How to Implement Multiline Label in C#
-
Create a Multiline Label With the
Label.AutoSize
Property inC#
-
Create a Multiline Label With the
Panel
Method inC#
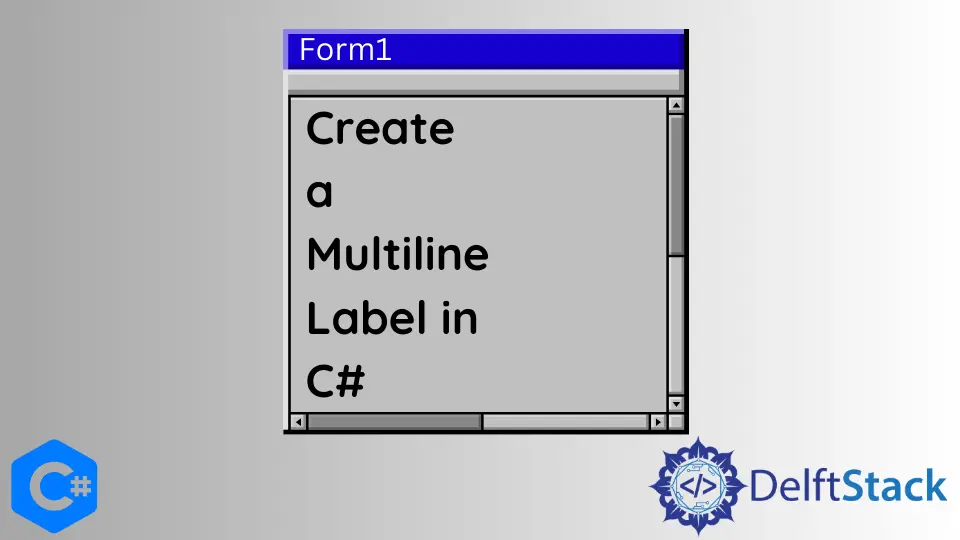
This tutorial will introduce the methods to create a multiline label in C#.
Create a Multiline Label With the Label.AutoSize
Property in C#
The Label.AutoSize
property specifies whether the label can automatically adjust its size to fit the text being displayed in C#. The Label.AutoSize
property has a boolean value and must be set to true
if we want our label to automatically resize itself to fit the text being displayed and false
if we want do not want our label to automatically resize itself to fit the text being displayed. We can then set the label’s maximum size with the Control.MaximumSize
property in C#. The following code example shows us how to create a multiline label with the Label.AutoSize
property in C#.
using System;
using System.Drawing;
using System.Windows.Forms;
namespace multi_line_label {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e) {
string data = "This is some data that we want to display";
label1.Text = data;
label1.AutoSize = true;
label1.MaximumSize = new Size(50, 0);
}
}
}
Output:
In the above code, we created a multiline label with the Label.AutoSize
and Control.MaximumSize
properties in C#.
Create a Multiline Label With the Panel
Method in C#
We can also use a Panel
control to create a multiline label in C#. We can place the desired label inside a panel and then handle the ClientSizeChanged
event for the panel. The ClientSizeChanged
event is invoked whenever the size of a control inside the panel changes. We can resize the label with the Label.MaximumSize
property in C#. The following code example shows us how to create a multiline label with the Panel
method in C#.
using System;
using System.Drawing;
using System.Windows.Forms;
namespace multi_line_label {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e) {
string data = "This is some data that we want to display";
label1.Text = data;
label1.AutoSize = true;
}
private void panel1_ClientSizeChanged(object senderObject, EventArgs eventArguments) {
label1.MaximumSize =
new Size((senderObject as Control).ClientSize.Width - label1.Left, 10000);
}
}
}
Output:
We created a multiline label in the above code by placing the label inside a panel and handling the ClientSizeChanged
event inside the panel in C#. We first specified the Label.AutoSize
property to true
and specified the label’s maximum size inside the ClientSizeChanged
event in the panel.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn