How to Export HTML Table to Excel Using JavaScript
- Basic JavaScript Codes to Export HTML Table to Excel
-
Use the
TabletoExcel
Library to Export HTML Table to Excel in JavaScript -
Use the
TableExport
Library to Export HTML Table to Excel File in JavaScript -
Use the jQuery
table2excel
Library to Export HTML Table to Excel File in JavaScript -
Use SheetJS
xlsx
to Export HTML Table to Excel File in JavaScript
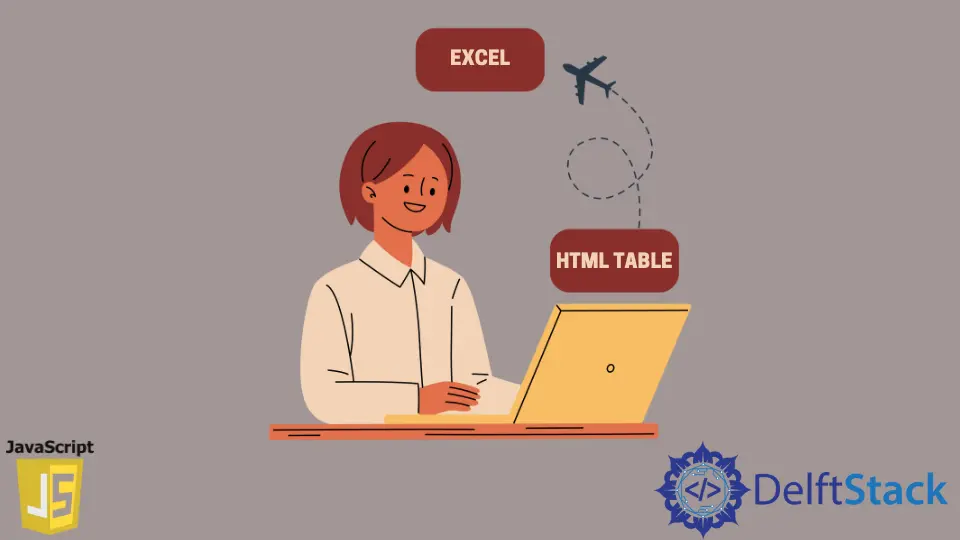
Excel spreadsheets serve as the bedrock for organizing and comprehending tabular information in the dynamic landscape of data management.
Navigating the transition from HTML tables to Excel sheets is a pivotal skill, facilitating seamless data transfer.
This tutorial covers diverse methods that empower you to seamlessly export HTML tables to Excel files, including from foundational JavaScript techniques to powerful libraries.
Basic JavaScript Codes to Export HTML Table to Excel
This method involves crafting a JavaScript function that seamlessly converts an HTML table into an Excel file, ready for download. The approach utilizes native browser features without relying on external libraries. Let’s explore the step-by-step implementation of this approach.
First, create the HTML structure that houses the table you intend to export. We will use a simple HTML table with a button to initiate the export process for demonstration purposes.
<!DOCTYPE html>
<html>
<head>
<title>Export HTML Table to Excel</title>
</head>
<body>
<table id="tableId">
<!-- Your table content here -->
</table>
<button id="exportButton">Export to Excel</button>
<script>
// JavaScript code will go here
</script>
</body>
</html>
Next, let’s implement the JavaScript function responsible for exporting the table to an Excel file. This function leverages the Blob
and URL.createObjectURL
features to create a downloadable link.
function exportTableToExcel(tableId) {
// Get the table element using the provided ID
const table = document.getElementById(tableId);
// Extract the HTML content of the table
const html = table.outerHTML;
// Create a Blob containing the HTML data with Excel MIME type
const blob = new Blob([html], {type: 'application/vnd.ms-excel'});
// Create a URL for the Blob
const url = URL.createObjectURL(blob);
// Create a temporary anchor element for downloading
const a = document.createElement('a');
a.href = url;
// Set the desired filename for the downloaded file
a.download = 'table.xls';
// Simulate a click on the anchor to trigger download
a.click();
// Release the URL object to free up resources
URL.revokeObjectURL(url);
}
// Attach the function to the export button's click event
document.getElementById('exportButton').addEventListener('click', function() {
exportTableToExcel('tableId');
});
The JavaScript function exportTableToExcel(tableId)
retrieves the HTML content of the specified table using its ID. It then creates a Blob
containing the HTML data with the Excel MIME type.
A temporary anchor (<a>
) element is created to hold the URL, and the desired filename for the downloaded file is set. By simulating a click on the anchor, the browser triggers the Excel file download. Finally, the URL object is revoked to free up resources.
When a user clicks the Export to Excel
button, the exportTableToExcel
function will be invoked. This function retrieves the table’s HTML content, converts it into a downloadable Excel file, and prompts the user to save it.
Use the TabletoExcel
Library to Export HTML Table to Excel in JavaScript
The TableToExcel
library provides a straightforward way to export an HTML table to an Excel file, streamlining the process of converting tabular data into a more widely used format. This approach simplifies data sharing and reporting within web applications.
In this section, we will delve into the details of utilizing the TableToExcel
library, focusing on the convert()
method to facilitate the export operation.
The convert()
in the TabletoExcel
Library
At the heart of the TableToExcel
library lies the convert()
method, a crucial function that bridges the gap between HTML tables and Excel files.
By calling this method, developers trigger the transformation of an HTML table into a downloadable Excel spreadsheet.
This approach not only enhances user interaction but also contributes to the seamless flow of data.
The code snippet provided below exemplifies the usage of the convert()
method in exporting an HTML table to an Excel file:
<button id="btnExport" onclick="exportReportToExcel(this)">Export HTML Table</button>
<script type="text/javascript">
function exportReportToExcel() {
let table = document.getElementsByID("table");
TableToExcel.convert(table[0], {
name: `file.xlsx`,
sheet: {
name: 'Sheet 1'
}
});
}
</script>
Code Explanation:
Breaking down the code snippet, we can identify the following key elements:
- Button Element: A button element with the ID
btnExport
is presented, allowing users to initiate the export process by clicking it. - JavaScript Function
exportReportToExcel()
: This function, triggered by the button click, serves as the entry point for exporting the HTML table to an Excel file. - Accessing the Table: The function first retrieves the HTML table element using the
getElementsByID()
method. In the provided snippet, the table’s ID is assumed to be"table"
. TableToExcel.convert()
Method: The crux of the export process lies in this method call. Theconvert()
method is invoked with the HTML table element as the first argument. Additionally, an object is passed as the second argument, containing configuration details for the export operation.name
: Specifies the name of the exported Excel file. In the example, the file will be named"file.xlsx"
.sheet
: Configures the sheet properties of the exported Excel file. In this case, the sheet is named"Sheet 1"
.
Use the TableExport
Library to Export HTML Table to Excel File in JavaScript
The TableExport
library emerges as a versatile solution for exporting HTML tables to a range of formats, including XLSX, XLS, CSV, and text files.
This library’s simplicity belies its powerful functionality, enabling developers to integrate export capabilities into their web applications seamlessly.
Offering an array of customizable properties, the TableExport
library empowers users to tailor the final output according to their specific needs.
In this section, we’ll delve deeper into the capabilities of the TableExport
library, explore its usage, and dissect the provided code snippet.
Below provided code snippet offers a concise introduction to the TableExport
library’s functionality. By including the library’s source using a script
tag, developers set the stage for utilizing its features. The example showcases how to initiate the export process and customize the resulting file’s properties.
<script src="https://cdnjs.cloudflare.com/ajax/libs/TableExport/5.2.0/js/tableexport.min.js" integrity="sha512-XmZS54be9JGMZjf+zk61JZaLZyjTRgs41JLSmx5QlIP5F+sSGIyzD2eJyxD4K6kGGr7AsVhaitzZ2WTfzpsQzg==" crossorigin="anonymous" referrerpolicy="no-referrer">
TableExport(document.getElementsByTagName("table"), {
filename: 'excelfile',
sheetname: "sheet1"
});
</script>
Step-by-Step Breakdown
- Importing the Library: The foundation of this method lies in importing the
TableExport
library. By providing the library’s source using ascript
tag, developers grant access to its export capabilities. Theintegrity
attribute ensures the integrity of the library’s content, while thecrossorigin
attribute handles cross-origin policies. - Exporting the HTML Table: Within the same
script
tag, theTableExport
function is invoked. By selecting the desired HTML table using thedocument.getElementsByTagName("table")
method, developers indicate the table that should undergo the export process. - Customizing Export Properties: The provided object within the function call contains properties that customize the export process. The
filename
property determines the exported file’s name, while thesheetname
property sets the sheet’s name within the file. These properties offer valuable customization options, enhancing user experience.
Use the jQuery table2excel
Library to Export HTML Table to Excel File in JavaScript
The table2excel
plugin is a lightweight yet powerful solution built on the foundation of jQuery
. This plugin empowers developers to easily export tabular data, delivering a smooth experience for users interacting with data-rich web applications.
The below code snippet below offers an initial glimpse into the functionality of the table2excel
plugin.
By importing the essential jQuery
library using a script
tag, developers pave the way for harnessing the plugin’s capabilities.
The example demonstrates the export process and introduces the customization aspect through the provided function.
<script src="//ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js" type="text/javascript">
function Export() {
$("#table").table2excel({
filename: "file.xls"
});
}
</script>
Step-by-Step Breakdown
jQuery
Library Inclusion: The foundation of this method hinges on importing thejQuery
library. By including the library’s source within ascript
tag, developers enable the usage ofjQuery
functions and methods.- Export Trigger: The function
Export()
takes center stage in initiating the export operation. Invoked through a trigger, this function orchestrates the conversion of HTML table data to an Excel spreadsheet. - Selecting the Table: The core of the export process lies in the
$("#table")
selector. This directive identifies the specific HTML table targeted for export. - Customizing Export: The
table2excel()
method is applied to the selected table within the same function. Thefilename
property is integral to customization, determining the name of the exported Excel file. In this case, the file will be namedfile.xls
.
Use SheetJS xlsx
to Export HTML Table to Excel File in JavaScript
SheetJS is a powerful JavaScript library that allows for manipulating and converting spreadsheet file formats, including Excel. This library allows developers to effortlessly export data from HTML tables into Excel spreadsheets. Let’s dive into the step-by-step implementation of this approach.
To begin, ensure you have the SheetJS library installed. You can add it to your project using a package manager like npm:
npm install xlsx
Next, import the necessary modules in your HTML file:
<!DOCTYPE html>
<html>
<head>
<title>Export HTML Table to Excel</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/xlsx/0.17.1/xlsx.full.min.js"></script>
</head>
<body>
<table id="myTable">
<!-- Your table content here -->
</table>
<script>
// JavaScript code will go here
</script>
</body>
</html>
Now, let’s create a function to export the HTML table to an Excel file using SheetJS.
function exportTableToExcel(tableElement, filename) {
const table = document.querySelector(tableElement);
const tableData = [];
// Extract table data
table.querySelectorAll('tr').forEach(row => {
const rowData = [];
row.querySelectorAll('th, td').forEach(cell => {
rowData.push(cell.textContent);
});
tableData.push(rowData);
});
// Create worksheet
const worksheet = XLSX.utils.aoa_to_sheet(tableData);
// Create workbook
const workbook = XLSX.utils.book_new();
XLSX.utils.book_append_sheet(workbook, worksheet, 'Sheet1');
// Export the workbook to Excel file
XLSX.writeFile(workbook, filename);
}
The exportTableToExcel
function takes the HTML table element and a filename as parameters. It first extracts the table’s data into a 2D array. Then, it creates a worksheet using SheetJS’s XLSX.utils.aoa_to_sheet
method. The workbook is created by combining the worksheet, and the download is initiated using the XLSX.writeFile
method.
To trigger the export process, call the exportTableToExcel
function, passing the table element’s selector and the desired filename.
// Example usage
exportTableToExcel('#myTable', 'table_data.xlsx');