How to Convert an Image Into Base64 String Using JavaScript
-
Use
canvas
to Convert Image to Base64 String in JavaScript -
Use
FileReader
to Convert Image to Base64 String in JavaScript
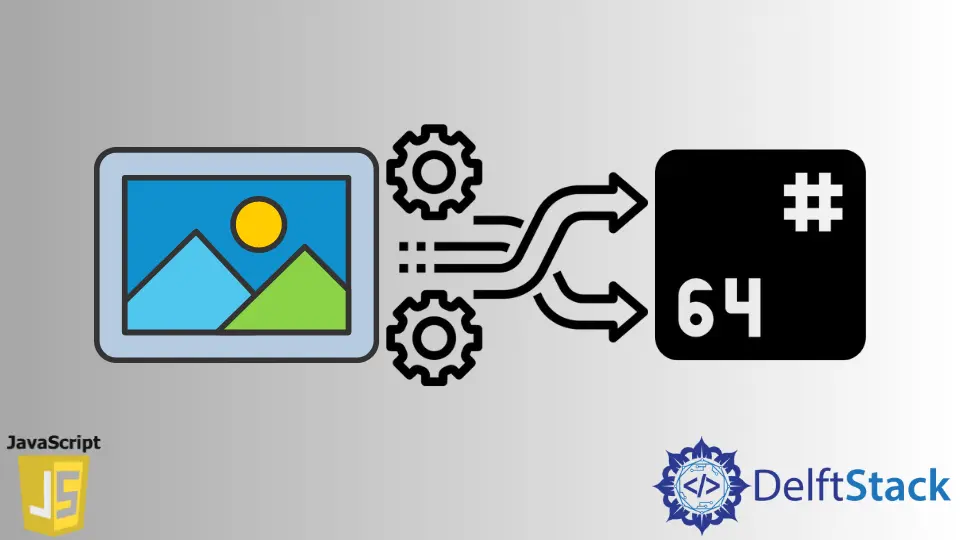
JavaScript has the convention to convert an image URL or image from a local PC to a base64 string. This string can have a wide number of symbols and letters.
We will discuss creating a canvas
element, loading the image into it, and using toDataURL
to show the string representation. We will also try the file reader
option to get the base64 string representation.
Use canvas
to Convert Image to Base64 String in JavaScript
In this case, we create a canvas
element and define its dimensions - the dataURL
where we will store the string representation.
We will add random images from online sources, and to avoid security issues, we will ensure the object.crossOrigin = 'Anonymous'
. Lastly, our callback function will take the dataURL
to the toDataURL
function to alert the window to preview the base64 string for the corresponding image.
Code Snippet:
<!DOCTYPE html>
<html lang="en">
<head>
<title>Test</title>
<style>
img{
height: 200px;
}
</style>
</head>
<body>
<img src="https://images.unsplash.com/photo-1606115915090-be18fea23ec7?ixlib=rb-1.2.1&ixid=MnwxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8&auto=format&fit=crop&w=465&q=80" id="myImg">
<script>
function toDataURL(src, callback){
var image = new Image();
image.crossOrigin = 'Anonymous';
image.onload = function(){
var canvas = document.createElement('canvas');
var context = canvas.getContext('2d');
canvas.height = this.naturalHeight;
canvas.width = this.naturalWidth;
context.drawImage(this, 0, 0);
var dataURL = canvas.toDataURL('image/jpeg');
callback(dataURL);
};
image.src = src;
}
toDataURL('https://images.unsplash.com/photo-1606115915090-be18fea23ec7?ixlib=rb-1.2.1&ixid=MnwxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8&auto=format&fit=crop&w=465&q=80', function(dataURL){
alert(dataURL);
})
</script>
</body>
</html>
Output:
Use FileReader
to Convert Image to Base64 String in JavaScript
For a file reading convention, we will dynamically initialize a new object. The object will trigger the onload
method and draw out the base64 string. Our input
element is taking images from the local computer through upload.
Code Snippet:
<!DOCTYPE html>
<html lang="en">
<head>
</head>
<body>
<input type="file" name="" id="fileId"
onchange="Uploaded()">
<br><br>
<button onclick="display()">
Display String
</button>
</body>
</html>
var base64String = '';
function Uploaded() {
var file = document.querySelector('input[type=file]')['files'][0];
var reader = new FileReader();
reader.onload = function() {
base64String = reader.result.replace('data:', '').replace(/^.+,/, '');
imageBase64Stringsep = base64String;
} reader.readAsDataURL(file);
}
function display() {
console.log('Base64String about to be printed');
alert(base64String);
}
Output:
Related Article - JavaScript Image
- How to Add Onclick Event on HTML Image Tag in JavaScript
- How to Crop an Image in JavaScript Using HTML Canvas
- How to Fade-In Image Using JavaScript
- How to Change Image on Hover in JavaScript
- How to Load Image From URL in JavaScript
- How to Swap Images in JavaScript