How to Set the Precision of Floating-Point Numbers in C++
-
Use
std::setprecision
to Set the Precision of Floating-Point Numbers in C++ -
Use
std::floor
andstd::ceil
to Modify the Precision of Floating-Point Numbers -
Use
std::round
andstd::lround
to Modify the Precision of Floating-Point Numbers
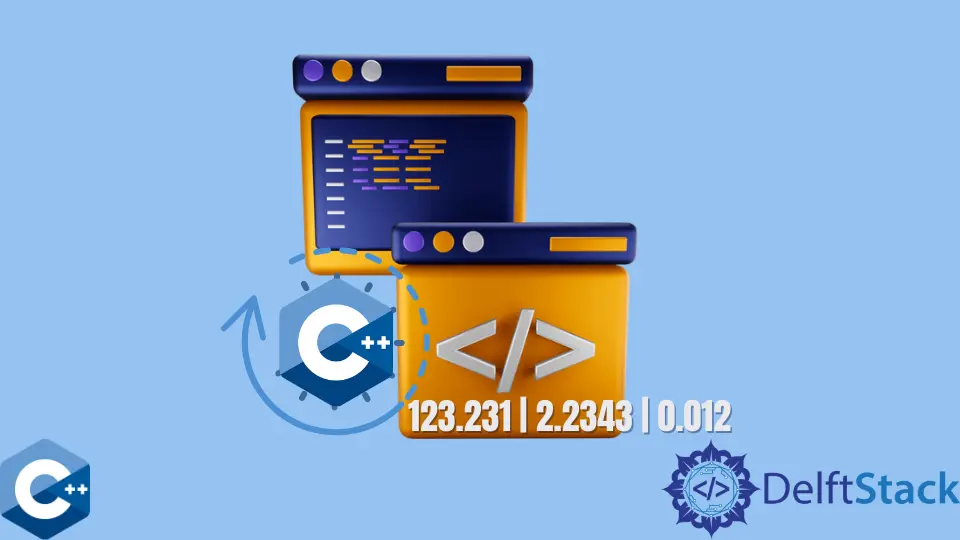
This article will explain several methods of how to set the precision of floating-point numbers in C++.
Use std::setprecision
to Set the Precision of Floating-Point Numbers in C++
std::setprecision
is part of the STL I/O manipulators library which can be used to format the input/output streams. setprecision
changes the precision of the floating-point numbers, and it only takes an integral parameter specifying the number digits to display after the decimal point. Namely, the default precision assumed implicitly for the floating-point numbers is six digits after the comma. Still, sometimes the float may be displayed by the scientific notation when the number is too small, and no manipulators are used. Note that such numbers may lose all significant digits and appear as zeros, as demonstrated in the following example code.
#include <iomanip>
#include <iostream>
#include <vector>
using std::cout;
using std::endl;
using std::fixed;
using std::setprecision;
using std::vector;
int main() {
vector<double> d_vec = {123.231, 2.2343, 0.012,
26.9491092019, 113, 0.000000234};
for (auto &i : d_vec) {
cout << i << " | ";
}
cout << endl;
for (auto &i : d_vec) {
cout << setprecision(3) << i << " | ";
}
cout << endl;
return EXIT_SUCCESS;
}
Output:
123.231 | 2.2343 | 0.012 | 26.9491 | 113 | 2.34e-07 |
123.231 | 2.234 | 0.012 | 26.949 | 113.000 | 0.000 |
Use std::floor
and std::ceil
to Modify the Precision of Floating-Point Numbers
std::floor
and std::ceil
functions are provided by the <cmath>
header, which was originally implemented in C standard library. ceil
function computes the smallest integer value greater than or equal to the floating-point passed as the only argument. On the other hand, floor
computes the largest integer value less than or equal to the argument. These functions are defined for float
, double
, and long double
types.
#include <cmath>
#include <iomanip>
#include <iostream>
#include <vector>
using std::cout;
using std::endl;
using std::fixed;
using std::vector;
int main() {
vector<double> d_vec = {123.231, 2.2343, 0.012,
26.9491092019, 113, 0.000000234};
for (auto &i : d_vec) {
cout << i << " | ";
}
cout << endl;
for (auto &i : d_vec) {
cout << fixed << std::ceil(i) << " | ";
}
cout << endl;
for (auto &i : d_vec) {
cout << fixed << std::floor(i) << " | ";
}
cout << endl;
return EXIT_SUCCESS;
}
Output:
123.231 | 2.2343 | 0.012 | 26.9491 | 113 | 2.34e-07 |
124.000000 | 3.000000 | 1.000000 | 27.000000 | 113.000000 | 1.000000 |
123.000000 | 2.000000 | 0.000000 | 26.000000 | 113.000000 | 0.000000 |
Use std::round
and std::lround
to Modify the Precision of Floating-Point Numbers
Alternatively, std::round
and std::round
can be utilized to calculate the nearest integer values that are rounded away from zero. These functions are likely to throw floating-point arithmetic-related errors, which are discussed in detail on the page.
#include <cmath>
#include <iomanip>
#include <iostream>
#include <vector>
using std::cout;
using std::endl;
using std::fixed;
using std::vector;
int main() {
vector<double> d_vec = {123.231, 2.2343, 0.012,
26.9491092019, 113, 0.000000234};
for (auto &i : d_vec) {
cout << i << " | ";
}
cout << endl;
for (auto &i : d_vec) {
cout << fixed << std::round(i) << " | ";
}
cout << endl;
for (auto &i : d_vec) {
cout << fixed << std::lround(i) << " | ";
}
cout << endl;
return EXIT_SUCCESS;
}
Output:
123.231 | 2.2343 | 0.012 | 26.9491 | 113 | 2.34e-07 |
123.000000 | 2.000000 | 0.000000 | 27.000000 | 113.000000 | 0.000000 |
123 | 2 | 0 | 27 | 113 | 0 |
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook