How to Redirect to Relative URL in JavaScript
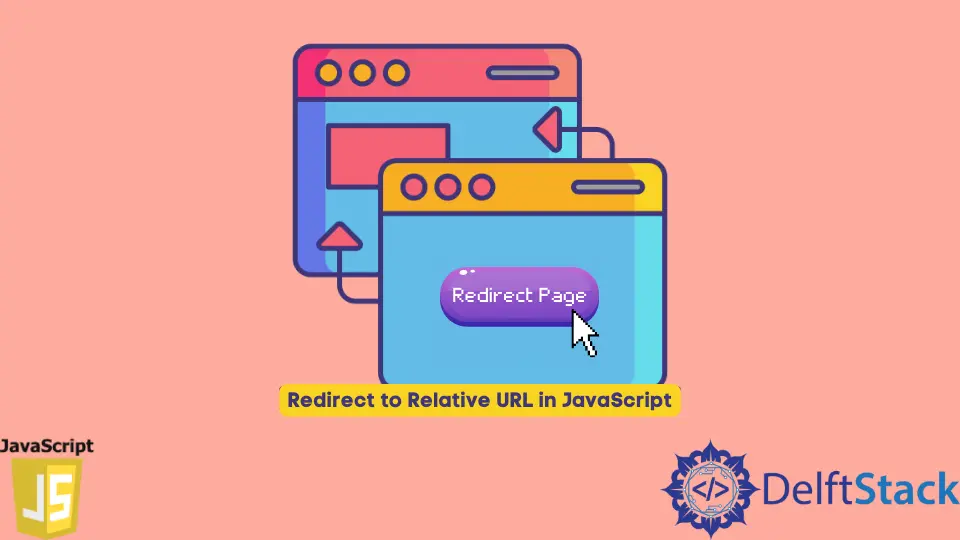
On your websites, you might need to redirect pages often. Although it is advised to turn web pages and URLs from the server side, there may be situations when you need to use JavaScript to redirect pages from the client-side.
We will discover how to shift using JavaScript in this tutorial.
Redirect to Relative URL in JavaScript
Although most JavaScript libraries, such as jQuery, have premade functions to redirect web pages, using plain JavaScript is always more straightforward. There are two JavaScript redirect methods.
Consider the scenario where you wish to reroute to www.newwebsite.com/new-location
. In this situation, you can include one of the following commands in the JS code of your web page.
Syntax:
window.location.replace("/url or path"); //or
window.location.href = "/url or path";
The redirect will not add a record to the browser’s history in the first instance. However, similar to clicking on another URL from your website, a description will be added in the second instance.
Example:
<!DOCTYPE html>
<html>
<head>
<script>
function nUrl() {
window.location.href = "b.html";
}
</script>
</head>
<body>
<input type="button" value="Redirect page" onclick="nUrl()" />
</body>
</html>
b.html
.Output:
When you click on the Redirect page
button, the page link will be redirected like this:
Note the following:
-
JavaScript cannot be used to redirect from an HTTPS web page to an HTTP website. The majority of browsers won’t let you do it.
You may redirect from HTTP to HTTP, HTTP to HTTPS, and HTTPS only to HTTPS pages using JavaScript. You must use the server-side redirecting from an HTTPS to an HTTP website.
-
You can use absolute paths (like
/new-location
) or complete URLs (likehttp://www.newwebsite.com/new-location
). The browser will reroute to the website using the same HTTP/HTTPS protocol without modifying it if you specify a relative path for redirection.It would be best if you supplied the whole URL to redirect from an HTTP to an HTTPS page.
-
It might not work if you place the redirection in the function handler for the
Submit
button since the browser will submit the form rather than rerouting the page. In these circumstances, you may use AJAX to submit the form and then redirect based on the button’s answer.
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedIn