How to Print Array Elements in JavaScript
- Different Ways to Print Array Elements in JavaScript
-
Use
for
Loop to Print Array Elements in JavaScript -
Use
while
Loop to Print Array Elements in JavaScript -
Use
forEach
Method to Print Array Elements in JavaScript -
Use
console.log()
Function to Print Array Elements in JavaScript -
Use
map()
Withjoin()
Method to Print Array Elements in JavaScript -
Use
toString()
Function to Print Array Elements in JavaScript -
Use
join()
Function to Print Array Elements in JavaScript -
Use
pop()
Function to Print Array Elements in JavaScript -
Use
shift()
Method to Print Array Elements in JavaScript -
Use
concat()
Method to Print Array Elements in JavaScript -
Use
slice()
Method to Print Array Elements in JavaScript
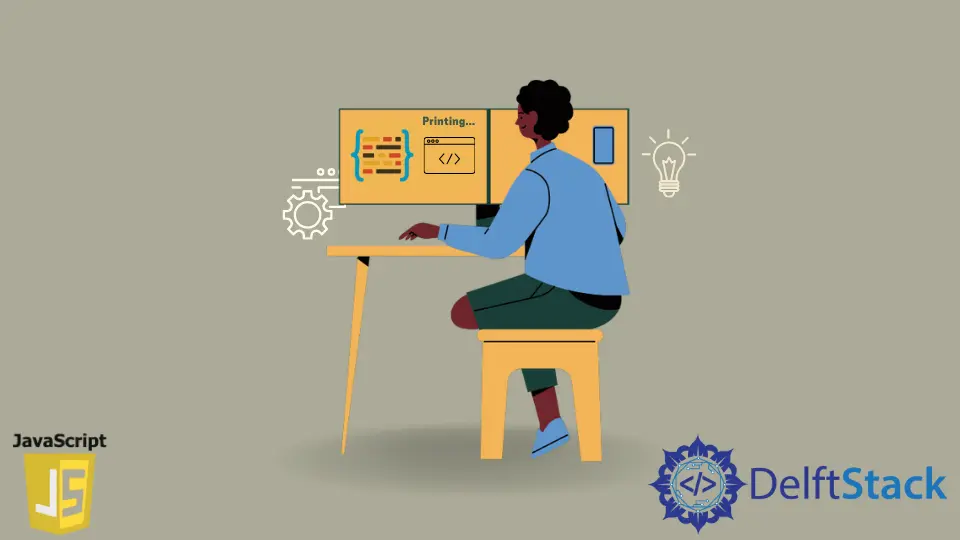
This tutorial demonstrates how you can print JavaScript array elements. It also depends on your project needs, whether you want to print the whole array or a particular number of elements.
Or you want to print array elements in string format or something else. Let’s explore different ways to print JavaScript arrays.
Different Ways to Print Array Elements in JavaScript
The for
and while
loops iterates over the array elements, one at a time, and each element is printed on a separate line while we can get this same output using the forEach()
method.
The forEach()
function runs the particular method once for every array element.
We can print all array elements by passing the array’s name to the console.log()
function. We can also use map()
and join()
methods to manipulate first and then print the array’s elements.
For instance, we want to number each array element in the output.
The map()
function creates a new array from calling a function once for each element but does not change the original array. Remember, it does not run the function for an empty element.
The join()
function acts like a separator that separates the array’s elements with a specified separator.
The pop()
returns you the last element while shift()
returns the first element of the array, but both are best to use when you want those elements to be removed from a string because both can change the original array.
The toString()
function converts the array to string format. The slice()
function gives us the selected element of the array as a new array but does not change the original array.
It can also work according to the particular start
and end
indexes (the end
index is exclusive here).
Use for
Loop to Print Array Elements in JavaScript
Let’s start with using a simple for
loop to print a complete JavaScript array.
var names = ['mehvish', 'tahir', 'aftab', 'martell'];
// for loop
for (var i = 0; i < names.length; i++) {
console.log(names[i]);
}
Output:
"mehvish"
"tahir"
"aftab"
"martell"
Use while
Loop to Print Array Elements in JavaScript
Let’s see below how the while
loop produces the output.
var names = ['mehvish', 'tahir', 'aftab', 'martell'];
var i = 0;
// while loop
while (i < names.length) {
console.log(names[i]);
i++;
}
Output:
"mehvish"
"tahir"
"aftab"
"martell"
Use forEach
Method to Print Array Elements in JavaScript
Have you thought of the forEach
method to print a complete JavaScript array (one element per line)? See the code snippet below.
var names = ['mehvish', 'tahir', 'aftab', 'martell'];
names.forEach(element => console.log(element));
Output:
"mehvish"
"tahir"
"aftab"
"martell"
Use console.log()
Function to Print Array Elements in JavaScript
We have explored the ways that print the complete array but one element on each line. You can print all array elements on a single line as follows.
var names = ['mehvish', 'tahir', 'aftab', 'martell'];
console.log(names);
Output:
["mehvish", "tahir", "aftab", "martell"]
Use map()
With join()
Method to Print Array Elements in JavaScript
We can also display all elements in one line using the following way as well.
var names = ['mehvish', 'tahir', 'aftab', 'martell'];
var element = names.map((e, i) => (i + 1 + '.' + e)).join(' ');
console.log(element);
Output:
"1.mehvish 2.tahir 3.aftab 4.martell"
Use toString()
Function to Print Array Elements in JavaScript
Have you thought that we have a function named toString()
that can convert the array into a string. See the following code example.
var names = ['mehvish', 'tahir', 'aftab', 'martell'];
console.log(names.toString());
Output:
"mehvish,tahir,aftab,martell"
Use join()
Function to Print Array Elements in JavaScript
We saw the array is converted into string above, but each element is separated with a comma. What if we want all elements to be separated into a single space? For that, the join()
function is used.
var names = ['mehvish', 'tahir', 'aftab', 'martell'];
console.log(names.join(' '));
Output:
"mehvish tahir aftab martell"
Use pop()
Function to Print Array Elements in JavaScript
Are you thinking to print only the last element of the array? We can do that by using the pop()
function.
var names = ['mehvish', 'tahir', 'aftab', 'martell'];
console.log(names.pop());
Output:
"martell"
Use shift()
Method to Print Array Elements in JavaScript
In the following code, the shift()
method prints the first element of the array.
var names = ['mehvish', 'tahir', 'aftab', 'martell'];
console.log(names.shift());
Output:
"mehvish"
Use concat()
Method to Print Array Elements in JavaScript
We have two separate JavaScript arrays and print both in one line. We want the elements of the second array after the first array’s elements.
We will concatenate the fruitsArrayTwo
with fruitsArrayOne
using the Concat()
method.
var fruitsArrayOne = ['apple', 'banana', 'grapes'];
var fruitsArrayTwo = ['mango', 'water-melon'];
console.log(fruitsArrayOne.concat(fruitsArrayTwo));
Output:
["apple", "banana", "grapes", "mango", "water-melon"]
Use slice()
Method to Print Array Elements in JavaScript
We can also print a certain array of elements using the slice()
method. We can also use the splice()
method to add and remove the array’s elements. For details, check this page.
var names = ['mehvish', 'tahir', 'aftab', 'martell'];
console.log(names.slice(0, 2)); // end index is not inclusive here
Output:
["mehvish", "tahir"]
Related Article - JavaScript Array
- How to Check if Array Contains Value in JavaScript
- How to Create Array of Specific Length in JavaScript
- How to Convert Array to String in JavaScript
- How to Remove First Element From an Array in JavaScript
- How to Search Objects From an Array in JavaScript
- How to Convert Arguments to an Array in JavaScript