How to Reload DIV in JavaScript
-
Use
window.location.href
in.load()
to Reload adiv
in JavaScript -
Use
" #id > *"
With.load()
to Reload adiv
in JavaScript -
Use
window.setInterval()
to Refresh adiv
in JavaScript
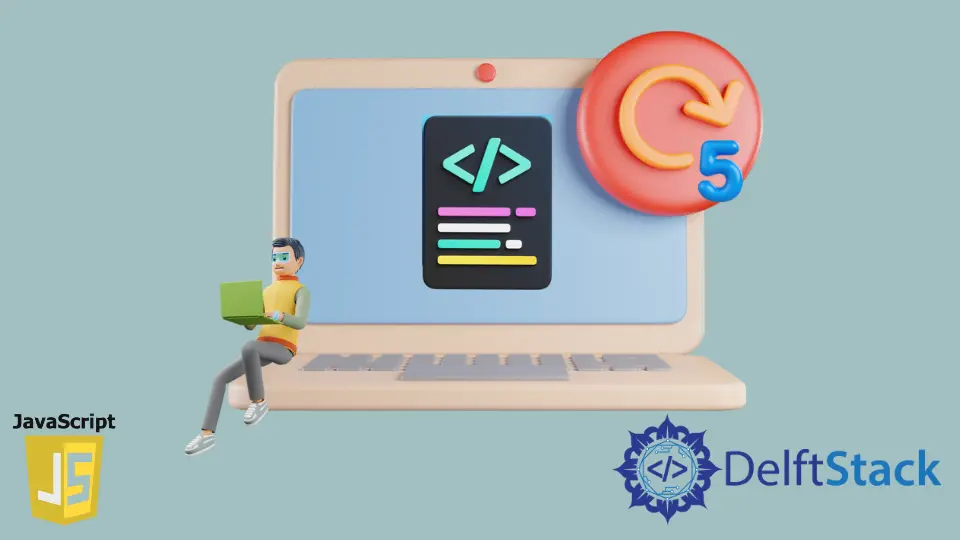
Finding a one-line coding convention to reload a div
element in JavaScript is hard. The general way to reload or refresh is to adjust the onload
function or trigger the whole webpage.
This might not always be preferred if the webpage is bulky; more likely, we want an agile way to refresh certain sections.
To solve this issue, we will solely depend upon the jQuery .load()
function that takes the current path of the webpage and adds the div
’s id along with it. This is to ensure that we will specifically add the id
to represent the location of the div
in our webpage content and refresh it.
Another way we will follow is to track the time-bound after when we wish to refresh the div
. In this case, we will require a simple dynamic attribute in HTML to collaborate with the user-defined time-bound.
This might not seem as dedicated as jQuery’s.load()
, but it can be comparatively easy to deal with via JavaScript. Let’s jump on to the code blocks.
Use window.location.href
in .load()
to Reload a div
in JavaScript
The most important segment in this example is the id
attribute that would take the targeted div
instance. We will load that part of the webpage with the div
and not the entire one.
Initially, we will have a div
with a span
tag to calculate the time frame (to check if it is working fine). Later we will take an instance of the div
(#here
) to use the load()
function.
In the .load()
function, our parameter would be the window.location.href
, which refers to the path of the current website. And associated with the #here
id
will allocate the destination and refresh it.
Code Snippet:
<script src="https://code.jquery.com/jquery-3.6.0.min.js" integrity="sha256-/xUj+3OJU5yExlq6GSYGSHk7tPXikynS7ogEvDej/m4=" crossorigin="anonymous"></script>
<div>
<div id="here">dynamic content <span id='off'>9</span></div>
<div>ABC</div>
</div>
<script>
$(document).ready(function(){
var counter = 9;
window.setInterval(function(){
counter = counter - 3;
if(counter>=0){
document.getElementById('off').innerHTML=counter;
}
if (counter===0){
counter=9;
}
$("#here").load(window.location.href + " #here" );
}, 3000);
});
</script>
Output:
Here, our counter is changed after every 3 seconds. Due to the screen-grab, the timing ratio can be at least 3 seconds, but it does infer that the process is functioning.
Use " #id > *"
With .load()
to Reload a div
in JavaScript
In this section we will again use the .load()
function and this time as the parameter we will pass " #here > *"
. Consider adding a space before denoting the #id
.
This overall section will perform like the other example explained.
Code Snippet:
<script src="https://code.jquery.com/jquery-3.6.0.min.js" integrity="sha256-/xUj+3OJU5yExlq6GSYGSHk7tPXikynS7ogEvDej/m4=" crossorigin="anonymous"></script>
<div>
<div id="here"> again dynamic content <span id='off'>3</span></div>
<div>ABC</div>
</div>
<script>
$(document).ready(function(){
var counter = 3;
window.setInterval(function(){
counter--;
if(counter>=0){
document.getElementById('off').innerHTML=counter;
}
if (counter===0){
counter=3;
}
$("#here").load(" #here > *");
}, 1000);
});
</script>
Output:
Use window.setInterval()
to Refresh a div
in JavaScript
Here, we will take a div
and set its id
attribute. In the script section, we will make our count clock active.
This example can be a good example where we have an array of content to show on the webpage after a certain amount of times. Supposedly, we have multiple div
to present, and after each full countdown of time, we will change the array index.
Thus we will have a dynamic way of dealing with many contents.
In the example below, we haven’t fetched any such array data. Rather we just initiated the clock cycle. Let’s check the code fence for a better preview.
<div>
The DIV below will refresh after <span id="cnt" style="color:red;">7</span> Seconds
</div>
</body>
<script>
var counter = 7;
window.setInterval(function () {
counter--;
if (counter >= 0) {
var span;
span = document.getElementById("cnt");
span.innerHTML = counter;
}
if (counter === 0) {
counter = 7;
}
}, 1000);
</script>
Output: