How to Add Element to Vector of Pairs in C++
-
Use
push_back
andmake_pair
to Add Element to Vector of Pairs -
Use
push_back
and Cast to Pair to Add Element to Vector of Pairs -
Use
emplace_back
to Add Element to Vector of Pairs
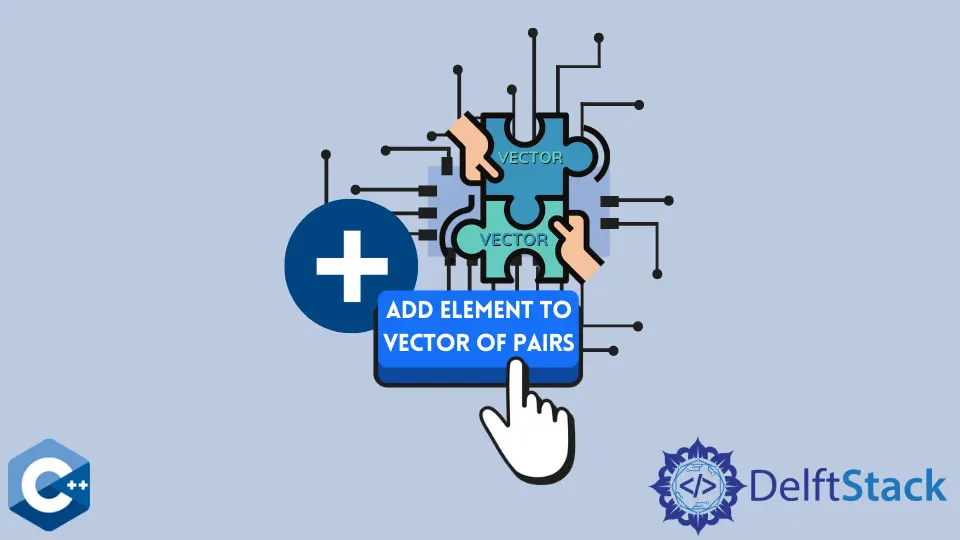
This article will explain several methods of adding an element to a vector of pairs in C++.
Use push_back
and make_pair
to Add Element to Vector of Pairs
The vector
container can hold std::pair
type elements, which is the class template for holding the two heterogeneous object types as one data unit. It’s similar to the more generally known tuple
data type from different programming languages like Python, except that it can only hold 2 elements.
A vector of pairs is declared with the expression - vector<pair<int, string>>
and it can be initialized the same way as the structure. Once we need to push additional std::pair
type elements to the vector
, the push_back
method can be utilized. Notice, though, it needs an element to be constructed using the make_pair
function.
In the following example, we use <int, string>
pairs and the syntax to add an element to the vector of pairs is push_back(make_pair(55, "fifty-five"))
.
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::make_pair;
using std::pair;
using std::string;
using std::vector;
template <typename T>
void printVectorElements(vector<T> &vec) {
for (auto i = 0; i < vec.size(); ++i) {
cout << "(" << vec.at(i).first << "," << vec.at(i).second << ")"
<< "; ";
}
cout << endl;
}
int main() {
vector<pair<int, string>> vec1 = {
{12, "twelve"}, {32, "thirty-two"}, {43, "forty-three"}};
cout << "vec1: ";
printVectorElements(vec1);
vec1.push_back(make_pair(55, "fifty-five"));
cout << "vec1: ";
printVectorElements(vec1);
cout << endl;
return EXIT_SUCCESS;
}
Output:
vec1: (12,twelve); (32,thirty-two); (43,forty-three);
vec1: (12,twelve); (32,thirty-two); (43,forty-three); (55,fifty-five);
Use push_back
and Cast to Pair to Add Element to Vector of Pairs
As an alternative to the previous method, we can cast the literal values to a pair and then insert the expression into the push_back
method. Although, this method is less clear for readability and arguably error-prone for larger codebases.
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::make_pair;
using std::pair;
using std::string;
using std::vector;
template <typename T>
void printVectorElements(vector<T> &vec) {
for (auto i = 0; i < vec.size(); ++i) {
cout << "(" << vec.at(i).first << "," << vec.at(i).second << ")"
<< "; ";
}
cout << endl;
}
int main() {
vector<pair<int, string>> vec1 = {
{12, "twelve"}, {32, "thirty-two"}, {43, "forty-three"}};
cout << "vec1: ";
printVectorElements(vec1);
vec1.push_back(pair(55, "fifty-five"));
cout << "vec1: ";
printVectorElements(vec1);
cout << endl;
return EXIT_SUCCESS;
}
Output:
vec1: (12,twelve); (32,thirty-two); (43,forty-three);
vec1: (12,twelve); (32,thirty-two); (43,forty-three); (55,fifty-five);
Use emplace_back
to Add Element to Vector of Pairs
The emplace_back
method is a built-in function of the vector
container that constructs a new element at the end of the object. Notice that, for the emplace_back
to work, an element type should have a constructor for args
. Since we are using the function to construct the std::pair
elements, it’s safe to call it with literal values as shown in the following code sample.
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::make_pair;
using std::pair;
using std::string;
using std::vector;
template <typename T>
void printVectorElements(vector<T> &vec) {
for (auto i = 0; i < vec.size(); ++i) {
cout << "(" << vec.at(i).first << "," << vec.at(i).second << ")"
<< "; ";
}
cout << endl;
}
int main() {
vector<pair<int, string>> vec1 = {
{12, "twelve"}, {32, "thirty-two"}, {43, "forty-three"}};
cout << "vec1: ";
printVectorElements(vec1);
vec1.emplace_back(55, "fifty-five");
cout << "vec1: ";
printVectorElements(vec1);
cout << endl;
return EXIT_SUCCESS;
}
Output:
vec1: (12,twelve); (32,thirty-two); (43,forty-three);
vec1: (12,twelve); (32,thirty-two); (43,forty-three); (55,fifty-five);
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook