How to Convert Enum to String in Java
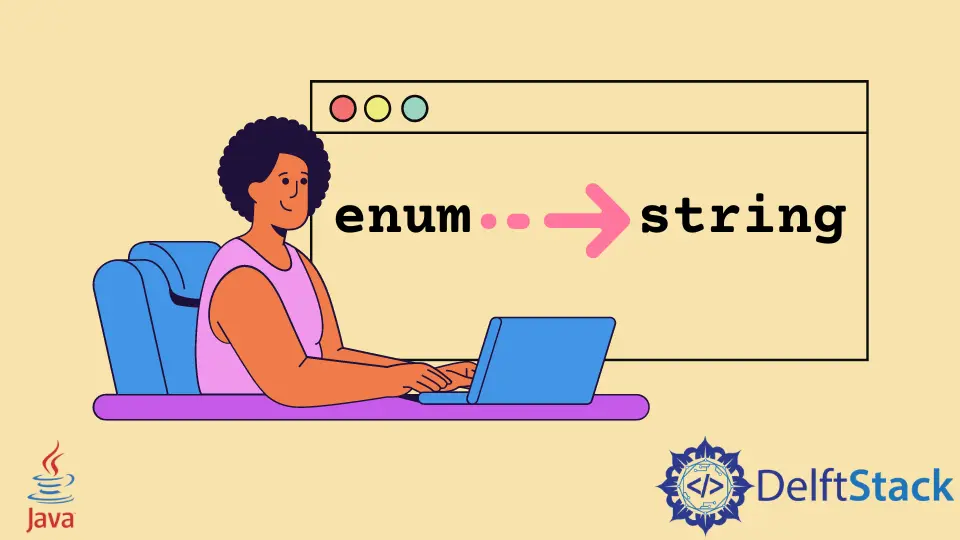
Enum in Java is a special data type or class that holds a set of constants. We can add constructors and methods in an enum, too. To create an enum in Java, we use the keyword enum
and give it a name just like a class. In this article, we will go through the ways to convert an enum to string Java.
Convert Enum to String Using name()
in Java
In the first example, we will use name()
of the Enum
class to return the enum constant’s exact name as a string. Below, we have created an enum inside the class, but we can create an enum either outside or inside it. We named the enum Directions
, which contains the names of directions as enum constant.
We can fetch any constant using the name()
method. Directions.WEST.name()
will return WEST
as a string, which is stored in the string variable getWestInString
and then print in the output.
public class EnumToString {
enum Directions {
NORTH,
SOUTH,
EAST,
WEST
}
public static void main(String[] args) {
String getWestInString = Directions.WEST.name();
System.out.println(getWestInString);
}
}
Output:
WEST
Convert Enum to String Using toString()
in Java
Just like name()
we have the toString()
method, but when it comes to using an enum constant for any important purpose, name()
is preferred because it returns the same constant while toString()
can be overridden inside the enum. It means that we can modify what is returned as a string using toString()
, which we will see in the next example.
In this example, we use the toString()
method on the constant that needs to be converted to a string.
public class EnumToString {
enum Currencies { USD, YEN, EUR, INR }
public static void main(String[] args) {
String getCurrency = Currencies.USD.toString();
System.out.println(getCurrency);
}
}
Output:
USD
We have discussed above that we can override the toString()
method to modify what we want to return as a string with the enum constant. In the example below, we have four currencies as constants calling the enum constructors with a string passed as an argument.
Whenever a constant sees a toString()
method, it will pass the string name to getCurrencyName
, a string variable. Now we have to override the toString()
method inside the enum and return the getCurrencyName
with a string.
In the main()
method, we used toString()
to get the INR
constant as string. We can see in the output that the modified string is printed. We can also print all the values of an enum using Enum.values()
, which returns an array of enum constants and then loop through every constant to print them as strings.
public class EnumToString {
enum Currencies {
USD("USD"),
YEN("YEN"),
EUR("EUR"),
INR("INR");
private final String getCurrencyName;
Currencies(String currencyName) {
getCurrencyName = currencyName;
}
@Override
public String toString() {
return "Currency: " + getCurrencyName;
}
}
public static void main(String[] args) {
String getCurrency = Currencies.INR.toString();
System.out.println("Your " + getCurrency);
Currencies[] allCurrencies = Currencies.values();
for (Currencies currencies : allCurrencies) {
System.out.println("All " + currencies);
}
}
}
Output:
Your Currency: INR
All Currency: USD
All Currency: YEN
All Currency: EUR
All Currency: INR
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedInRelated Article - Java String
- How to Perform String to String Array Conversion in Java
- How to Remove Substring From String in Java
- How to Convert Byte Array in Hex String in Java
- How to Convert Java String Into Byte
- How to Generate Random String in Java
- The Swap Method in Java