How to Get Type of a Variable in JavaScript
-
Using the
typeof
Operator to Find the Type of Variable -
Using the
typeof
Operator in Conditional Checks - Note:
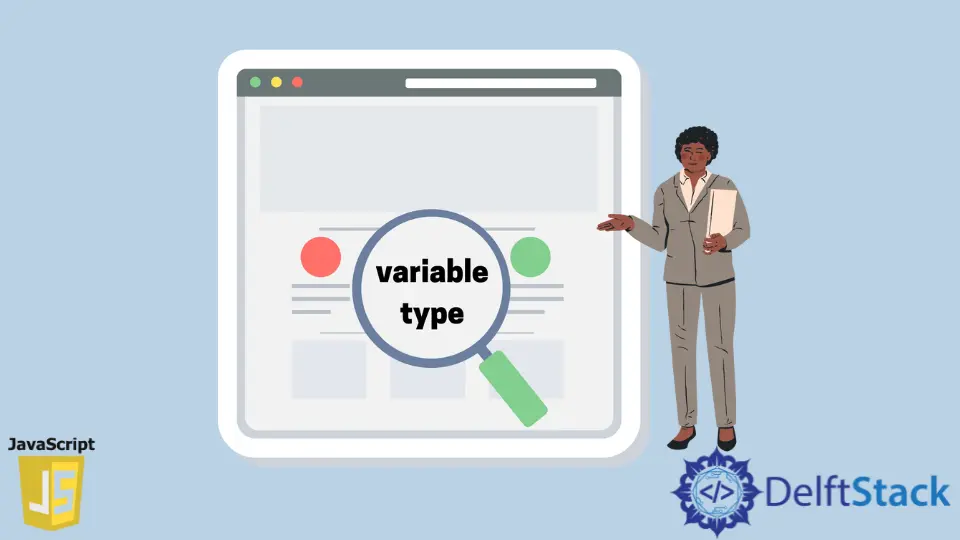
Comparing to other programming languages like C, Java etc., JavaScript gives the liberty to developers to define variables of any type with a single keyword (the var
keyword). JavaScript, in turn, decides the data type of the variable, later, depending on the values assigned to these variables. It is seemingly easy to determine the data type of a variable. But some scenarios can put us in a fix. Especially in the case of values returned by the REST API response of the server, we may need to know the type of the value or variable before we further code to process it.
Using the typeof
Operator to Find the Type of Variable
typeof
is a unary operator in javascript that returns the type of an operand to which it is applied. It usually returns the variable type as a string object. There are standard return types to the typeof
operator in javascript.
string
:typeof
returnsstring
for a variable type string.number
: It returnsnumber
for a variable holding an integer or a floating-point value.boolean
: For a variable holdingtrue
orfalse
values,typeof
returnsboolean
.undefined
: In case we do not assign values to a variable, the type of the variable will be marked asundefined
by JavaScript. Hence, thetypeof
operand will returnundefined
for such undeclared variables.object
: For variables that hold an array, or an object in{}
, or variables assigned withnull
value, javascript considers the type of such variables as an object. Hence, thetypeof
operand will returnobject
.function
: JavaScript allows us to assign functions to a variable. For such cases, the type of such variables will befunction
. Thetypeof
operator will returnfunction
for a function assignment.
The following code snippet demonstrates the behaviour of the typeof
operator for different variable assignments and different scenarios.
var s1 = 'hello';
var n1 = 120;
var n1 = 11.1234;
var b1 = true;
var x;
var u = undefined;
var o1 = null;
var o2 = {id: 1, value: 200};
var o3 = [1, 2, 3];
var f = function() {
return 1 + 2
};
console.log(typeof s1);
console.log(typeof n1);
console.log(typeof n1);
console.log(typeof b1);
console.log(typeof x);
console.log(typeof u);
console.log(typeof o1);
console.log(typeof o2);
console.log(typeof o3);
console.log(typeof f);
Output:
"string"
"number"
"number"
"boolean"
"undefined"
"undefined"
"object"
"object"
"object"
"function"
Using the typeof
Operator in Conditional Checks
We can use the typeof
operator in conditional-checks like in the if
block by checking the value returned by the operator and comparing it with the standard type values. We use the ===
operator for comparison as it includes type checks of operands at both ends of the operator.
var a = 'hello';
if (typeof a === 'string') {
console.log(true)
}
Output:
true
Similarly, we can do conditional checks for number
, boolean
, object
and even for function
. As a best practice, we should create a constant variable for the standard data types returned by the typeof
operator of javascript. Then, compare the typeof
of a variable against the declared constants. This approach makes it easy to code and reduces typos while writing the conditional blocks, which are usually not caught in a glance. Refer to the following code to understand better.
const STRING_TYPE = 'string';
const NUMBER_TYPE = 'number';
var a = 'hello';
var b = 123;
if (typeof a === STRING_TYPE) {
console.log(true)
}
if (typeof b === NUMBER_TYPE) {
console.log(true)
}
Output:
true
true
Note:
- For cases having a variable assigned with a
new
keyword, javascript will consider such an assignment as an object. Hence thetypeof
operator will returnobject
for such assignments. Refer to the following code.
var s = new String('hello');
var n = new Number(100);
console.log(typeof s);
console.log(typeof n);
Output:
object
object
- If we assign a function using the
new
keyword, then the data type of such variables is taken as a function by javascript. Thetypeof
variable withnew function()
will return asfunction
instead ofobject
. Let us look at the following code.
var fn = new Function();
Output:
"function"
- All browsers support the
typeof
operator of javascript, including the old versions of Internet Explorer. Hence we can use the operator without any worries in a project supported on multiple browsers.
Related Article - JavaScript Variable
- How to Access the Session Variable in JavaScript
- How to Check Undefined and Null Variable in JavaScript
- How to Mask Variable Value in JavaScript
- Why Global Variables Give Undefined Values in JavaScript
- How to Declare Multiple Variables in a Single Line in JavaScript
- How to Declare Multiple Variables in JavaScript