How to Replace Space With Dash in JavaScript
-
Use
replaceAll()
to Replace Space With a Dash in JavaScript -
Use
replace()
to Replace Space With a Dash in JavaScript
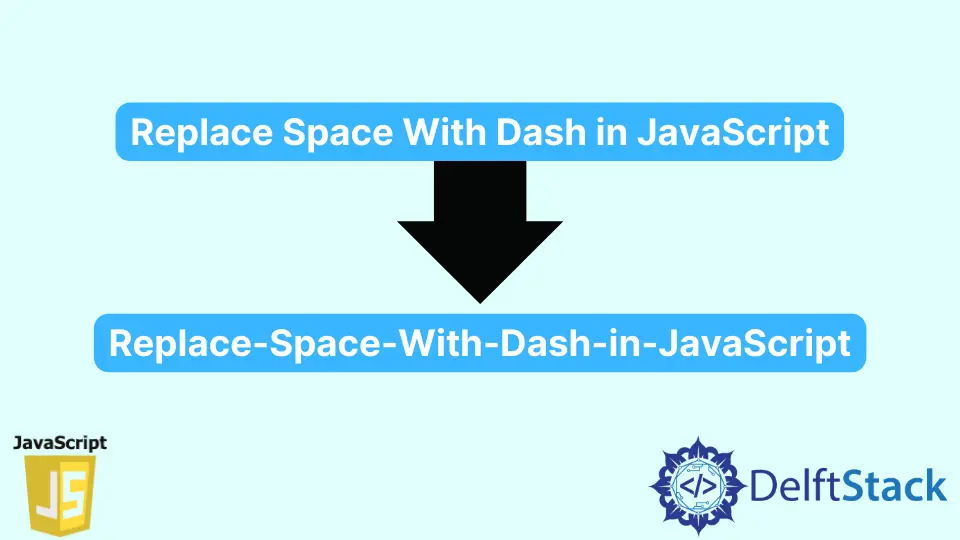
JavaScript provides two functions to replace a string with another string. Today’s post will teach us both the functions to replace space (’ ‘) with a dash (’-’).
Use replaceAll()
to Replace Space With a Dash in JavaScript
The replaceAll()
technique returns a new string with all matches of a pattern replaced by a replacement.
The pattern is often a string or a RegExp, and so the replacement may be a string or a function that must be called for each match.
Syntax:
replaceAll(regexp, newSubstr)
replaceAll(regexp, replacerFunction)
replaceAll(substr, newSubstr)
replaceAll(substr, replacerFunction)
The regexp
or pattern is an object or literal with the global flag. Matches are replaced with newSubstr
or the value returned by the specified replacement function.
A RegExp without the global flag g
raises a TypeError: replaceAll must be called with a regular expression
. The substr
is a string that should be replaced with newSubstr
.
It is treated as a literal string and not interpreted as a regular expression.
The newSubstr
or replace
is the string that replaces the specified substring with the specified regexp
or substr
parameter. Several special replacement patterns are allowed.
The replacerFunction
or replacement
function is called to create the new substring used to replace matches with the specified regular expression or substring.
A new string is returned as output, with all matches of a pattern replaced with a replacement.
Further information on the replaceAll
function can be found in this documentation.
const p = 'Hello World! Welcome to my blog post.';
console.log(p.replaceAll(' ', '-'));
const regex = /\s/ig;
console.log(p.replaceAll(regex, '-'));
In the example above, we replaced the space with the string and applied ‘-’ to the declaration as a new string. If you want to replace a complex string, you can use regex.
It automatically finds the appropriate pattern and replaces it with the replaceAll
function or the replace string.
Output:
"Hello-World!-Welcome-to-my-blog-post."
"Hello-World!-Welcome-to-my-blog-post."
Use replace()
to Replace Space With a Dash in JavaScript
The replace()
technique returns a new string with all matches of a pattern replaced by a replacement.
The pattern is often a string or a RegExp, and so the replacement may be a string or a function that must be called for each match.
If the pattern is the string, it will only replace the first matching occurrence.
Syntax:
replace(regexp, newSubstr)
replace(regexp, replacerFunction)
replace(substr, newSubstr)
replace(substr, replacerFunction)
The regexp
or pattern is an object or literal with the global flag. Matches are replaced with newSubstr
or the value returned by the specified replacement function.
A RegExp without the global flag g
raises a TypeError: replace must be called with a regular expression
. The substr
is a string that should be replaced with newSubstr
.
It is treated as a literal string and not interpreted as a regular expression.
The newSubstr
or replace
is the string that replaces the specified substring with the specified regexp
or substr
parameter. Several special replacement patterns are allowed.
The replacerFunction
or replacement
function is called to create the new substring used to replace matches with the specified regular expression or substring.
A new string is returned as output, with all matches of a pattern replaced with a replacement.
Further information on the replace
function can be found in this documentation.
const p = 'Hello World! Welcome to my blog post.';
console.log(p.replace(' ', '-'));
const regex = /\s/ig;
console.log(p.replace(regex, '-'));
In the example above, we replaced the space with the string and applied ‘-’ to the declaration as a new string. If you want to replace a complex string, you can use regex.
It automatically finds the appropriate pattern and replaces it with the replace
function or the replace string.
Output:
"Hello-World! Welcome to my blog post."
"Hello-World!-Welcome-to-my-blog-post."
The only difference between replace
and replaceAll
is that if the search argument is a string, replaceAll()
replaces all occurrences of search with replacement value or function.
In contrast, replace()
only replaces the first occurrence.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn