How to Change String Character in JavaScript
-
Change String Character Using
substring()
in JavaScript -
Change String Character Using
split()
andjoin()
in JavaScript
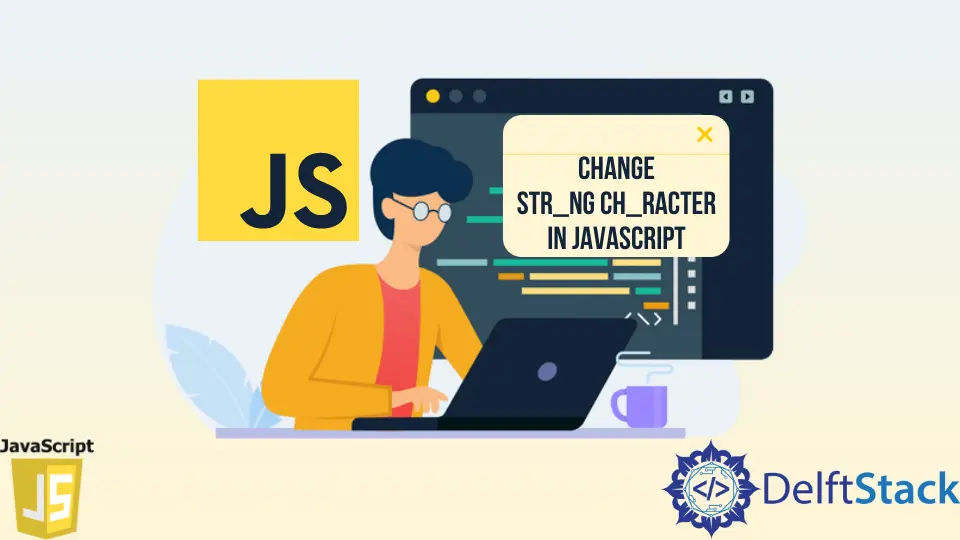
There is no built-in or default method in JavaScript to change the character in a string directly, but we can do this with the help of other string methods like substring()
, split()
, and join()
.
In this article, we will create custom functions to replace or change the character from the strings at any position we want with the help of default string methods with different examples.
Change String Character Using substring()
in JavaScript
The substring()
method is a pre-defined method in JavaScript, and we use it on strings to extract the defined characters of a string with the help of indexes. It searches the defined indexes from the complete declared string and extracts the portion from start to end.
The original string does not change by the substring()
method. It returns the new string.
Syntax:
let string = 'Hello world!';
let result = string.substring(1, 5); // result will be "ello"
Now, by using the substring()
method, we will initialize the string where we want to change the desired character at a specific position. We will be required to provide the desired character and index we want to change.
<script>
let string = "Delft stack is the b_st website to learn programming" // here we want to change "_" with "e"
function changeChar() {
let result = setCharOnIndex(string,20,'e');
console.log("Original string : "+string)
console.log("Updated string : "+result)
}
function setCharOnIndex(string,index,char) {
if(index > string.length-1) return string;
return string.substring(0,index) + char + string.substring(index+1);
}
changeChar()
</script>
Output:
"Original string : Delft stack is the b_st website to learn programming"
"Updated string : Delft stack is the best website to learn programming"
Example code explanation:
- We initialized a string containing a spelling mistake in the above JavaScript source code.
- We have declared a custom function
setCharOnIndex()
, which will take a string, index, and character as an argument. - On the provided index, it will break the passed string into two portions using the default
substring()
method. - Then, we concatenated the passed character in between and finalized the string.
- We have displayed the updated strings to see the result and differentiate the working of the methods.
- We have declared the
changeChar()
function in which we called oursetCharOnIndex()
function. - You can see the output in the console log box.
Change String Character Using split()
and join()
in JavaScript
In JavaScript, split()
is a pre-defined method that splits a declared string into a substring array. The original string does not change by the split()
method; it returns a new array of string characters.
The join()
method returns a string from an array. It will not change the original array.
We can use the split()
method along with join()
on strings to change the character in any position. We will initialize strings with spelling mistakes and test the split()
and join()
methods for changing characters at any desired index or position.
Example code:
<script>
let string = "Delft stack is the b_st website to learn programming"; // here we want to change "_" with "e"
let array = string.split(''); // converting into an array
array[20] = "e"; // added "e" in the place of "_"
let result = array.join(''); // created string again
console.log("Original string : "+string)
console.log("Updated string : "+result)
</script>
Output:
"Original string : Delft stack is the b_st website to learn programming"
"Updated string : Delft stack is the best website to learn programming"
Example code explanation:
- Again, we initialized a string containing a spelling mistake in the above JavaScript source code.
- We used the
split()
method string to split the string into a substring array. - We have assigned the
e
char on index 20 to change with_
. - We then used the
join()
method to generate the string again from the final array, which is changed. - Finally, we displayed the updated strings to see the result and differentiate the working of the methods.
- See the output in the console log box.