How to Concatenate Two Slices in Go
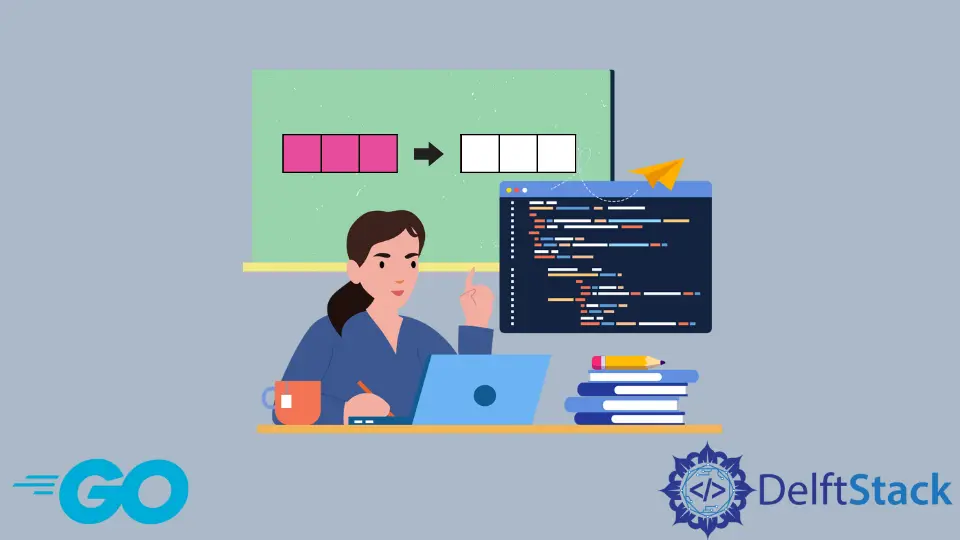
In Go, a slice
is the dynamic data structure that stores multiple elements of the same data type. They can also be thought of as variable-sized arrays that have indexing as of array but their size is not fixed as they can be resized. As slices are dynamic in nature we can append a new element to slice or concatenate two or more slices using the append
function.
Declaration of Slice
The declaration of a Slice
is similar to the declaration of arrays, but the size is not specified.
package main
import "fmt"
func main() {
var Slice1 = []int{1, 2, 3, 4}
fmt.Printf("Length of Slice: %v\n", len(Slice1))
fmt.Printf("First Element: %v\n", Slice1[0])
fmt.Printf("Second Element: %v\n", Slice1[1])
fmt.Printf("Third Element: %v\n", Slice1[2])
fmt.Printf("Fourth Element: %v\n", Slice1[3])
}
Output:
Length of Slice: 4
First Element: 1
Second Element: 2
Third Element: 3
Fourth Element: 4
Concatenation of Slices
We use the built-in append()
function to add elements to list or concatenate two or more slices together. If there is sufficient capacity, the destination is re-sliced to accommodate the new elements. If no sufficient capacity is available, a new underlying is allocated and contents are copied over. Finally, the append
function returns the updated slice.
Append a Single Element to Slice
package main
import "fmt"
func main() {
var slice_1 = []int{1, 2}
slice_2 := append(slice_1, 3)
fmt.Printf("slice_1: %v\n", slice_1)
fmt.Printf("slice_2: %v\n", slice_2)
}
Output:
slice_1: [1 2]
slice_2: [1 2 3]
Append Multiple Elements to a Slice
package main
import "fmt"
func main() {
var slice_1 = []int{1, 2}
slice_2 := append(slice_1, 3)
slice_3 := append(slice_2, 4, 5, 6)
fmt.Printf("slice_3: %v\n", slice_3)
}
Output:
slice_3: [1 2 3 4 5 6]
Concatenate Two Slices
package main
import "fmt"
func main() {
var slice_3 = []int{1, 2, 3, 4, 5, 6}
var slice_4 = []int{7, 8}
slice_5 := append(slice_3, slice_4...)
fmt.Printf("slice_4: %v\n", slice_4)
fmt.Printf("slice_5: %v\n", slice_5)
}
Output:
slice_4: [7 8]
slice_5: [1 2 3 4 5 6 7 8]
An important point to be noticed while concatenating two slices in a go is that ...
is essential after slice_4
as append()
is a variadic function, just like other variadic functions. ...
lets you pass multiple arguments to a function from a slice. Here append
function is called recursively for each element in slice_4
.
Append a String to a Byte Slice
var slice []byte = append([]byte("abc "), "def"...)
fmt.Printf("slice: %v\n", slice)
Output:
slice: [97 98 99 32 100 101 102]
Here the characters are encoded in UTF-8 using 3 bytes which represent ASCII value of characters. The slice byte can be converted back to string as:
slice_str := string(slice)
fmt.Printf("String: %v\n", slice_str)
Output:
Slice: abc def
Working of Go append()
Function
The append()
built-in function takes a slice, appends all the elements to the end of an input slice and finally returns the concatenated slice. If the destination slice has sufficient capacity, the slice is re-sliced to accommodate the new appended elements. But if the destination does not have sufficient capacity, a new array is created and the elements present in the existing slices are copied into the new array and finally, a new array is returned by the function.
package main
import "fmt"
func main() {
slice_1 := []int{0, 1, 2}
fmt.Println("slice_1 : ", slice_1)
fmt.Println("Capacity of slice_1: ", cap(slice_1))
res_1 := append(slice_1, 3)
fmt.Println("res_1 : ", res_1)
fmt.Println("Capacity of res_1: ", cap(res_1))
slice_2 := make([]int, 3, 5) //Create slice_1 with length 3 and capacity 5
copy(slice_2, []int{0, 1, 2}) //Copy elements into slice_1
fmt.Println("slice_2 : ", slice_2)
fmt.Println("Capacity of slice_2: ", cap(slice_2))
res_2 := append(slice_2, 3)
fmt.Println("res_2 : ", res_2)
fmt.Println("Capacity of res_2: ", cap(res_2))
}
Output:
slice_1 : [0 1 2]
Capacity of slice_1: 3
res_1 : [0 1 2 3]
Capacity of res_1: 6
slice_2 : [0 1 2]
Capacity of slice_2: 5
res_2 : [0 1 2 3]
Capacity of res_2: 5
Here slice_1
doesn’t have enough capacity to append new elements so a new slice of capacity 6 is created and all elements in existing slices are copied to newly created slice.
However, slice_2
has enough capacity to append new elements and hence elements are appended in the existing slice.
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn