Java Dictionary
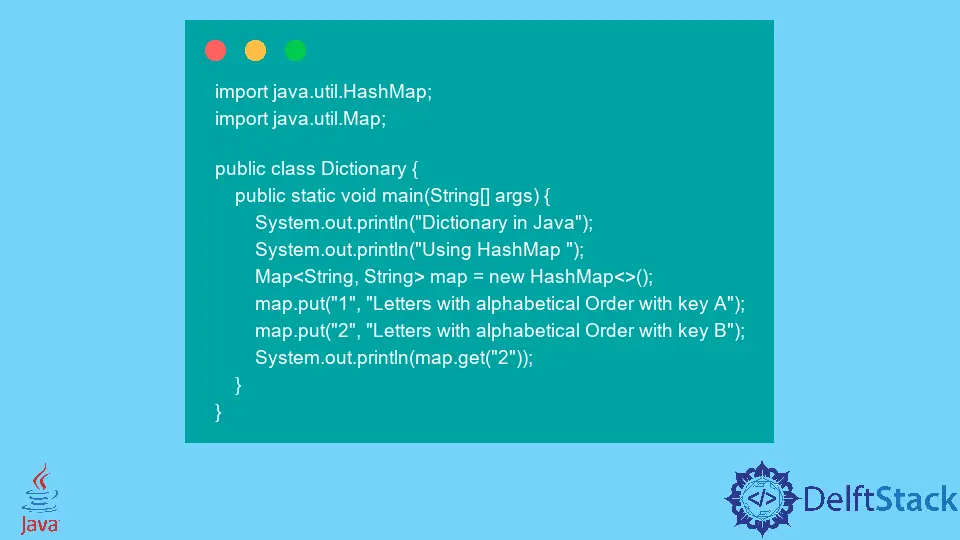
In Java language, the Dictionary gets represented using a data structure called the Map
interface. The Map
data structure gets used to present data in key-value pairs. The Map
is an interface that keeps track of all the keys and the corresponding values. A user can retrieve the value based on a unique key. The Map
interface holds the following properties:
- All the keys present in the
Map
are unique. - There can be a unique key corresponding to one or more values.
- Keys are the entities that are non-null.
Below is the code block demonstrating the use of a dictionary or Map
in Java language.
import java.util.HashMap;
import java.util.Map;
public class Dictionary {
public static void main(String[] args) {
System.out.println("Dictionary in Java");
System.out.println("Using HashMap ");
Map<String, String> map = new HashMap<>();
map.put("1", "Letters with alphabetical Order with key A");
map.put("2", "Letters with alphabetical Order with key B");
System.out.println(map.get("2"));
}
}
The Map
is an interface present separately, apart from the Collection
framework hierarchy.
The Map
is present in the java.util
package and shows how a key gets mapped to the values. There can be multiple implementations to a map, that are:
HashMap
LinkedHashMap
Hashtable
The usage of each can vary based on the users’ needs for the implementation.
In the above code snippet, the HashMap
implementation gets used. It is the default and widely used class in the Java Language. The difference between all three types is defined below.
HashMap
is introduced in Java 1.2 version, whereas Hashtable
is a legacy class. It is thread-safe, and hence concurrent operations are allowed among multiple threads, maintaining consistency in the code. But the HashMap
’s is not thread-safe and allows no concurrency.
Due to the thread-safe nature of HashMap
, it is faster in performance than the Hashtable
. Additionally, HashMap
is fail-fast and throws ConcurrencyException
when its instance gets manipulated by multiple threads at a time. In contrast to it, Hashtable
seems fail-safe. The use case of LinkedHashMap
resides when a user wants to save the insertion order.
The Map
instance gets created using the HashMap
class in the code block above. The operations like get
and put
are used to store and retrieve the specific values from the instance. The put
method takes two parameters that are the key and its value.
Internally, the key gets first evaluated if it is present in the map object using the containsKey()
function. If it evaluates to true, then the current value of the key is replaced with the new value. The method throws UnsupportedOperationException
if the defined operation gets not supported by the Map
with the given key.
The ClassCastException
if key or value prevents the key from storing, NullPointerException
if the specified key or value is null
and the Map
does not permit null
keys or values. IllegalArgumentException
if some property of the key or value prevents it from being stored in the Map
.
Once the key gets inserted into the Map
, it gets retrieved using the get
function. The get
function takes a single parameter that is the unique key. It throws ClassCastException
and NullPointerException
when the key is inappropriate or if the specified key is null
.
Below is the output of the map interface using the HashMap
class.
Dictionary in Java Using HashMap Letters with alphabetical Order with key B
Rashmi is a professional Software Developer with hands on over varied tech stack. She has been working on Java, Springboot, Microservices, Typescript, MySQL, Graphql and more. She loves to spread knowledge via her writings. She is keen taking up new things and adopt in her career.
LinkedIn