Background Colors in Java
- Changing Background Color in Swing
- Changing Background Color in JavaFX
- Using Color Constants and Custom Colors
- Implementing Background Colors in Layouts
- Conclusion
- FAQ
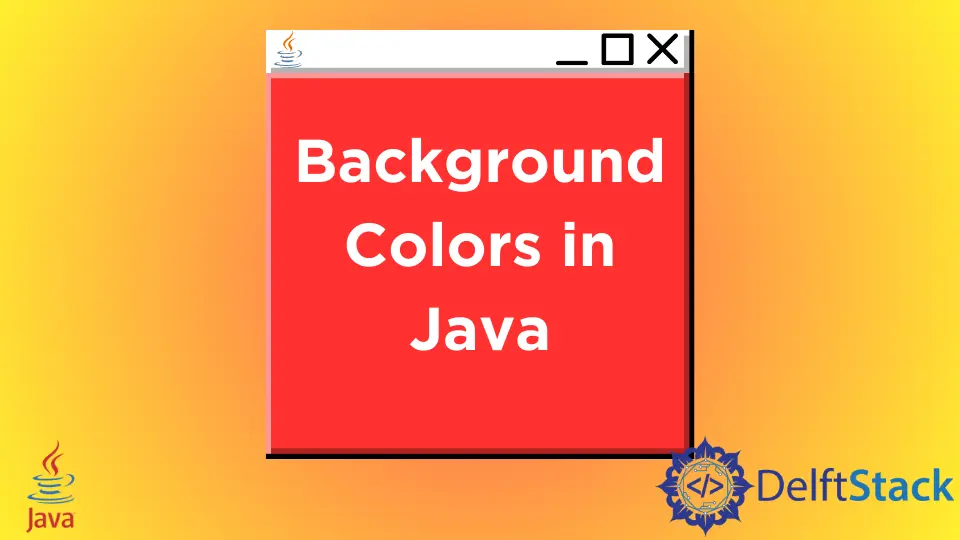
Changing background colors in Java applications can significantly enhance the user interface, making it more engaging and visually appealing. Whether you’re building a desktop application using Swing or a web application with JavaFX, understanding how to manipulate background colors is essential. In this tutorial, we will explore various methods to change background colors in Java, providing clear examples and explanations for each approach. By the end of this guide, you’ll have a solid grasp of how to implement background colors effectively in your Java projects, creating a more vibrant user experience.
Changing Background Color in Swing
Java Swing is a popular toolkit for building graphical user interfaces. One of the simplest ways to change the background color of a component is by using the setBackground
method. This method allows you to specify a color from the Color
class. Below is a straightforward example demonstrating how to change the background color of a JFrame.
import javax.swing.*;
import java.awt.*;
public class BackgroundColorExample {
public static void main(String[] args) {
JFrame frame = new JFrame("Background Color Example");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(400, 400);
frame.getContentPane().setBackground(Color.BLUE);
frame.setVisible(true);
}
}
Output:
A blue background is displayed in the JFrame.
In this example, we create a JFrame and set its default close operation. The setSize
method defines the dimensions of the window. The crucial part is the getContentPane().setBackground(Color.BLUE)
line, which changes the background color of the JFrame’s content pane to blue. Finally, we make the frame visible. You can experiment with different colors by replacing Color.BLUE
with other color constants or creating custom colors using new Color(r, g, b)
.
Changing Background Color in JavaFX
JavaFX is another powerful framework for building graphical user interfaces in Java. Changing the background color in JavaFX is just as straightforward. You can utilize the setStyle
method to apply CSS-like styling to your nodes. Here’s an example of how to change the background color of a JavaFX application.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class BackgroundColorJavaFX extends Application {
@Override
public void start(Stage primaryStage) {
StackPane root = new StackPane();
root.setStyle("-fx-background-color: green;");
Scene scene = new Scene(root, 400, 400);
primaryStage.setTitle("JavaFX Background Color Example");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Output:
A green background is displayed in the JavaFX window.
In this JavaFX example, we create a StackPane
and apply a background color using the setStyle
method. The style string -fx-background-color: green;
is used to set the background color to green. We then create a scene with the specified dimensions and set it on the primary stage. Finally, we make the stage visible. You can easily change the color by modifying the style string to any valid CSS color value.
Using Color Constants and Custom Colors
In both Swing and JavaFX, you have the flexibility to use predefined color constants or create custom colors. Java’s Color
class provides a variety of constants like Color.RED
, Color.GREEN
, and Color.YELLOW
. If you need a specific shade, you can create a custom color by specifying its RGB values.
Here’s how you can create a custom color in Swing:
import javax.swing.*;
import java.awt.*;
public class CustomColorExample {
public static void main(String[] args) {
JFrame frame = new JFrame("Custom Color Example");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(400, 400);
Color customColor = new Color(128, 0, 128); // Purple
frame.getContentPane().setBackground(customColor);
frame.setVisible(true);
}
}
Output:
A purple background is displayed in the JFrame.
In this example, we create a custom color by instantiating the Color
class with RGB values. The RGB values (128, 0, 128) correspond to a purple color. This custom color is then set as the background of the JFrame. Similarly, you can apply this technique in JavaFX by using the Color.rgb(red, green, blue)
method.
Implementing Background Colors in Layouts
When working with layouts, changing the background color of individual components can enhance the overall design. In Swing, you can set the background color of specific components like buttons, panels, or labels. Here’s an example of changing the background color of a JPanel.
import javax.swing.*;
import java.awt.*;
public class PanelBackgroundExample {
public static void main(String[] args) {
JFrame frame = new JFrame("Panel Background Example");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(400, 400);
JPanel panel = new JPanel();
panel.setBackground(Color.ORANGE);
frame.add(panel);
frame.setVisible(true);
}
}
Output:
An orange background is displayed in the JPanel.
In this example, we create a JPanel and set its background color to orange using the setBackground
method. This panel is then added to the JFrame. You can use similar techniques in JavaFX by applying background colors to specific nodes within a layout, allowing for a more structured and visually appealing interface.
Conclusion
Changing background colors in Java is a simple yet powerful way to enhance your application’s user interface. Whether you are using Swing or JavaFX, you have various methods at your disposal to customize the appearance of your components. From using predefined colors to creating custom shades, the possibilities are endless. By following the examples provided in this guide, you can easily implement background color changes in your Java projects, making your applications more engaging and visually appealing.
FAQ
-
How do I change the background color of a JFrame in Java?
You can change the background color of a JFrame by using thegetContentPane().setBackground(Color.colorName)
method. -
Can I use custom colors in Java Swing?
Yes, you can create custom colors using thenew Color(r, g, b)
constructor, where r, g, and b are the red, green, and blue color values. -
How do I apply a background color in JavaFX?
In JavaFX, you can use thesetStyle
method with a CSS-like syntax, e.g.,root.setStyle("-fx-background-color: colorName;")
. -
Are there predefined color constants in Java?
Yes, Java provides several predefined color constants in thejava.awt.Color
class, such asColor.RED
,Color.BLUE
, andColor.GREEN
. -
Can I change the background color of individual components in Java?
Yes, you can set the background color of individual components like JPanel, JButton, and more using thesetBackground
method in Swing orsetStyle
in JavaFX.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook