Increment Map in Java
-
Method 1: General Method for Incrementing
Map
Value in Java -
Method 2: Increment
Map
Value UsingcontainsKey()
in Java -
Method 3: Increment
Map
Value UsingAtomicInteger
orMutableInt
Class in Java
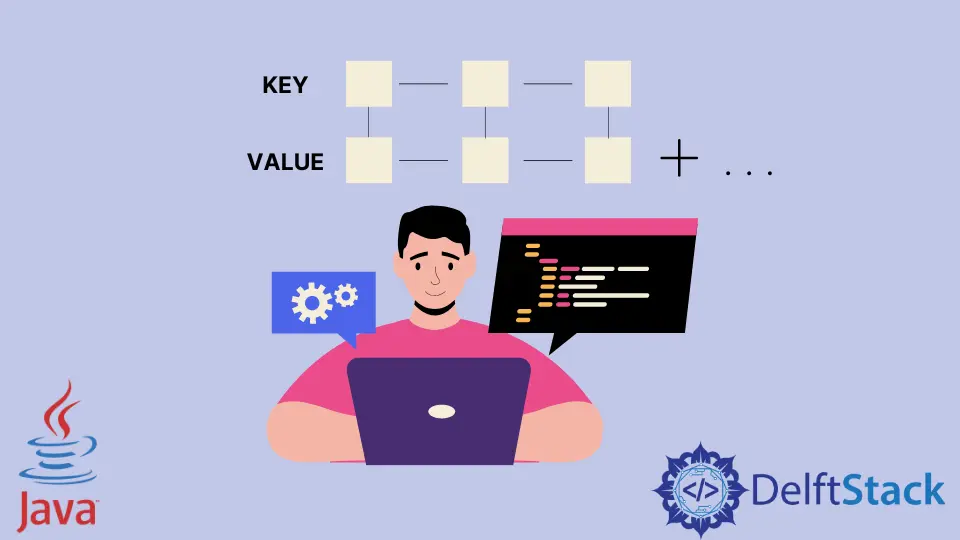
You may need to increment the value when working with Maps
or HashMaps
in Java. In Java, there are many ways to increment the Map
’s value.
In this article, we will see how we can increment a Map
value in Java, along with some necessary examples and explanations to make the topic easier.
Before we go to the example, let’s look at the basic steps required to increment a Map
value.
- To increment the value, firstly, we need to get the key of the
Map
. - After that, we need to get the current value from the
Map
. - We can easily increase the
Map
value by using any of the methods shared below.
Let’s see the example from this method.
Method 1: General Method for Incrementing Map
Value in Java
Our example below illustrates the simplest method of incrementing the Map
. Take a look at the example code.
// Importing necessary packages
import java.util.HashMap;
import java.util.Map;
// Our main class
class Main {
public static <K> void IncrementMapValue(Map<K, Integer> MyMap, K key) {
// Collecting the Map key
Integer count = MyMap.get(key);
// Checking whether the Map value is null
if (count == null) {
count = 0;
}
// increment the Map value
MyMap.put(key, count + 1);
}
public static void main(String[] args) {
Map<String, Integer> MyMap = new HashMap(); // Creating an object of Map
MyMap.put("Value-A", 100); // Putting a value for MyMap
IncrementMapValue(MyMap, "Value-A"); // Incrementing value
IncrementMapValue(MyMap, "Value-B"); // Incrementing value
System.out.println(MyMap);
}
}
We already commented on each line’s purpose, and after executing the above example code, you will get an output like the one below.
{Value-B=1, Value-A=101}
Method 2: Increment Map
Value Using containsKey()
in Java
// Importing necessary packages
import java.util.HashMap;
import java.util.Map;
// Our main class
class Main {
public static <K> void IncrementMapValue(Map<K, Integer> MyMap, K key) {
// Collecting the Map key and getting the current value of the Map
Integer count = MyMap.containsKey(key) ? MyMap.get(key) : 0;
MyMap.put(key, count + 1); // increment the Map value
}
public static void main(String[] args) {
Map<String, Integer> MyMap = new HashMap(); // Creating an object of Map
MyMap.put("Value-A", 100); // Putting a value for MyMap
IncrementMapValue(MyMap, "Value-A"); // Incrementing value
IncrementMapValue(MyMap, "Value-B"); // Incrementing value
System.out.println(MyMap);
}
}
We already commented on the purpose of each line. In the example above, the containsKey()
checks whether the Map
contains any mapping for the key.
After executing the above example code, you will get an output like the one below.
{Value-B=1, Value-A=101}
Method 3: Increment Map
Value Using AtomicInteger
or MutableInt
Class in Java
// Importing necessary packages
import java.util.HashMap;
import java.util.Map;
import java.util.concurrent.atomic.AtomicInteger;
// Our main class
class Main {
public static <K> void IncrementMapValue(Map<K, Integer> MyMap, K key) {
// Collecting the Map key and getting the current value of the Map
AtomicInteger AtomicInt = new AtomicInteger(MyMap.containsKey(key) ? MyMap.get(key) : 0);
MyMap.put(key, AtomicInt.incrementAndGet()); // increment the Map value
}
public static void main(String[] args) {
Map<String, Integer> MyMap = new HashMap(); // Creating an object of Map
MyMap.put("Value-A", 100); // Putting a value for MyMap
IncrementMapValue(MyMap, "Value-A"); // Incrementing value
IncrementMapValue(MyMap, "Value-B"); // Incrementing value
System.out.println(MyMap);
}
}
We already commented on the purpose of each line. Here, the method incrementAndGet()
is used for incrementing the Map
value.
After executing the above example code, you will get an output like the one below.
{Value-B=1, Value-A=101}
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn