How to Merge Two Maps in Golang
-
Merge Two Maps in Golang Using the
Copy
Method - Merge Two Maps in Golang Using a User-Defined Method for Union
-
Merge Two Maps in Golang Using a
for
Loop - Merge Two Maps in Golang Using Generics (Golang 1.18+)
- Conclusion
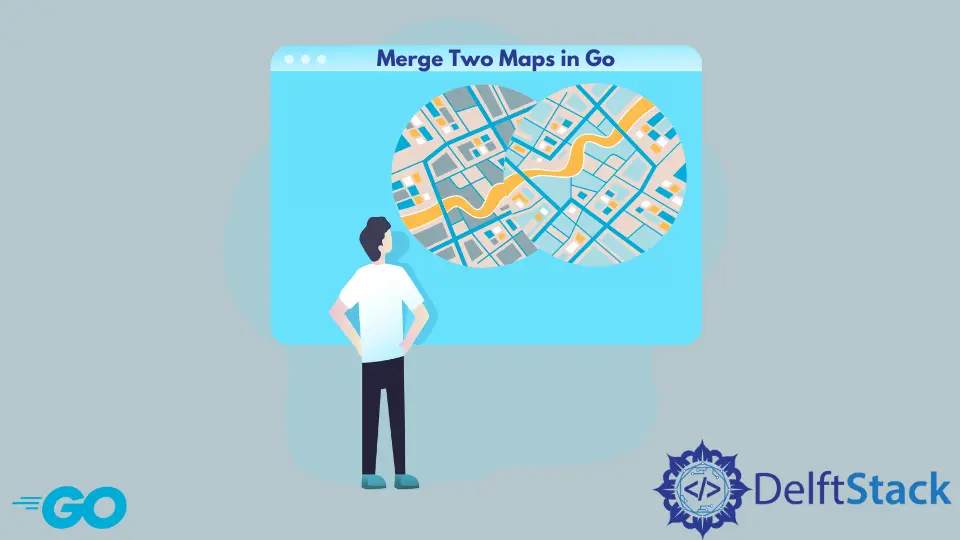
Merging two maps is a common operation in Golang, often encountered when combining data from multiple sources or consolidating information. While Golang lacks a built-in method for merging maps, developers have multiple approaches at their disposal.
In this article, we will explore various methods to merge two maps in Golang, discussing the use of the Copy
method, a user-defined method for union, a simple for
loop, and the application of generics. Each method offers its own strengths, providing you with the flexibility to choose an approach that aligns with your specific needs.
Merge Two Maps in Golang Using the Copy
Method
While the Go language doesn’t offer a built-in method for merging two maps, the Copy
method in the maps
package can be utilized to achieve this functionality.
The Copy
method in the maps
package, introduced in Golang version 1.21, provides a straightforward way to merge the contents of one map into another. It takes two parameters, which are maps, and copies the content of the second map into the first map.
While this method is convenient, it’s essential to note that it modifies the first map in the process.
The Copy
method from this package has the following syntax:
maps.Copy(dst, src)
Here, dst
represents the destination map where the content will be copied, and src
represents the source map from which the content will be copied.
The Copy
method copies the key-value pairs from the source map to the destination map. If there are overlapping keys between the two maps, the values from the source map will overwrite those in the destination map.
It’s important to note that the maps
package is an experimental package, and its features might not be stable or included in the standard library. If you intend to use this package, make sure to check the documentation for the specific version you are using.
Now, let’s see a code example demonstrating the use of the Copy
method to merge two maps.
package main
import (
"fmt"
"maps"
)
func main() {
// Define two maps to be merged
map1 := map[string]int{
"DelftstackOne": 10,
"DelftstackTwo": 20,
"DelftstackThree": 30,
}
map2 := map[string]int{
"DelftstackTwo": 20,
"DelftstackThree": 30,
"DelftstackFour": 40,
"DelftstackFive": 50,
}
// Display the maps before merging
fmt.Println("The First Map: ", map1)
fmt.Println("The Second Map: ", map2)
// Use the Copy method to merge the maps
maps.Copy(map1, map2)
// Display the merged map
fmt.Println("The Merged Map: ", map1)
}
In this example, we start by defining two maps, map1
and map2
, that we want to merge. We then print the content of both maps before the merging process using fmt.Println
.
The key action occurs when we call maps.Copy(map1, map2)
, which copies the content of map2
into map1
. The merged map is subsequently printed using fmt.Println
.
It’s important to understand that the Copy
method modifies the destination map (map1
in this case) by adding the content of the source map (map2
). If there are overlapping keys between the two maps, the values from map2
will overwrite those in map1
.
The output of the program will be:
This output demonstrates the successful merging of the two maps using the Copy
method. The resulting map, map1
, now contains the combined key-value pairs from both maps.
The Copy
method simplifies the process of merging maps in Golang by providing a clean and efficient way to combine the contents of two maps into a single, unified map. This can be especially handy in scenarios where you need to aggregate data from multiple sources or configurations.
Merge Two Maps in Golang Using a User-Defined Method for Union
While Golang’s maps
package provides a convenient Copy
method for merging maps, it’s also possible to create a custom method for union operations. This approach allows for more fine-grained control and customization.
The approach involves designing a method named MapUnion
that takes two maps, map1
and map2
, and merges them based on their union of values. In other words, entries with the same key will have their values summed up.
This method is flexible and allows customization based on specific requirements. The syntax is as follows:
func MapUnion(map1, map2 EmployeeMaps) EmployeeMaps {
// Implementation
}
Where:
map1
: The first map to be merged.map2
: The second map to be merged.EmployeeMaps
: The type of maps representing a map with integer keys and struct values.
Let’s explore a practical example demonstrating the use of a user-defined method for merging maps.
package main
import "fmt"
type Delftstack struct {
Id int
Name string
Salary int
}
type EmployeeMaps map[int]Delftstack
// Method to merge maps based on union, excluding entries with the same value
func MapUnion(map1, map2 EmployeeMaps) EmployeeMaps {
for x, y := range map1 {
if m, n := map2[x]; n {
y.Salary += m.Salary
}
map2[x] = y
}
return map2
}
func main() {
// Define two maps to be merged
map1 := EmployeeMaps{2: {30, "Jack", 2000}, 5: {50, "Samuel", 1500}, 6: {60, "John", 1800}}
map2 := EmployeeMaps{1: {10, "Pamela", 3000}, 2: {20, "Connor", 2000}, 3: {30, "Jack", 2000}, 4: {40, "Joe", 4000}}
// Merge maps using the user-defined method
result := MapUnion(map2, map1)
fmt.Println(result)
}
In this example, we’ve defined two maps, map1
and map2
, each representing an EmployeeMaps
type with integer keys and Delftstack
struct values. The MapUnion
method is then called with these two maps as parameters.
Within the MapUnion
method, a for
loop iterates over the entries in map1
. For each entry, it checks if the corresponding key exists in map2
.
If it does, the salaries of the two entries are added, effectively merging the maps based on the union of values. The modified map2
is then returned.
The output of the program will be:
This output demonstrates the successful merging of the two maps using the user-defined method MapUnion
. The resulting map, result
, contains the combined key-value pairs from both maps and entries with the same key have their values summed up.
This user-defined method for merging maps provides flexibility in customization. You can easily modify the merging logic to accommodate specific requirements, such as handling conflicts or applying transformations to values during the merge process.
It serves as a versatile alternative to the Copy
method for scenarios where more control is desired over the merging behavior.
Merge Two Maps in Golang Using a for
Loop
Merging two maps can also be accomplished using a straightforward for
loop, where each key-value pair from one map is added to another. This method provides a clear and explicit approach to map merging.
Typically, the for
loop syntax for merging maps involves iterating through the key-value pairs of one map and copying them into another. The basic structure is as follows:
for key, value := range sourceMap {
destinationMap[key] = value
}
Here, sourceMap
is the map from which we are copying, and destinationMap
is the map to which we are copying the key-value pairs.
Take a look at the following example demonstrating the use of a for
loop to merge two maps.
package main
import "fmt"
func mergeMaps(map1, map2 map[string]int) map[string]int {
// Iterate through map1 and add key-value pairs to map2
for key, value := range map1 {
map2[key] = value
}
return map2
}
func main() {
// Define two maps to be merged
map1 := map[string]int{
"DelftstackOne": 10,
"DelftstackTwo": 20,
"DelftstackThree": 30,
}
map2 := map[string]int{
"DelftstackTwo": 20,
"DelftstackThree": 30,
"DelftstackFour": 40,
"DelftstackFive": 50,
}
// Display the maps before merging
fmt.Println("The First Map: ", map1)
fmt.Println("The Second Map: ", map2)
// Use a for loop to merge the maps
mergedMap := mergeMaps(map1, map2)
// Display the merged map
fmt.Println("The Merged Map: ", mergedMap)
}
In this example, we’ve defined two maps, map1
and map2
, representing maps with string keys and integer values. The mergeMaps
function takes two maps as parameters and uses a for
loop to iterate through map1
.
For each key-value pair in map1
, the corresponding key is added to map2
along with its associated value. The result is a merged map, and this map is then printed.
The main
function demonstrates the process by displaying the content of both maps before merging, invoking the mergeMaps
function, and finally printing the resulting merged map.
The output of the program will be:
This output confirms the successful merging of the two maps using a for
loop. The resulting map, mergedMap
, now contains the combined key-value pairs from both maps.
Merging maps using a for
loop provides a straightforward and explicit way to combine map contents. It offers fine-grained control over the merging process and can be easily customized to handle specific scenarios, such as resolving conflicts or applying transformations during the merge.
While it may require more manual effort compared to the Copy
method or user-defined functions, it remains a reliable and widely-used approach in Golang.
Merge Two Maps in Golang Using Generics (Golang 1.18+)
With the introduction of generics in Golang in its 1.18 version, the process of merging two maps becomes even more versatile and expressive. Generics allow developers to write functions and data structures that can work with different types.
Generics in Golang allow us to create functions that can work with different data types without sacrificing type safety. The function for merging two maps using generics is designed to be flexible and reusable.
The syntax for a generic function to merge two maps looks like this:
func mergeMaps[T, U any](map1 map[T]U, map2 map[T]U) map[T]U {
for key, value := range map1 {
map2[key] = value
}
return map2
}
Where:
T
: The type of keys in the maps.U
: The type of values in the maps.map1
: The first map to be merged.map2
: The second map to be merged.
Here’s an example:
package main
import "fmt"
func mergeMaps[T comparable, U any](map1 map[T]U, map2 map[T]U) map[T]U {
for key, value := range map1 {
map2[key] = value
}
return map2
}
func main() {
// Define two maps to be merged
map1 := map[string]int{
"DelftstackOne": 10,
"DelftstackTwo": 20,
"DelftstackThree": 30,
}
map2 := map[string]int{
"DelftstackTwo": 20,
"DelftstackThree": 30,
"DelftstackFour": 40,
"DelftstackFive": 50,
}
// Display the maps before merging
fmt.Println("The First Map: ", map1)
fmt.Println("The Second Map: ", map2)
// Use the generic function to merge the maps
mergedMap := mergeMaps(map1, map2)
// Display the merged map
fmt.Println("The Merged Map: ", mergedMap)
}
In this example, the mergeMaps
function is defined with generic types T
and U
for keys and values, respectively. The function takes two maps, map1
and map2
, and iterates through map1
using a for
loop. For each key-value pair in map1
, it is added to map2
. The resulting merged map, map2
, is then returned.
The main
function demonstrates the process by displaying the content of both maps before merging, invoking the mergeMaps
function with string keys and integer values, and finally printing the resulting merged map.
The output of the program will be:
This output confirms the successful merging of the two maps using a generic function. The resulting map, mergedMap
, now contains the combined key-value pairs from both maps.
Generics in Golang enable us to write more flexible and reusable code. The MergeMaps
function, thanks to generics, can merge maps of different types, providing a type-safe solution for map merging.
This approach is particularly beneficial when dealing with maps containing various key and value types across different parts of the application.
Conclusion
Merging two maps in Golang can be achieved through various methods, each offering its own set of advantages. The Copy
method, a user-defined method for union, a simple for
loop, and the use of generics provide developers with flexibility in choosing an approach that aligns with their specific requirements.
The Copy
method, found in the maps
package, is convenient for a straightforward merge, but it modifies the original map. The user-defined method for union allows customization, excluding entries with the same value.
A simple for
loop provides explicit control, allowing developers to manage the merging process efficiently. Finally, the introduction of generics in Golang 1.18 empowers developers to create a generic function capable of merging maps of different types while maintaining type safety.
Ultimately, the choice of method depends on the particular needs of the task at hand. Golang’s simplicity and flexibility provide developers with a range of options to merge maps, ensuring they can select the method that best fits their use case.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook