How to Fix Java Error Operation Not Allowed After ResultSet Closed
-
Java Error
java.sql.SQLException: Operation not allowed after ResultSet closed
-
Fix Java Error
java.sql.SQLException: Operation not allowed after ResultSet closed
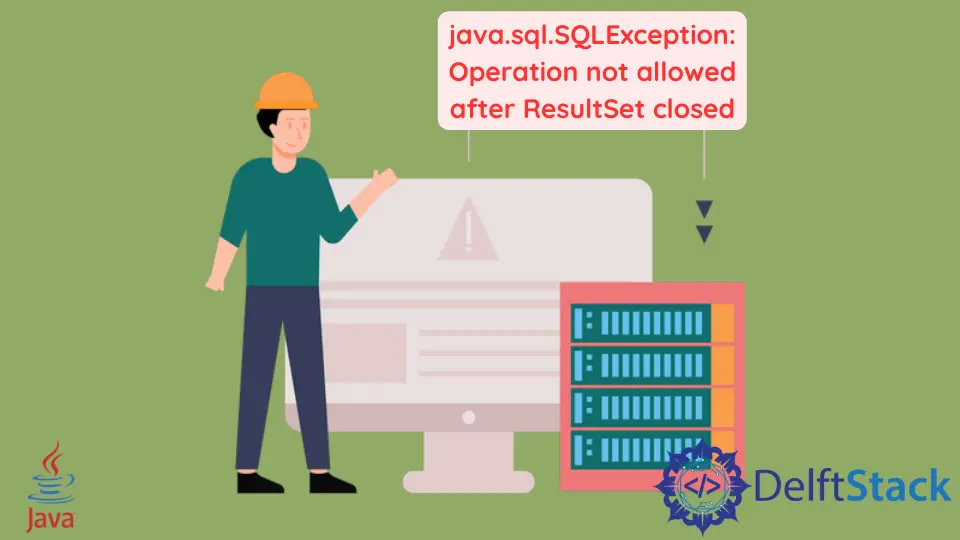
This tutorial demonstrates the java.sql.SQLException: Operation not allowed after ResultSet closed
error in Java.
Java Error java.sql.SQLException: Operation not allowed after ResultSet closed
The error Operation Not Allowed After Resultset Closed
is an SQL exception when we try to access a closed result set. As the Java Doc mentions, whenever a statement object is closed, If its Resultset
object exists, it will also be closed.
The problem with the error is that the Resultset
instance will also save the underlying statement. So when the underlying statement is closed, the Resultset
will also be closed, which throws the error.
Here’s a snippet of code that will throw the same error.
Code:
ResultSet Result_Set; // class variable
Statement Demo_Statement = null;
try {
Demo_Statement = DB_Connection.createStatement();
Result_Set = Demo_Statement.getGeneratedKeys();
} catch (Exception e) {
throw e;
} finally {
try {
if (Demo_Statement != null)
Demo_Statement.close();
} catch (SQLException e) {
throw e;
}
}
System.out.println(Result_Set.next());
The code above with a database connection will throw the following error.
java.sql.SQLException: Operation not allowed after ResultSet closed
Fix Java Error java.sql.SQLException: Operation not allowed after ResultSet closed
The problem in the code is that we cannot close the statement instance before we are done with Resultset
. Once we are done with the Resultset
, we can close both Resultset
and Statement Instance.
As we can see, we are trying to print the Boolean from Result_Set.next()
, but we are using the Resultset
after closing the statement instance, which is also closing the Resultset
.
In the code above, the fix will be not to close the statement at this place or not to use the Resultset
after closing the statement instance. It is based on your application either we can remove the Demo_Statement.close()
or the System.out.println(Result_Set.next());
statement.
Code:
ResultSet Result_Set; // class variable
Statement Demo_Statement = null;
try {
Demo_Statement = DB_Connection.createStatement();
Result_Set = Demo_Statement.getGeneratedKeys();
} catch (Exception e) {
throw e;
} finally {
try {
if (Demo_Statement != null)
Demo_Statement.close();
} catch (SQLException e) {
throw e;
}
}
We just removed the part where we are trying the use the Resultset
after the statement instance is closed. Once all the operations are done with the Resultset
, we can close both the statement instance and Resultset
.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn FacebookRelated Article - Java Error
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix the Missing Server JVM Error in Java
- How to Fix the 'No Java Virtual Machine Was Found' Error in Eclipse
- How to Fix Javax.Net.SSL.SSLHandShakeException: Remote Host Closed Connection During Handshake
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix Java.Lang.VerifyError: Bad Type on Operand Stack