How to Add Text to Element in JavaScript
-
Add Text to an Existing Text Element in JavaScript via DOM Using
appendChild
-
Add Text to an Existing Text Element in JavaScript via DOM Using
textContent
-
Add Text to an Existing Text Element in JavaScript via DOM Using
innerText
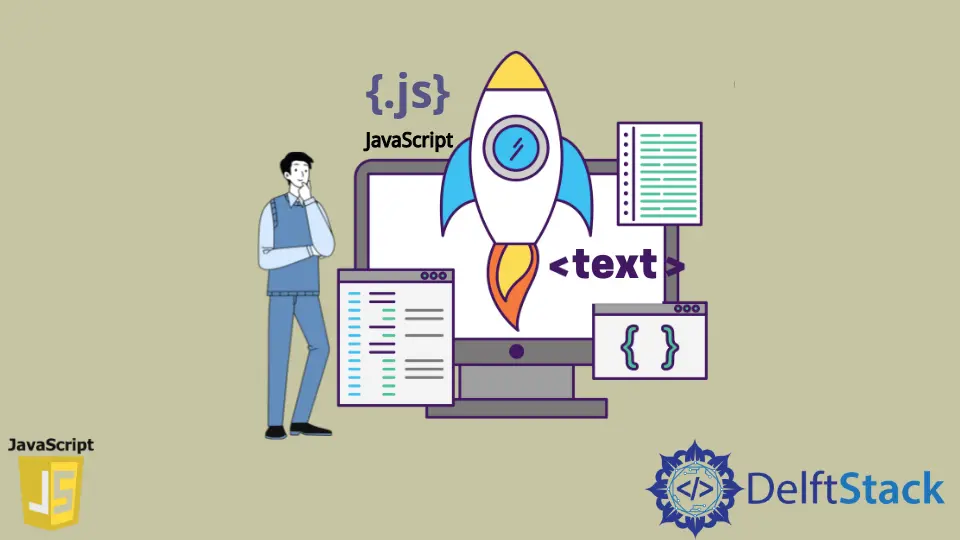
Modifying DOM content is the most basic task when it comes to browsers. Sometimes we want to change the DOM text based on certain actions without re-rendering the entire DOM. In this article, we will learn how to add text to an existing text element in JavaScript via DOM.
Add Text to an Existing Text Element in JavaScript via DOM Using appendChild
DOM contains the list of nodes. Each node contains its own data. JavaScript enables the new node instance to be added to the list of existing child nodes within the selected parent node.
Syntax:
appendChild(aChild);
The appendChild()
function takes the input parameter of a newly created node or the most common element that has to be added to the parent node. appendChild()
moves the given child from its current location to the new location if it references an existing node.
It returns the node that is the added child (a child), except when a child is a DocumentFragment. In this case, the empty DocumentFragment is returned. For more information, read the documentation of the appendChild
method.
<p id="p">Hello </p>
<p id="p1">JavaScript Channel </p>
const text = document.createTextNode('Welcome to My channel');
const pNode = document.getElementById('p');
pNode.appendChild(text);
In the above example, we have created the text node using document.createTextNode("Welcome to My channel")
and then appended that to the p
node of the document. The output of the above code will create a DOM like below.
Output:
"Hello Welcome to My channel"
Add Text to an Existing Text Element in JavaScript via DOM Using textContent
It is the property of the Node
interface, which represents the textual content of the selected node and its descendants. JavaScript allows the other text to be added to the current text content from child nodes within the selected parent node.
Syntax:
textContent = data;
The textContent property updates the text content of the selected node. Users can modify the text data as needed, such as concatenation, updating, etc. If the node is a document or a document type, textContent returns null. textContent returns the concatenation of the textContent of each child node for other node types, except comments and processing instructions. This is an empty string when a node has no children. For more information, read the documentation of the textContent
property.
const pNode = document.getElementById('p');
pNode.textContent += 'Welcome to My channel';
In the example above, we got the paragraph node using document.getElementById("p")
and then added Welcome to my channel
to the existing textual content of the paragraph node. The output of the code above updates a DOM as follows:
Output:
"Hello Welcome to My channel"
Add Text to an Existing Text Element in JavaScript via DOM Using innerText
It belongs to the HTMLElement
. This property represents the rendered textual content of the selected node and its descendants. JavaScript allows the other text to be added to the current text content from child nodes within the selected parent node.
Syntax:
const renderedText = htmlElement.innerText
htmlElement.innerText = string
The innerText property updates the text content of the selected node. Users can change the text data as needed, such as concatenation, updating, etc. For more information, see the documentation of the innerText
property.
const pNode = document.getElementById('p');
pNode.innerText += 'Welcome to My channel';
In the example above, we got the paragraph node using document.getElementById("p")
and then added Welcome to my channel
to the existing textual content of the paragraph node. The output of the code above updates a DOM as follows:
Output:
"Hello Welcome to My channel"
The only difference between textContent and innerText is that textContent gets the contents of all elements, including script
and style
elements. In contrast, innerText only displays human-readable elements. textContent
returns all elements of the node. In contrast, innerText
is style-conscious and does not return any text from hidden elements. In principle, innerText recognizes the rendered appearance of text, but textContent does not.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn