How to Create Constructors in Golang
-
Use the
init
Function to Create Constructors in Golang -
Use the
struct
Function to Create Constructors in Golang - Use the Factory Function to Create Constructors in Golang
- Conclusion
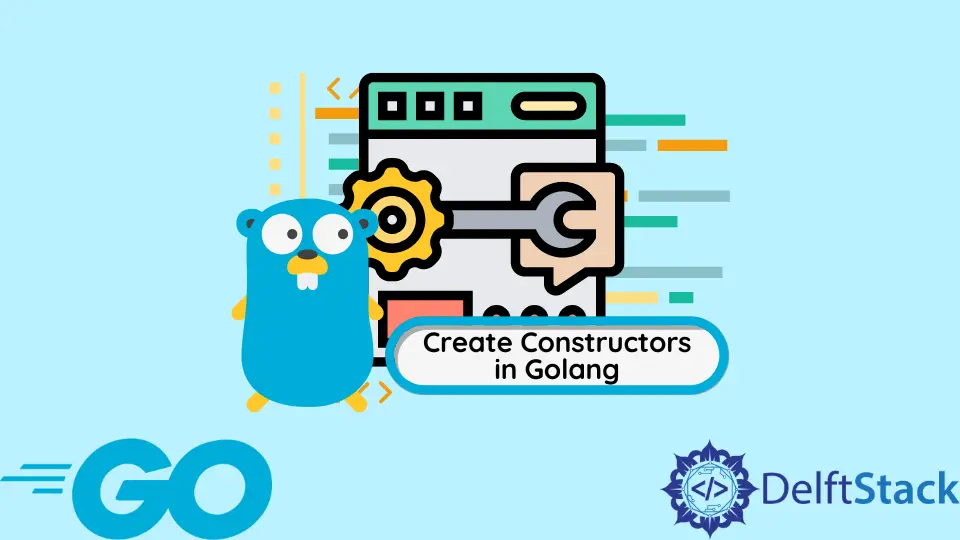
Go is not an object-oriented language in the same sense that Java is. Constructors aren’t a standard language feature.
When developing Go programs, you should construct modular components that are only weakly tied to one another. This article will tackle creating constructors in Golang using different functions.
Use the init
Function to Create Constructors in Golang
There are no default constructors in Go, but you can define functions for any type. The init
function is a special function that is called automatically when a package is initialized.
It is not used to create constructors in the traditional sense, but it can be leveraged to perform package-level initialization tasks. We will create constructors using the init
function in this example.
Code:
package main
import "fmt"
type Thing struct {
Name string
Num int
}
func (t *Thing) Init(name string, num int) {
t.Name = name
t.Num = num
}
func main() {
t := new(Thing)
t.Init("Hello Boss!", 99)
fmt.Printf("%s: %d\n", t.Name, t.Num)
}
In this code, we define a Thing
struct with two fields, Name
and Num
. The struct has a receiver function, Init
, which sets the values of these fields based on the parameters provided.
In the main
function, we create a new instance of the Thing
struct using the new
keyword and subsequently initialize it with the Init
function, setting the name to "Hello Boss!"
and the number to 99
.
Finally, we print the values of the Name
and Num
fields using fmt.Printf
.
Output:
Hello Boss!: 99
The output of this program will be Hello Boss!: 99
, showcasing the successful initialization of the Thing
struct and the printing of its updated values.
Keep in mind that the init
function is automatically called by the Go runtime before main
is executed, and there can be multiple init
functions in a package (they run in the order in which they are declared). It’s generally used for global setup tasks rather than creating instances of types.
For creating instances, factory functions or struct
functions are more appropriate in Go, which will be discussed in the following sections.
Use the struct
Function to Create Constructors in Golang
There isn’t a concept of a traditional constructor method associated with a struct
like in some other object-oriented languages. Instead, Go encourages the use of factory functions or initializer methods to achieve similar results.
There isn’t a direct equivalent to a constructor method associated with a struct. However, you can create a function that returns an instance of a struct with some initial configuration, and this function can serve a similar purpose as a constructor.
We’re declaring the employee’s name and age using this example’s struct
function.
Code 1:
package main
import "fmt"
type Employee struct {
Name string
Age int
}
func (e *Employee) Init(name string, age int) {
e.Name = name
e.Age = age
}
func main() {
emp := new(Employee)
emp.Init("Jay Singh", 23)
fmt.Printf("%s: %d\n", emp.Name, emp.Age)
}
In this code, we define an Employee
struct with two fields, Name
and Age
. We also include a receiver function, Init
, which allows us to initialize the struct’s fields with specific values.
In the main
function, we create a new instance of the Employee
struct using the new
keyword. We then call the Init
function on this instance, setting the name to "Jay Singh"
and the age to 23
.
Finally, we use fmt.Printf
to print the values of the Name
and Age
fields.
Output:
Jay Singh: 23
The output of this program will be Jay Singh: 23
, indicating the successful creation and initialization of the Employee
struct, with the printed values reflecting the specified name and age.
Another example is the newUser
constructor method in the example, which produces a new User
struct. The function returns a pointer to the newly created struct.
Code 2:
package main
import "fmt"
type User struct {
firstName string
lastName string
email string
}
func newUser(firstName string, lastName string, email string) *User {
user := User{firstName, lastName, email}
return &user
}
func main() {
user := newUser("Jay", "Singh", "jayexamplesingh@gmail.com")
fmt.Println("firstName:", user.firstName)
fmt.Println("lastName:", user.lastName)
fmt.Println("email:", user.email)
}
In this code, we define a User
struct with three fields: firstName
, lastName
, and email
. We create a newUser
function, acting as a constructor, which takes parameters for each field and returns a pointer to a newly initialized User
struct.
In the main
function, we use this constructor to create a new user instance with the values "Jay"
for the first name, "Singh"
for the last name, and "jayexamplesingh@gmail.com"
for the email.
We then print the user’s details using fmt.Println
, displaying the first name, last name, and email.
Output:
firstName: Jay
lastName: Singh
email: jayexamplesingh@gmail.com
This output reflects the successful creation and initialization of the User
struct, demonstrating the provided values for each field.
Use the Factory Function to Create Constructors in Golang
In Go, a factory function is a function that returns an instance of a struct
, typically used to initialize and configure the struct. The factory functions serve a similar purpose by encapsulating the logic of creating and initializing objects.
Code:
package main
import "fmt"
type Employee struct {
Name string
Age int
}
func NewEmployee(name string, age int) *Employee {
return &Employee{
Name: name,
Age: age,
}
}
func main() {
emp := NewEmployee("Jay Singh", 23)
fmt.Printf("%s: %d\n", emp.Name, emp.Age)
}
In this code, we define an Employee
struct with two fields: Name
and Age
. We implement a factory function, NewEmployee
, which takes parameters for each field and returns a pointer to a newly created and initialized Employee
struct.
In the main
function, we utilize this factory function to create a new employee instance with the values "Jay Singh"
for the name and 23
for the age.
We then print the employee’s details using fmt.Printf
, displaying the name and age.
Output:
Jay Singh: 23
This output signifies the successful use of the factory function to create and initialize the Employee
struct, demonstrating the specified values for each field.
By using factory functions, you can encapsulate the initialization logic and provide a clean interface for creating instances of your structs. This is a common practice in Go for achieving similar functionality to constructors in other programming languages.
Remember, Go doesn’t have constructors like languages such as Java or C++. Instead, it emphasizes simplicity and clarity through other means, like explicit initialization using functions or methods.
Conclusion
In conclusion, Go, not being an object-oriented language in the traditional sense, lacks standard constructors. Instead, it promotes the creation of modular, loosely coupled components.
This article explores different methods for creating constructors in Golang. The init
function, a special function called during package initialization, can be leveraged for package-level tasks, though it’s not used as a traditional constructor.
Struct functions or initializer methods, exemplified with an Employee
struct, provide an alternative to traditional constructors. These functions return instances of structs with specific configurations.
Additionally, factory functions, such as NewEmployee
, offer a clean interface for creating and initializing struct instances, aligning with Go’s emphasis on simplicity and clarity.
In summary, Go encourages the use of functions, such as init
for package-level tasks and factory functions for struct initialization, to achieve object creation in a modular and straightforward manner.