Difference Between Static and Final Variables in Java
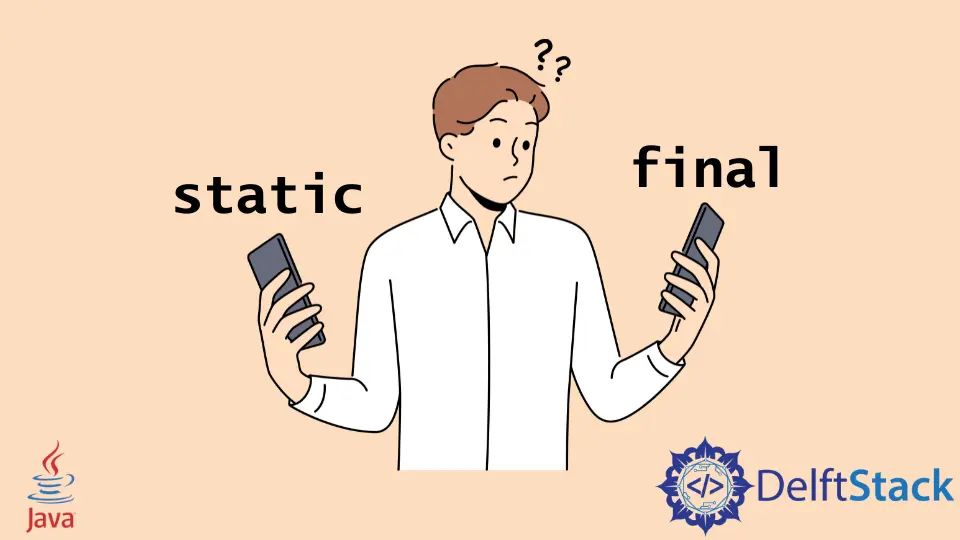
This tutorial shows the differences between static
and final
keywords. Let’s discuss the differences in a tabular format and working examples.
static |
final |
---|---|
Initialization of a static variable is not required during declaration. | It is required to initialize a final variable when it is declared. |
Static variables can be reinitialized | Final variables cannot be reinitialized. |
It is used with nested static classes, variables, methods, and blocks. | It is used with class, methods, and variables. |
Static methods can only be called by other static methods. | Final methods cannot be overridden by subclasses. |
Static Variables in Java
When we create a static variable or a method, it is attached to the class and not to the object. The variable or method will share the same reference for the whole program.
The following program has a class DummyClass
containing a static variable num
and a static
method called dummyMethod()
. To access these two members from another class, we cannot create an object of the DummyClass
because they are static.
We directly call them using the class name.
Example:
public class ExampleClass3 {
public static void main(String[] args) {
DummyClass.num = 5;
System.out.println("Static variable value: " + DummyClass.num);
DummyClass.dummyMethod();
}
}
class DummyClass {
public static int num = 0;
public static void dummyMethod() {
System.out.println("Inside Static Dummy Method");
}
}
Output:
Static variable value: 5
Inside Static Dummy Method
Nested class using static
keyword without reference to the outer class. We can create an inner class inside a class using the static
keyword.
In the below example, we have a class DummyClass
, and inside it, we create a static class called InnerClass
, and in this class, we make a method dummyMethod()
.
Now in the ExampleClass3
class, we get the InnerClass
using DummyClass.InnerClass
and create an object of the DummyClass
class and call the dummyMethod()
function.
Example:
public class ExampleClass3 {
public static void main(String[] args) {
DummyClass.InnerClass dummyClassObj = new DummyClass.InnerClass();
dummyClassObj.dummyMethod();
}
}
class DummyClass {
static class InnerClass {
void dummyMethod() {
System.out.println("Inside Static Dummy Method Of The Inner Static Class");
}
}
}
Output:
Inside Static Dummy Method Of The Inner Static Class
Final Variables in Java
A final variable cannot be modified once it is initialized. The example shows that if we create a final variable and then try to reinitialize it, we get an error in the output.
public class ExampleClass3 {
public static void main(String[] args) {
final int num = 0;
num = 1;
}
}
Output:
java: cannot assign a value to final variable num
Another class cannot extend a final
class. Using the final
keyword while creating a class restricts the class from being inherited by any other class.
Example:
public class ExampleClass3 {
public static void main(String[] args) {
new DummyClass().dummyMethod();
}
}
final class DummyClass {
public void dummyMethod() {
System.out.println("Inside Static Dummy Method");
}
}
class DummyClass2 extends DummyClass {
public void dummyMethod2() {
System.out.println("Inside Static Dummy Method 2");
}
}
Output:
java: cannot inherit from final com.tutorial.DummyClass
We cannot override a final
method in a subclass. Like a final class, if a function uses the final
keyword, it cannot be overridden by its subclasses.
public class ExampleClass3 {
public static void main(String[] args) {
new DummyClass2().dummyMethod();
}
}
class DummyClass {
public final void dummyMethod() {
System.out.println("Inside Static Dummy Method");
}
}
class DummyClass2 extends DummyClass {
public void dummyMethod() {
System.out.println("Inside Overridden Dummy Method");
}
}
Output:
java: dummyMethod() in com.tutorial.DummyClass2 cannot override dummyMethod() in com.tutorial.DummyClass
overridden method is final
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn