How to Change Console Color in C++
- Use ANSI Escape Codes to Change Console Color
-
Use
SetConsoleTextAttribute()
Method to Change Console Color in C++
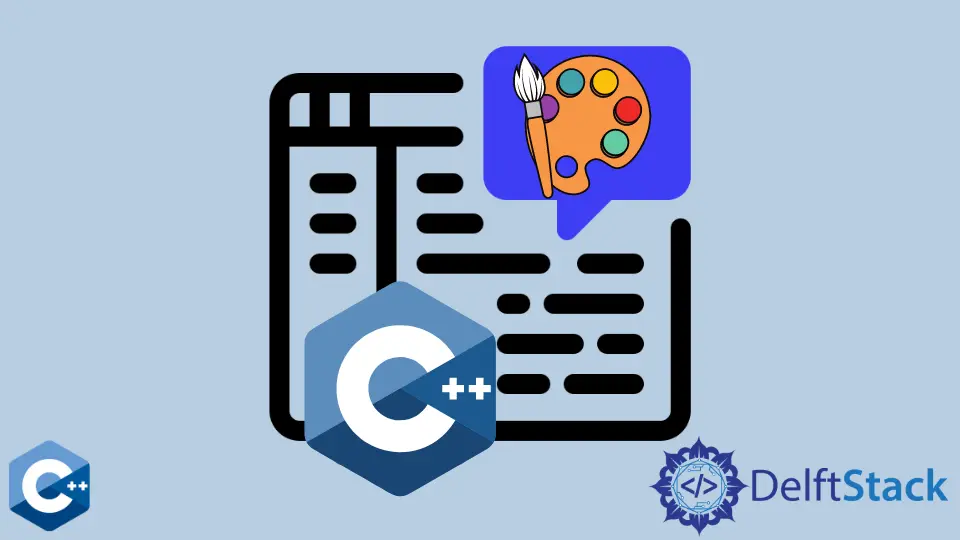
This article will explain several methods of how to change the console color in C++.
Use ANSI Escape Codes to Change Console Color
Since the colors are not part of the standard C++ library and the console-specific features are mostly handled by the operating systems, there’s no native language feature to add colors to output streams. Although, we discuss some platform-specific ways to handle colorizing text output.
ANSI escape codes are a relatively portable way to solve this problem. Escape codes are byte sequences starting mostly with an ASCII Escape character and bracket character followed by parameters. These characters are embedded into the output text, and the console interprets the sequences as commands rather than text to display. ANSI codes include multiple color formats, full details of which can be seen on this page. In the following code example, we demonstrate defining several color characters as macros and later include these symbols in the printf
string arguments. Notice that printf
concatenates multiple double-quoted strings passed in the first parameter place.
#include <iostream>
#define NC "\e[0m"
#define RED "\e[0;31m"
#define GRN "\e[0;32m"
#define CYN "\e[0;36m"
#define REDB "\e[41m"
using std::cout;
using std::endl;
int main(int argc, char *argv[]) {
if (argc < 2) {
printf(RED "ERROR" NC
": provide argument as follows -> ./program argument\n");
exit(EXIT_FAILURE);
}
printf(GRN "SUCCESS!\n");
return EXIT_SUCCESS;
}
Output (without program argument):
ERROR: provide argument as follows -> ./program argument
Output (with program argument):
SUCCESS!
Alternatively, the same escape codes can be used with a cout
call. Note that it’s not necessary to use the <<
stream insert operator multiple times and just combine macro symbols in conjunction with the string literals as they will automatically get combined.
#include <iostream>
#define NC "\e[0m"
#define RED "\e[0;31m"
#define GRN "\e[0;32m"
#define CYN "\e[0;36m"
#define REDB "\e[41m"
using std::cout;
using std::endl;
int main() {
cout << CYN "Some cyan colored text" << endl;
cout << REDB "Add red background" NC << endl;
cout << "reset to default colors with NC" << endl;
return EXIT_SUCCESS;
}
Output:
Some cyan colored text
Add red background
reset to default with NC
Use SetConsoleTextAttribute()
Method to Change Console Color in C++
SetConsoleTextAttribute
is the Windows API method to set output text colors using different parameters. This function sets the attributes of characters written to the console screen buffer by the WriteFile
or WriteConsole
functions. The full description of the character attributes can be seen on this page.
#include << windows.h>>
#include <iostream>
#include <string>
using std::cout;
using std::endl;
int main() {
std::string str("HeLLO tHERe\n");
DWORD bytesWritten = 0;
HANDLE cout_handle = GetStdHandle(STD_OUTPUT_HANDLE);
SetConsoleTextAttribute(console_handle,
FOREGROUND_RED | BACKGROUND_INTENSITY);
WriteFile(cout_handle, str.c_str(), str.size(), &bytesWritten, NULL);
return EXIT_SUCCESS;
}
Output:
Some red colored text
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook