not mostly welcomed for dynamic cases.
When scrolling to an ID of the same webpage, we call it internal linking. We will see ways of performing internal linking via JavaScript methods in this article’s examples.
The scrollIntoView()
method is the most reliable way of scrolling to a particular ID. But the most important case is to get the correct querySelector
.
Only then will it be possible to route to that element. Then, we will also use the scrollTo()
method to set up the offset remarks.
Let’s check these methods for a better understanding.
Use the element.scrollIntoView()
Method to Scroll to ID in JavaScript
To scroll to a particular ID in JavaScript, we can employ the scrollIntoView()
method.
The scrollIntoView()
method is a built-in function in JavaScript that’s specifically designed to bring an element into view within the viewport. It is used to scroll an element into the visible area of the browser window or container.
Its basic syntax is as follows:
element.scrollIntoView(options);
Here, element
refers to the DOM element you want to scroll into view, and options
is an optional parameter that allows you to customize the scrolling behavior. The options
parameter is an object with properties that control various aspects of the scrolling operation.
Basic Example:
var element = document.getElementById('exampleElement');
element.scrollIntoView();
In this example, the element with the ID exampleElement
will be scrolled into view using the default scrolling behavior.
Basic Example with Options:
element.scrollIntoView({
behavior: 'smooth',
block: 'start',
inline: 'nearest'
});
In this example, the behavior
property is set to 'smooth'
, which results in a smooth animated scroll. The block
property is set to 'start'
, which aligns the top edge of the element with the top of the viewport.
The inline
property is set to 'nearest'
, which aligns the element with the nearest edge of the viewport.
Options:
-
behavior
(optional): Determines the scrolling behavior. It accepts two values:'auto'
: Default behavior, providing an immediate jump to the target element.'smooth'
: Creates a smooth, animated scroll.
-
block
(optional): Defines the vertical alignment of the element within the viewport. Possible values are:'start'
: Aligns the top edge of the element with the top of the viewport.'center'
: Places the element in the center of the viewport.'end'
: Aligns the bottom edge of the element with the bottom of the viewport.
-
inline
(optional): Specifies the horizontal alignment. Options include:'start'
: Aligns the element with the left edge of the viewport.'center'
: Centers the element horizontally.'end'
: Aligns the element with the right edge of the viewport.
options
is not provided, the element will be scrolled into view with the default behavior, which is an immediate jump to the target element.Example - Scroll Up:
To scroll to elements in the upper segment from the action link, we will set the parameter of scrollIntoView()
to true
. After clicking the link, this ensures that the page will be stressed down, and we will reach the target above the page.
Code Snippet:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>Document</title>
<style>
#blue{
height: 200px;
background: blue;
}
#buff{
height:800px;
}
a{
text-decoration: none;
color: black;
}
</style>
</head>
<body>
<div id="blue"></div>
<div id="buff"></div>
<a href="javascript: scroll();">Get to BLUE</a>
<script>
function scroll(){
var getMeTo = document.getElementById("blue");
getMeTo.scrollIntoView({behavior: 'smooth'}, true);
}
</script>
</body>
</html>
This HTML document sets up a one-page website with a blue section and a link that when clicked, smoothly scrolls up to the blue section.
The scroll()
function, triggered by the link, uses the scrollIntoView()
method to achieve this effect, with options for smooth scrolling and alignment. The CSS styles define the appearance of the sections and links.
Output:
Example - Scroll Down:
Similarly, here we will set the additional parameter to false
, and thus the difference is created.
Code Snippet:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>Document</title>
<style>
#blue{
height: 200px;
background: blue;
}
#buff{
height:800px;
}
a{
text-decoration: none;
color: black;
}
</style>
</head>
<body>
<a href="javascript: scroll();">Get to BLUE</a>
<div id="buff"></div>
<div id="blue"></div>
<script>
function scroll(){
var getMeTo = document.getElementById("blue");
getMeTo.scrollIntoView({behavior: 'smooth'}, false);
}
</script>
</body>
</html>
This HTML document establishes a one-page website featuring a link. When clicked, the link triggers a smooth scroll down to a blue-colored section.
The page structure includes CSS styling for the blue section, a spacer element, and the link. JavaScript is used to implement the smooth scrolling effect, aligning the blue section’s top with the viewport’s top.
Output:
Example with Options:
Here’s a complete HTML code example demonstrating the usage of scrollIntoView()
with options to scroll to an element with a specific ID:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Scrolling with ScrollIntoViewOptions</title>
<style>
#red {
height: 300px;
background-color: red;
}
#blue {
height: 300px;
background-color: blue;
}
#green {
height: 300px;
background-color: green;
}
a {
display: block;
margin: 10px 0;
cursor: pointer;
color: black;
text-decoration: none;
}
</style>
</head>
<body>
<a href="javascript:scrollToElement('red')">Scroll to Red Section</a>
<a href="javascript:scrollToElement('blue')">Scroll to Blue Section</a>
<a href="javascript:scrollToElement('green')">Scroll to Green Section</a>
<div id="red"></div>
<div id="blue"></div>
<div id="green"></div>
<script>
function scrollToElement(elementId) {
var element = document.getElementById(elementId);
element.scrollIntoView({
behavior: 'smooth',
block: 'start',
inline: 'nearest'
});
}
</script>
</body>
</html>
In this example, we have three sections (red
, blue
, and green
) with different background colors. Above these sections, we have three clickable links to scroll to each section respectively.
-
When a link is clicked, it triggers the
scrollToElement
function. -
The function takes the
elementId
as an argument, which corresponds to the ID of the section we want to scroll to. -
Inside the function,
scrollIntoView
is called on the specified element with thescrollIntoViewOptions
object.behavior: 'smooth'
: This enables smooth scrolling animation.block: 'start'
: This ensures the element is aligned to the start of the viewport.inline: 'nearest'
: This scrolls the element to be as close as possible to the viewport.
This code will create a simple webpage where clicking on the links smoothly scrolls the page to the respective sections.
Use the window.scrollTo()
Method With Manual View Calculation to Scroll to ID in JavaScript
The window.scrollTo()
method in JavaScript allows you to programmatically set the scroll position of the document. It has two possible syntaxes:
-
Using
x
andy
coordinates:window.scrollTo(x-coord, y-coord);
Here,
x-coord
andy-coord
are the horizontal and vertical coordinates where you want the window to be scrolled.Example:
window.scrollTo(0, 500); // Scrolls to 0px horizontally and 500px vertically
-
Using an
options
object:window.scrollTo(options);
Here,
options
is an object with properties that specify the scroll behavior and position:top
: Vertical position to scroll to.left
: Horizontal position to scroll to.behavior
: Specifies the scrolling behavior, which can be'auto'
or'smooth'
.
window.scrollTo({ top: 100, left: 0, behavior: 'smooth' });
This example scrolls to
100px
from the top, aligns the left edge with the left of the viewport, and uses smooth scrolling behavior.
In the below example, we will initially select our element, and then we will also set the offset
value. For the next step, we will grab the position of the top of the body and similarly ensure our elements’ top position via the .getBoundingClientRect()
method.
Next, we will perform a general mathematical operation to get the desired measurements for the scroll. The code snippet will demonstrate this more intuitively.
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>Document</title>
<style>
#purple{
height: 200px;
background: purple;
}
#buff{
height:800px;
}
a{
text-decoration: none;
color: black;
}
</style>
</head>
<body>
<div id="purple"></div>
<div id="buff"></div>
<a href="javascript: scroll();">Get to Purple</a>
<script>
function scroll(){
const element = document.getElementById('purple');
const offset = 50;
const bodyRect = document.body.getBoundingClientRect().top;
const elementRect = element.getBoundingClientRect().top;
const elementPosition = elementRect - bodyRect;
const offsetPosition = elementPosition - offset;
window.scrollTo({
top: offsetPosition,
behavior: 'smooth'
});
}
</script>
</body>
</html>
This HTML document creates a one-page website with a purple section and a link labeled "Get to Purple"
. When clicked, the link triggers a smooth scroll up to the purple section.
The code uses JavaScript to calculate the precise scroll position based on an offset from the top. This provides a seamless and visually appealing scrolling experience for users.
Output:
Use the scrollTo()
Method With scrollLeft
and scrollTop
to Scroll to ID in JavaScript
The scrollTo()
method allows you to specify the exact scroll coordinates in both the horizontal and vertical directions. By combining this method with scrollLeft
and scrollTop
, you can precisely control the scroll position of an element.
The scrollLeft
and scrollTop
properties in JavaScript are used to get or set the number of pixels an element is scrolled horizontally and vertically, respectively. Here are the syntaxes for both:
Getting the Scroll Position:
var horizontalScrollPosition = element.scrollLeft;
var verticalScrollPosition = element.scrollTop;
In this syntax, element
refers to the DOM element for which you want to get the scroll position.
Setting the Scroll Position:
element.scrollLeft = value;
element.scrollTop = value;
In this syntax, element
is the DOM element whose scroll position you want to set, and value
is the number of pixels you want to set as the scroll position.
Examples:
Getting Scroll Position:
This code is used to get the horizontal and vertical scroll positions of an HTML element with the id
of 'myElement'
.
var element = document.getElementById('myElement');
var horizontalScrollPosition = element.scrollLeft;
var verticalScrollPosition = element.scrollTop;
Setting Scroll Position:
This code snippet sets the horizontal scroll position of the element with the ID 'myElement'
to 200
pixels and the vertical scroll position to 300
pixels.
var element = document.getElementById('myElement');
element.scrollLeft = 200; // Sets horizontal scroll position to 200 pixels
element.scrollTop = 300; // Sets vertical scroll position to 300 pixels
Keep in mind that scrollLeft
and scrollTop
are properties of elements with scrollable content. They apply to elements like div
elements with overflow
CSS property set to scroll
or auto
or elements like the window
object.
Additionally, scrollLeft
is used for horizontal scrolling, while scrollTop
is used for vertical scrolling.
The below example implies setting the attributes for the scrollTo()
method. We will take the instance of our element and set the top and left positions of the scroll via element.scrollTop
and element.scrollLeft
.
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>Document</title>
<style>
#yellow{
height: 200px;
background: yellow;
}
#buff{
height:800px;
}
a{
text-decoration: none;
color: black;
}
</style>
</head>
<body>
<div id="yellow"></div>
<div id="buff"></div>
<a href="javascript: scroll();">Get to yellow</a>
<script>
function scroll(){
var getMeTo = document.getElementById("yellow");
window.scrollTo({
top: getMeTo.scrollTop,
left: getMeTo.scrollLeft});
}
</script>
</body>
</html>
This HTML document establishes a one-page website with a yellow section and a link labeled "Get to yellow"
. Clicking the link triggers a smooth scroll up to the yellow section.
The scroll position is determined by the current scroll position of the yellow
element ID.
Output:
Conclusion
In conclusion, this article provides a comprehensive guide to scrolling to specific sections on a webpage using JavaScript. It covers three main methods: element.scrollIntoView()
, window.scrollTo()
, and scrollLeft
/scrollTop
properties. Each method is explained in detail with examples.
The element.scrollIntoView()
method is highlighted as the most reliable way to scroll to a particular element. It offers options for customizing the scrolling behavior, ensuring a smooth and visually appealing experience for users.
Additionally, the article demonstrates how to use window.scrollTo()
with both coordinates and an options object for precise control over the scroll position. The scrollLeft
and scrollTop
properties are also introduced for getting and setting scroll positions, particularly useful for elements with scrollable content.