How to Filter Table in JavaScript
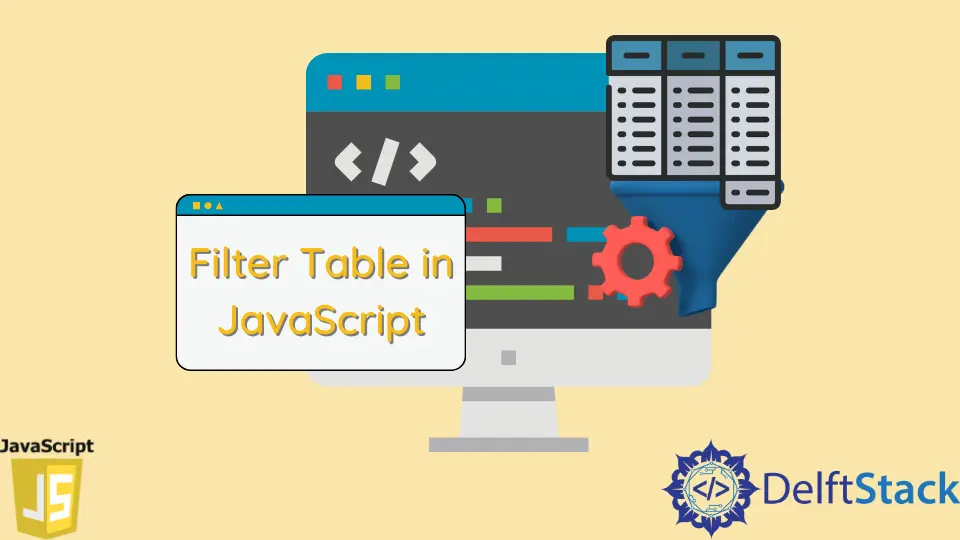
In today’s article, we will see how to filter the table using pure JavaScript.
A table is a structured data set of rows and columns (table data). In a spreadsheet, you can quickly and easily look up values that indicate some association between different data types, such as a person and their age, a day of the week, or a local pool’s hours of operation.
An HTML table is defined using the <table>
tag. Each table row is defined using the <tr>
tag.
The header of a table is defined using the <th>
tag. Table headings are bold and centered by default. The cell is defined using the <td>
tag.
Use getElementsByTagName()
and indexOf()
to Filter Table in JavaScript
The getElementsByTagName()
is a built-in document
method provided by JavaScript that returns NodeList
objects/elements whose tag
matches the specified tag name. Live HTML collections are returned, along with the root node in the search.
The getElementsByTagName()
takes its name as an input parameter. This is a required parameter that specifies the tagName
of an HTML attribute that must match.
If any matching elements are found, it returns the matched DOM element object; otherwise, it returns null
.
So let’s say we have users along with email id and names. We want to find out users whose email id ends with gmail.com
.
We can create search input, where we can enter the search query.
<input type="text" id="searchInput" onkeyup="myFunction()" placeholder="Search for names, email." title="Type in a name">
<table id="userTable">
<tr class="header">
<th style="width:60%;">Name</th>
<th style="width:40%;">Email</th>
</tr>
<tr>
<td>Alfreds Futterkiste</td>
<td>alfreds@example.com</td>
</tr>
<tr>
<td>Berglunds snabbkop</td>
<td>snabbkop@gmail.com</td>
</tr>
<tr>
<td>John Doe</td>
<td>john@dummy.com</td>
</tr>
<tr>
<td>Magazzini</td>
<td>magazzini@gmail.com</td>
</tr>
</table>
Now, let’s extract the value from search input box using getElementById()
. To solve the issue of case sensitivity, we can convert the value to either upper case or lower case.
Next step is to extract the table content and all the table rows using getElementsByTagName()
.
Once all the rows are extracted, iterate over all the rows using a for
loop to extract the individual cells inside a row. Initially, hide all the rows by changing the display property to none
.
function myFunction() {
var input, filter, table, tr, td, cell, i, j;
filter = document.getElementById('searchInput').value.toLowerCase();
table = document.getElementById('userTable');
tr = table.getElementsByTagName('tr');
for (i = 1; i < tr.length; i++) {
tr[i].style.display = 'none';
const tdArray = tr[i].getElementsByTagName('td');
for (var j = 0; j < tdArray.length; j++) {
const cellValue = tdArray[j];
if (cellValue && cellValue.innerHTML.toLowerCase().indexOf(filter) > -1) {
tr[i].style.display = '';
break;
}
}
}
}
Using getElementsByTagName
, we find an array of all the td
present inside that particular row. Now check each cell value, whether it consists of the search input or not.
If the search input matches with the cell content, unhide the row.
Now let’s run the above code and start typing gmail
; it will update the table.
Output:
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn