How to Resize an Image in C#
-
Resize an Image With the
Bitmap
Class inC#
-
Resize an Image With the
Graphics.DrawImage()
Function inC#
- Conclusion
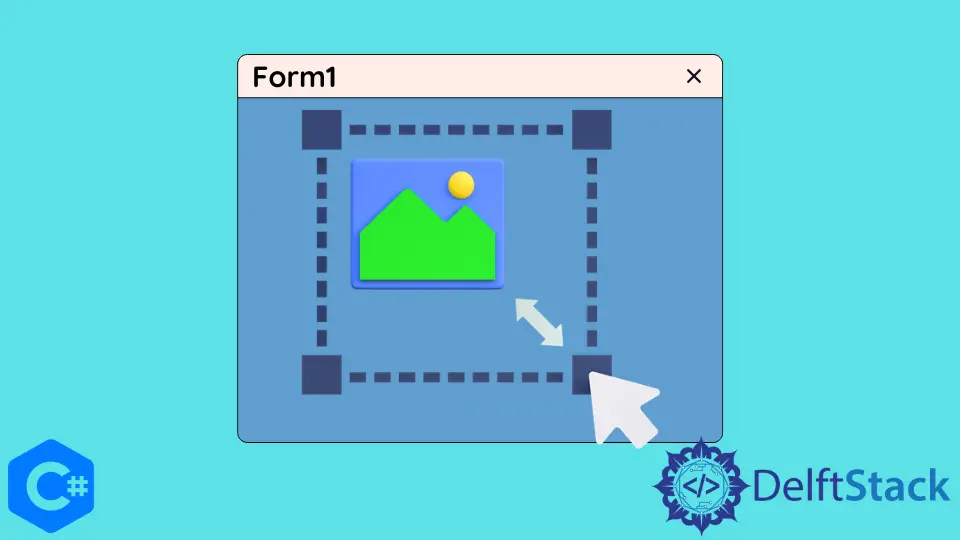
In this tutorial, we will discuss methods to resize an image in C#.
We will take you through the entire process, from loading the original image to saving the resized version.
Resize an Image With the Bitmap
Class in C#
The Bitmap
class provides many methods to work with images in C#. The Bitmap
class gets the pixel data of images. We can resize an image by initializing the Size
parameter inside the constructor of the Bitmap
class.
The following code example shows us how we can resize an image with the constructor of the Bitmap
class in C#.
using System;
using System.Drawing;
namespace resize_image {
class Program {
public static Image resizeImage(Image imgToResize, Size size) {
return (Image)(new Bitmap(imgToResize, size));
}
static void Main(string[] args) {
string path = "C:\\Images\\img1.jpg";
Image img = Image.FromFile(path);
Bitmap imgbitmap = new Bitmap(img);
Image resizedImage = resizeImage(imgbitmap, new Size(200, 200));
}
}
}
This code demonstrates the complete process of resizing an image using the Bitmap
class in C#. Let’s break down how it works.
Step 1: Load the Original Image
First, you need to load the original image using the Image.FromFile
method. Make sure to replace "C:\\Images\\img1.jpg"
with the path to your own image file.
string path = "C:\\Images\\img1.jpg";
Image img = Image.FromFile(path);
Step 2: Convert the Image to a Bitmap
Next, you convert the loaded image to a Bitmap
object. This step is necessary to perform the resizing operation.
Bitmap imgBitmap = new Bitmap(img);
Step 3: Resize the Image
Now comes the resizing part. The ResizeImage
function takes the Bitmap
object and the desired size (new Size(200, 200)
) and returns the resized image.
Image resizedImage = ResizeImage(imgBitmap, new Size(200, 200));
Step 4: Save the Resized Image
Finally, you can save the resized image to a file using the Save
method. In this example, the resized image is saved as "resized.jpg"
. You can choose the format of the saved image by changing the file extension (e.g., .jpg
, .png
, .bmp
).
resizedImage.Save("resized.jpg");
Resize an Image With the Graphics.DrawImage()
Function in C#
The Graphics.DrawImage()
function draws an image inside a specified location with specified dimensions in C#. With this method, we can eliminate many drawbacks of resizing an image. The following code example shows us how we can resize an image with the Graphics.DrawImage()
function in C#.
using System;
using System.Drawing;
namespace resize_image {
class Program {
public static Image resizeImage(Image image, int width, int height) {
var destinationRect = new Rectangle(0, 0, width, height);
var destinationImage = new Bitmap(width, height);
destinationImage.SetResolution(image.HorizontalResolution, image.VerticalResolution);
using (var graphics = Graphics.FromImage(destinationImage)) {
graphics.CompositingMode = CompositingMode.SourceCopy;
graphics.CompositingQuality = CompositingQuality.HighQuality;
using (var wrapMode = new ImageAttributes()) {
wrapMode.SetWrapMode(WrapMode.TileFlipXY);
graphics.DrawImage(image, destinationRect, 0, 0, image.Width, image.Height,
GraphicsUnit.Pixel, wrapMode);
}
}
return (Image)destinationImage;
}
static void Main(string[] args) {
string path = "C:\\Images\\img1.jpg";
Image img = Image.FromFile(path);
Bitmap imgbitmap = new Bitmap(img);
Image resizedImage = resizeImage(imgbitmap, new Size(200, 200));
}
}
}
Let’s break down how it works.
Step 1: Load the Original Image
First, you need to load the original image using Image.FromFile
. Make sure to replace "C:\\Images\\img1.jpg"
with the path to your own image file.
string path = "C:\\Images\\img1.jpg";
Image img = Image.FromFile(path);
Step 2: Create a Destination Bitmap
Create a destination Bitmap
object that will hold the resized image. Set its resolution to match the original image using SetResolution
.
The destinationImage.SetResolution()
function maintains the dpi of the image regardless of its actual size.
var destinationRect = new Rectangle(0, 0, width, height);
var destinationImage = new Bitmap(width, height);
destinationImage.SetResolution(image.HorizontalResolution, image.VerticalResolution);
Step 3: Resize the Image
Now, we use Graphics.FromImage
to obtain a Graphics
object associated with the destination Bitmap
. We set the compositing mode and quality for the best results.
using (var graphics = Graphics.FromImage(destinationImage)) {
graphics.CompositingMode = CompositingMode.SourceCopy;
graphics.CompositingQuality = CompositingQuality.HighQuality;
using (var wrapMode = new ImageAttributes()) {
wrapMode.SetWrapMode(WrapMode.TileFlipXY);
graphics.DrawImage(image, destinationRect, 0, 0, image.Width, image.Height, GraphicsUnit.Pixel,
wrapMode);
}
}
The graphics.CompositingMode = CompositingMode.SourceCopy
property specifies that when a color is rendered it overwrites the background color.
The graphics.CompositingQuality = CompositingQuality.HighQuality
property specifies that we only want high quality image to be rendered.
The wrapMode.SetWrapMode(WrapMode.TileFlipXY)
function prevents ghosting around the image borders.
Step 4: Save the Resized Image
Finally, you can save the resized image to a file using the Save
method. In this example, the resized image is saved as "resized.jpg"
. You can choose the format of the saved image by changing the file extension (e.g., .jpg
, .png
, .bmp
).
resizedImage.Save("resized.jpg");
Conclusion
Resizing images is a common task in software development, and C# offers multiple approaches to achieve this. In this article, we focused on resizing images using the Bitmap
class. We walked through the entire process, from loading the original image to saving the resized version.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn